All indicators are supplied with the terminal and indicators are supplied in open source code. MACD lies here: [data folder] \ MQL5 \ Indicators \ Examples \ MACD.mq5
Thank you for your advice.
It was useless.
I used the one you suggested and got exactly the same result, meaning nothing.
Exactly the same result as described by me, above.
The indicator in the Examples is exactly the same one I used.
It only works in the MODE_EMA for the MA handles.
In any other of the available MA_METHOD's it does not work.
Thank you for your advice.
It was useless.
I used the one you suggested and got exactly the same result, meaning nothing.
Exactly the same result as described by me, above.
The indicator in the Examples is exactly the same one I used.
It only works in the MODE_EMA for the MA handles.
In any other of the available MA_METHOD's it does not work.
Indicator idea
Now in the MACD indicator for Moving Average 'Fast' and 'Slow' you can configure the averaging method and price type.
Rice. 1. MACD Custom Averaging
Rice. 2. MACD Custom Averaging - parameters
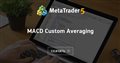
- www.mql5.com
MACD Custom Averaging
Indicator idea
Now in the MACD indicator for Moving Average 'Fast' and 'Slow' you can configure the averaging method and price type.
Rice. 1. MACD Custom Averaging
Rice. 2. MACD Custom Averaging - parameters
Now thanking you very much!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
There is this 3-10 Oscillator for MT4 which works like this:
It uses the MODE_SMA for Fast & Slow MA's instead of the MODE_EMA in the standard MACD indicator.
Theoretically, it should be simple to modify the MACD.mq5 in the same mode.
I used the following code:
Thus, I do this:
And the indicator stops working!
I get a blank picture, as in the atttached image, with "inf" & "-inf".
I wasn't able to get around this problem.
Asking for help!