What to use instead of booleans.
Where are you using the boolean?
What is problem you are facing?
Answer depends on that
Do you really expect an answer with such a vague question? There are no mind readers here and our crystal balls are cracked. Always post all relevant code (using Code button) or attach the source file.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problem
What to use instead of booleans.
Use enum, for example:
enum ENUM_BE_TYPE { aboveBE, // Standard aboveBE_Small, // Small aboveBE_Large // Large }; input ENUM_BE_TYPE InpBeType = aboveBE; // Breakeven Type
If you are not a programmer and have no understanding of the MQL language, then how are we going to be able to help?
If we give you code samples, as Vinicius has given, you won't understand it and don't know how to implement it.
If we explain it in English, you will then not be able to implement it either because you don't know how to code it.
So, please explain to us how it is that you want to NOT use boolean, if you don't understand what a "boolean" or a "enumeration" is or how to use it.
It might be best for you to hire someone to code it for you instead, given your requirements.
Hi Vinicius de Oliveira, and what would it look like, I'm not a programmer, I have no idea.
Hello Christer!
If you don't have the necessary programming knowledge to carry out the intended implementations in your code, you can still try the following options:
- Ask the code author to make the changes;
- Make a complete description, in detail, of what you want and create an order in the Freelance service;
- Dedicate time to study and learn the MQL5 programming language.
EDIT: As Fernando described above, it is difficult (if not impossible) for us to help you if you don't have some knowledge of the MQL5 language to understand what we are trying to explain to you.
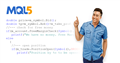
- www.mql5.com
Hello Christer!
If you don't have the necessary programming knowledge to carry out the intended implementations in your code, you can still try the following options:
- Ask the code author to make the changes;
- Make a complete description, in detail, of what you want and create an order in the Freelance service;
- Dedicate time to study and learn the MQL5 programming language.
EDIT: As Fernando described above, it is difficult (if not impossible) for us to help you if you don't have some knowledge of the MQL5 language to understand what we are trying to explain to you.
Hello everyone who is trying to help me, thank you all very much, I was a programmer from 2000 until 2017 when I had my stroke, so all the things I learned until 2017 are like blown away, but I am trying to learn again.
The reason I want to remove the bool is that it prevents the others from doing their job, as the first one goes in and does their job but it is always true, so those who come after cannot do their job, that is what I calculated, I am wrong, you can correct me.
That's how I read the code, because it's only 10 - 15 lines of code, and I have to admit one thing I run with Expert Advisor Bilder, I have no one to turn to, but what I do myself and I'm no one wealthy person, I am 67 years old and I have my pension after my stroke. Thanks anyway.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
What to use instead of booleans.
He doesn't do as I thought, because the first one is true, the others can't do their job.
void TrailingStopBE_Small(ENUM_ORDER_TYPE type, double profit_Small, bool aboveBE_Small, double aboveBEval_Small) //set Stop Loss to open price if in profit
{
profit_Small = NormalizeDouble(profit_Small, Digits());
int total = PositionsTotal();
for(int i = total-1; i >= 0; i--)
{
if(PositionGetTicket(i) <= 0) continue;
if(PositionGetInteger(POSITION_MAGIC) != MagicNumber || PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_TYPE) != type) continue;
MqlTick last_tick;
SymbolInfoTick(Symbol(), last_tick);
double SL = PositionGetDouble(POSITION_SL);
double openprice = PositionGetDouble(POSITION_PRICE_OPEN);
ulong ticket = PositionGetInteger(POSITION_TICKET);
if(type == ORDER_TYPE_BUY && last_tick.bid > openprice + profit_Small && (NormalizeDouble(SL, Digits()) <= 0 || openprice > SL))
myOrderModify(ORDER_TYPE_BUY, ticket, openprice + aboveBEval_Small, 0);
else if(type == ORDER_TYPE_SELL && last_tick.ask < openprice - profit_Small && (NormalizeDouble(SL, Digits()) <= 0 || openprice < SL))
myOrderModify(ORDER_TYPE_SELL, ticket, openprice + aboveBEval_Small, 0);
}
}
// ----------------------------------------------------------------------------------------------------------------
void TrailingStopBE(ENUM_ORDER_TYPE type, double profit, bool aboveBE, double aboveBEval) //set Stop Loss to open price if in profit
{
profit = NormalizeDouble(profit, Digits());
int total = PositionsTotal();
for(int i = total-1; i >= 0; i--)
{
if(PositionGetTicket(i) <= 0) continue;
if(PositionGetInteger(POSITION_MAGIC) != MagicNumber || PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_TYPE) != type) continue;
MqlTick last_tick;
SymbolInfoTick(Symbol(), last_tick);
double SL = PositionGetDouble(POSITION_SL);
double openprice = PositionGetDouble(POSITION_PRICE_OPEN);
ulong ticket = PositionGetInteger(POSITION_TICKET);
if(type == ORDER_TYPE_BUY && last_tick.bid > openprice + profit && (NormalizeDouble(SL, Digits()) <= 0 || openprice > SL))
myOrderModify(ORDER_TYPE_BUY, ticket, openprice + aboveBEval, 0);
else if(type == ORDER_TYPE_SELL && last_tick.ask < openprice - profit && (NormalizeDouble(SL, Digits()) <= 0 || openprice < SL))
myOrderModify(ORDER_TYPE_SELL, ticket, openprice + aboveBEval, 0);
}
}
// --------------------------------------------------------------------------------------------------------------------
void TrailingStopBE_Large(ENUM_ORDER_TYPE type, double profit_Large, bool aboveBE_Large, double aboveBEval_Large) //set Stop Loss to open price if in profit
{
profit_Large = NormalizeDouble(profit_Large, Digits());
int total = PositionsTotal();
for(int i = total-1; i >= 0; i--)
{
if(PositionGetTicket(i) <= 0) continue;
if(PositionGetInteger(POSITION_MAGIC) != MagicNumber || PositionGetString(POSITION_SYMBOL) != Symbol() || PositionGetInteger(POSITION_TYPE) != type) continue;
MqlTick last_tick;
SymbolInfoTick(Symbol(), last_tick);
double SL = PositionGetDouble(POSITION_SL);
double openprice = PositionGetDouble(POSITION_PRICE_OPEN);
ulong ticket = PositionGetInteger(POSITION_TICKET);
if(type == ORDER_TYPE_BUY && last_tick.bid > openprice + profit_Large && (NormalizeDouble(SL, Digits()) <= 0 || openprice > SL))
myOrderModify(ORDER_TYPE_BUY, ticket, openprice + aboveBEval_Large, 0);
else if(type == ORDER_TYPE_SELL && last_tick.ask < openprice - profit_Large && (NormalizeDouble(SL, Digits()) <= 0 || openprice < SL))
myOrderModify(ORDER_TYPE_SELL, ticket, openprice + aboveBEval_Large, 0);
}
}