Any questions from newcomers on MQL4 and MQL5, help and discussion on algorithms and codes - page 1493

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Please advise mql5, I want to collect statistics of the average size of eg a 12 hour candle, today for example is Friday, and I want to take the data from Thursday, Wednesday, Tuesday and Monday for the calculations.
I think we cannot do without the loop. But the loop can be built in different ways. Pay attention to CopyRates()
We set PERIOD_H1, start_time - date 12:00 and count 1. At the next iteration, we add to this date or subtract from it (depending on which way the loop is organized) PeriodSeconds(PERIOD_D1)
Please tell me how to enumerate indicator parameters in iCustom.
I have a stochastic indicator with allergy, it gives a signal on crossing of moving lines. Please tell me how to make it give a signal by crossing the slips only when it is above or below the overbought or oversold zone.
Good day to all.
Can you please tell me how to code the following condition correctly using I don't remember which mathematical function.
if (Bid - Low[1]>=0.0030 && Bid - Low[1]<0.0035) {action;}
I know that there is a math function that can be used in the above condition without && sign . But I don't remember what this mathematical function is called and how to apply it.
Thanks for the help.
If you change a global variable in a function, it will change. But this is a dangerous way of programming, because in the code, as it grows, there will be unobvious assignments in different functions of the program.
There's a main function in the program, that's where you do the global variable assignment. And in other functions, do it this way:
Or like this:
how to correctly code the following condition using I don't remember which mathematical function
I don't know a better way of setting the condition
Good day to all.
Can you please tell me how to code the following condition correctly using I don't remember which mathematical function.
if (Bid - Low[1]>=0.0030 && Bid - Low[1]<0.0035) {action;}
I know that there is a math function that can be used in the above condition without && sign and the program will check for price consistency in the range of 4 points. But I don't remember what this mathematical function is called and how to apply it.
Thanks for the help.
Without and
Thank you very much for the tip.
Here is the code
The program does not read Pr as 1.60854 althoughPrint( ) shows the value of Pr as 1.60854 . As a result, the program opens the second order, but it shouldn't do that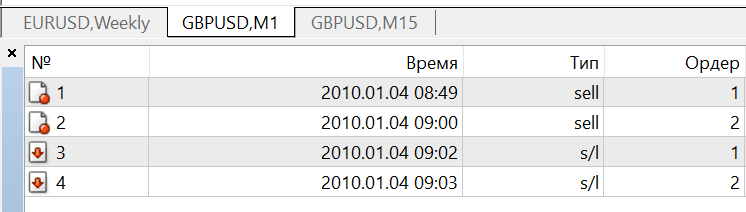
.
And if we set the number 1.60854 instead of the Pr variable , the program reads it and doesn't open the second order.
QUESTION What changes we should make in the code so that the program reads Pr and does not open the second order.
Thank you for your help.