hi there,
i am new here and new to coding.
I am trying to set a button that can automatically set a SL/TP based on points or ATR multiplier.
So far I have managed to get the SL based on points using the properties as a way to change it. I have a code at the end to capture the ATR, however I have not figured it out how to use that to actually be usable on the SL function.
So far i have also not figured it out how to input the TP (either via points or ATR value)
any help or direction is mostly welcome
thanks in advance
The return value of iATR is in ticks.
Once you have the value, you can add or subtract it from a quote (price). Because you'll be performing arithmetic to arrive at the stoploss, you'll need to normalize the result before modifying the order with the result. Read this for more info on how to go about that.
Also, do not use _Point. Use tick size, instead. You can get that value by using
SymbolInfoDouble(_Symbol, SYMBOL_TRADE_TICK_SIZE);
The reason you don't want to use point is because it only tells you the decimal place, not the smallest change in price. It works in some markets, like Forex, because the smallest change in price is 0.00001 or 0.0001 but, in other markets, the tick size can be 0.25 while the point would be 0.01.
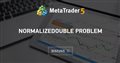
- 2022.06.18
- www.mql5.com
Incorrect! The values provided by iATR is price quote range, or a price change or price delta, or which ever name you use. It is NOT in ticks.
For all intents and purposes, your response provides nothing of value.
But I am curious: why not just help the guy out yourself? His question was sitting there for a week. You only chose to "contribute" when I offered to help. Considering our last exchange, this strikes me as rather peculiar.
NB! I will refer to the OP as "they" as I don't know their gender ...
Because their query is too generalised and requests answers for multiple issues, and the code seems not to be theirs.
- They request help for how to code a button.
- They request help for using the ATR to set a value for S/L and T/P
- They request help for setting the Take-Profit
The code itself does not compile and shows a mixture of code made by different people, which they seem to have repurposed but don't understand.
If they were able to code the stopless, then it stands to reason that they would with the same logic be able to code the take-profit, yet they state that they don't know how.
They claim to have created the function to calculate the stopless size from the iATR, but then don't know how to apply it.
In all, I get the sense of someone using snippets from other peoples code but not having the basic knowledge of coding to put it together.
My conclusion is that, what ever guidance we give, will not be sufficiently understood and that the "help" they want, is that someone just give them the final code with it all corrected for them.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi there,
i am new here and new to coding.
I am trying to set a button that can automatically set a SL/TP based on points or ATR multiplier.
So far I have managed to get the SL based on points using the properties as a way to change it. I have a code at the end to capture the ATR, however I have not figured it out how to use that to actually be usable on the SL function.
So far i have also not figured it out how to input the TP (either via points or ATR value)
any help or direction is mostly welcome
thanks in advance