I've been looking for it but can't find it.
I.e., the function that returns the "int pos, // number in the list of objects" referred to in the ObjectName function documentation page.
For example:
ObjectName accepts:
int pos, // number in the list of objects
So there is a list of objects somewhere, each with a unique "pos" in the list. How do we access it?
Object names are used as unique IDs, (the only IDs we can use with object Get and Set functions,) so where is the function that accepts names as unique IDs and returns their unique "pos" in the "list of objects" ?
I can't find one. I'd hoped that MQL5 would make up for some of the glaring deficits of 4, but it seems there are some basic ones that were not addressed.
It sucks when we have to hand-code routines that should have been (logically, reasonably) provided as stock, native functions in a coding language.
Use ObjectsTotal() to get the total objects on a chart, window and possibly type.
Amir, thanks -- and yes, I know how I'd code it, (I'm in the process right now.) ObjectsTotal is a good place to start, so...
I'll take your response as an implicit "There ain't no such function".
Pretty inexplicable from a conscientious coder perspective.
Use ObjectsTotal() to get the total objects on a chart, window and possibly type.
Actually, what I'm going to do is code an overload for ObjectCreate that maintains two arrays, one of object name and the other of object list index. Then I can forget about MQL5's deficit and each time an object is created anywhere on a chart I'll have easy, quick access to the info that rightly should have come stock with the MQL language.
Actually, what I'm going to do is code an overload for ObjectCreate that maintains two arrays, one of object name and the other of object list index. Then I can forget about MQL5's deficit and each time an object is created anywhere on a chart I'll have easy, quick access to the info that rightly should have come stock with the MQL language.
Actually, what I'm going to do is code an overload for ObjectCreate that maintains two arrays, one of object name and the other of object list index. Then I can forget about MQL5's deficit and each time an object is created anywhere on a chart I'll have easy, quick access to the info that rightly should have come stock with the MQL language.
Now that I've done it, I only needed an Obj_Name[] array. I already had a function that will return the array index of a given string in the array. I've got so many workaround functions now that could have been included stock in MQL5 I forget I coded them, LMAO! I'll have to create my own documentation reference, I guess.
Here's the code in case anyone is interested:
//--- Global Object array string sObj_Name[]; int iObj_CNT = 0; string Obj_Name(int piObxIX,long plChtID=0,int pSubWndw=0,ENUM_OBJECT peObjType=-1) { return(sObj_Name[piObxIX]); } int Obj_Index(string psObjNme,long plChtID=0,int pSubWndw=0,ENUM_OBJECT peObjType=-1) { return(GetIXFor(psObjNme,sObj_Name)); } int ObjectCreate(string psObjNme,ENUM_OBJECT peObjType,long plChtID=0,int pSubWndw=0,datetime pdtDTM1=0,double dPRC1=0.0,datetime pdtDTM2=0,double dPRC2=0.0) { int iGO_ID = E_V; iObj_CNT = ObjectsTotal(plChtID); bool bRslt = ObjectCreate(plChtID,psObjNme,peObjType,pSubWndw,pdtDTM1,dPRC1,pdtDTM2,dPRC2); if(bRslt==true) { iObj_CNT = ArrayResize(sObj_Name,iObj_CNT); iGO_ID = iObj_CNT - 1; } return(iGO_ID); } //+------------------------------------------------------------------+ //| Get the IX of the first or last cell with given value //+------------------------------------------------------------------+ template<typename T> // alias for WhereInArrayIs() int GetIXFor(T ptFindVLU,T& pAry[],int piSttRow=0,bool pbGetLast=false) { return(WhereInArrayIs(ptFindVLU,pAry,piSttRow,pbGetLast)); } // template<typename T> // alias for WhereInArrayIs() int GetIXFor(T ptFindVLU,T& pAry[][],int piSttRow=0,int piClm=0,bool pbGetLast=false) { return(WhereInArrayIs(ptFindVLU,pAry,piSttRow,piClm,pbGetLast)); } // template<typename T> // alias for WhereInArrayIs() int GetIXFor(T ptFindVLU,T& pAry[][][],int piSttRow=0,int piClm=0,int piCub=0,bool pbGetLast=false) { return(WhereInArrayIs(ptFindVLU,pAry,piSttRow,piClm,piCub,pbGetLast)); } // template<typename T> int WhereInArrayIs(T ptFindVLU,T& pAry[],int piSttRow=0,bool pbGetLast=false) { int iRowSZ = ArrayRange(pAry,0); if(iRowSZ<=0) return(E_V); int iFndRX = E_V; int iDir = 1; // Default to forward from piSttRow int iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = 0; int iEndAt = iRowSZ-1; if(pbGetLast==true) { iDir = -1; // backwards from piSttRow iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = iRowSZ - 1; // Start at end iEndAt = 0; } int iMin = MathMIN(iSttAt,iEndAt); int iMax = MathMAX(iSttAt,iEndAt); for(int iRX=iSttAt;(iRX<=iMax && iRX>=iMin);iRX+=iDir) { if(pAry[iRX]==ptFindVLU) { iFndRX = iRX; break; } } return(iFndRX); } template<typename T> int WhereInArrayIs(T ptFindVLU,T& pAry[][],int piSttRow=0,int piClm=0,bool pbGetLast=false) { int iRowSZ = ArrayRange(pAry,0); if(iRowSZ<=0) return(E_V); int iFndRX = E_V; int iDir = 1; // Default to forward from piSttRow int iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = 0; int iEndAt = iRowSZ-1; if(pbGetLast==true) { iDir = -1; // backwards from piSttRow iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = iRowSZ - 1; // Start at end iEndAt = 0; } int iMin = MathMIN(iSttAt,iEndAt); int iMax = MathMAX(iSttAt,iEndAt); for(int iRX=iSttAt;(iRX<=iMax && iRX>=iMin);iRX+=iDir) { if(pAry[iRX,piClm]==ptFindVLU) { iFndRX = iRX; break; } } return(iFndRX); } template<typename T> int WhereInArrayIs(T ptFindVLU,T& pAry[][][],int piSttRow=0,int piClm=0,int piCub=0,bool pbGetLast=false) { int iRowSZ = ArrayRange(pAry,0); if(iRowSZ<=0) return(E_V); int iFndRX = E_V; int iDir = 1; // Default to forward from piSttRow int iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = 0; int iEndAt = iRowSZ-1; if(pbGetLast==true) { iDir = -1; // backwards from piSttRow iSttAt = piSttRow; if(piSttRow<0 || piSttRow>=iRowSZ) iSttAt = iRowSZ - 1; // Start at end iEndAt = 0; } int iMin = MathMIN(iSttAt,iEndAt); int iMax = MathMAX(iSttAt,iEndAt); for(int iRX=iSttAt;(iRX<=iMax && iRX>=iMin);iRX+=iDir) { if(pAry[iRX,piClm,piCub]==ptFindVLU) { iFndRX = iRX; break; } } return(iFndRX); }
There is no MQL5 deficit, if you load the ea on a chart that has objects, you do not know their names. In order to get the names you have to query them one by one using the index.
Well, since you seem to want to argue about it... I won't argue, I'll just list the reasons why I disagree.
Here's why I say it's a deficit:
- The list of the objects on any chart exists. If I add an EA to that chart and loop through the pre-existing list, incrementing an index from 0 to ObjectsTotal(0)-1, MQL5 already knows where to find them. There's no reason it couldn't provide that feature instead of omitting it and forcing me to code it, as I explained, returning the index for a given object name. ArrayMaximum and ArrayMinimum do something similar, "Return Value: The function returns an index of a found element taking into account the array serial. In case of failure it returns -1."
- The functionality is necessary. I proved this because I need it. You confirmed this because you suggested how to code it.
- MQL5 does not provide the needed functionality.
That's a bona fide deficit, as far as I'm concerned.
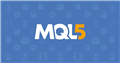
- www.mql5.com
Well, since you seem to want to argue about it... I won't argue, I'll just list the reasons why I disagree.
Here's why I say it's a deficit:
- The list of the objects on any chart exists. If I add an EA to that chart and loop through the pre-existing list, incrementing an index from 0 to ObjectsTotal(0)-1, MQL5 already knows where to find them. There's no reason it couldn't provide that feature instead of omitting it and forcing me to code it, as I explained, returning the index for a given object name. ArrayMaximum and ArrayMinimum do something similar, "Return Value: The function returns an index of a found element taking into account the array serial. In case of failure it returns -1."
- The functionality is necessary. I proved this because I need it. You confirmed this because you suggested how to code it.
- MQL5 does not provide the needed functionality.
That's a bona fide deficit, as far as I'm concerned.
Its interesting first to understand the need itself, because from what I see from going over the code it is trying to keep the indexes of its own created objects. Few queestions arise:
- Why do I care about the indexes? it is only used in order to get object names.
- What about objects created in between your program, by other programs or the user, on the same chart? First, your indexes kept in the program, will defer the indexes of the chart itself so why bother at all. Second, you will not get their names in your array. While you can intercept the object creation event while your program is running, but objects created prior to your program loading, you will not get their names unless you call the ObjectsTotal() and loop to get their names.
Also,I would also love to know what is the need for that at all.
- ObjectsTotal() and ObjectsName() is that list.
- Since everything about objects is by name, and you know the name of the objects you create, why do you need to know where the terminal stored it?
- If an object is deleted, by human or by other software, your index list is now bogus, and the name list contains garbage.
- If you maintain an array of all names, the index of a name is the index of the object. Index list is irrelevant (index[i] == i).
- ObjectsTotal() and ObjectsName() is that list.
- Since everything about objects is by name, and you know the name of the objects you create, why do you need to know where the terminal stored it?
- If an object is deleted, by human or by other software, your index list is now bogus, and the name list contains garbage.
- If you maintain an array of all names, the index of a name is the index of the object. Index list is irrelevant (index[i] == i).
Exactly.
Also can be added here, that the same Mql5 scheme is applied in many areas, such as orders (OrdersTotal() and getting order ticket), positions and so on.
The idea being the list is dynamic (can be added/deleted/updated) by any party, and the key (name,ticket etc) is accepted thru going over the internal list.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I've been looking for it but can't find it.
I.e., the function that returns the "int pos, // number in the list of objects" referred to in the ObjectName function documentation page.
For example:
ObjectName accepts:
int pos, // number in the list of objects
So there is a list of objects somewhere, each with a unique "pos" in the list. How do we access it?
Object names are used as unique IDs, (the only IDs we can use with object Get and Set functions,) so where is the function that accepts names as unique IDs and returns their unique "pos" in the "list of objects" ?
I can't find one. I'd hoped that MQL5 would make up for some of the glaring deficits of 4, but it seems there are some basic ones that were not addressed.
It sucks when we have to hand-code routines that should have been (logically, reasonably) provided as stock, native functions in a coding language.