- The value is not vague. It is not lagging. It is your code.
-
Do you really expect an answer? There are no mind readers here and our crystal balls are cracked. Always post all relevant code (using Code button) or attach the file.
How To Ask Questions The Smart Way. (2004
Be precise and informative about your problemWe can't see your broken code.
- The value is not vague. It is not lagging. It is your code.
-
Do you really expect an answer? There are no mind readers here and our crystal balls are cracked. Always post all relevant code (using Code button) or attach the file.
How To Ask Questions The Smart Way. (2004
Be precise and informative about your problemWe can't see your broken code.
//+------------------------------------------------------------------+ //| _5.mq5 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #include <Trade\PositionInfo.mqh> #include <Trade\Trade.mqh> CPositionInfo m_position; // trade position object CTrade m_trade; // trading object //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ //scalper without timecheck on exit ulong s_21magic=00121; input double TradeVolume=0.1; input int StopLoss=200; input int TakeProfit=1000; input int dummy_var=0; int rsiHandle1; double rsi1[]; input int rsiPeriod1=20; int rsiHandle2; double rsi2[]; input int rsiPeriod2=50; input double rsiEF=5.00; input double rsiEFdep=2.00; double rsiRF=rsiEF-rsiEFdep; double close[]; input int MAPeriod1=100; int ma1Handle; double ma1[]; input int MAPeriod2=200; int ma2Handle; double ma2[]; input bool TradeOnMonday = true; // Trade on Monday input bool TradeOnTuesday = true; // Trade on Tuesday input bool TradeOnWednesday = true; // Trade on Wednesday input bool TradeOnThursday = true; // Trade on Thursday input bool TradeOnFriday = true; // Trade on Friday input bool UsingTradingHour = true; // Using Trade Hour input int StartHour = 0; // Start Hour input int EndHour = 23; // End Hour bool openPosition=0; long positionType=0; ulong position_ticket=0; ulong pos_magic=0; input int twt=2; string ordercomment; int trp1=0; int trp3=0; //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int OnInit() { //--- rsiHandle1=iRSI(_Symbol,_Period,rsiPeriod1,PRICE_TYPICAL); rsiHandle2=iRSI(_Symbol,_Period,rsiPeriod2,PRICE_TYPICAL); ma1Handle=iMA(_Symbol,0,MAPeriod1,MODE_SMA,0,PRICE_CLOSE); ma2Handle=iMA(_Symbol,0,MAPeriod2,MODE_SMA,0,PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- ArraySetAsSeries(rsi1,true); ArraySetAsSeries(rsi2,true); ArraySetAsSeries(ma1,true); ArraySetAsSeries(ma2,true); ArraySetAsSeries(close,true); CopyBuffer(rsiHandle1,0,0,1,rsi1); CopyBuffer(rsiHandle2,0,0,1,rsi2); CopyBuffer(ma1Handle,0,0,1,ma1); CopyBuffer(ma2Handle,0,0,1,ma2); CopyClose(_Symbol,0,0,1,close); bool Time_Check = false; bool CheckDay = false; bool CheckHour = false; MqlDateTime TimeNow; TimeToStruct(TimeCurrent(),TimeNow); // Check day if((TimeNow.day_of_week == 1 && TradeOnMonday) || (TimeNow.day_of_week == 2 && TradeOnTuesday) || (TimeNow.day_of_week == 3 && TradeOnWednesday) || (TimeNow.day_of_week == 4 && TradeOnThursday) || (TimeNow.day_of_week == 5 && TradeOnFriday)) CheckDay = true; // Check hour if(StartHour < EndHour) if(TimeNow.hour >= StartHour && TimeNow.hour <= EndHour) CheckHour = true; if(StartHour > EndHour) if(TimeNow.hour >= StartHour || TimeNow.hour <= EndHour) CheckHour = true; if(StartHour == EndHour) if(TimeNow.hour == StartHour) CheckHour = true; // Check All if(UsingTradingHour && CheckDay && CheckHour) Time_Check = true; if(!UsingTradingHour && CheckDay) Time_Check = true; // Trade structures MqlTradeRequest request; MqlTradeResult result; ZeroMemory(request); // repeater preventers if(rsi1[0]-rsi2[0] < rsiRF && rsi1[0]-rsi2[0] > 0) trp1=2; if(rsi1[0]-rsi2[0] < 0 && rsi1[0]-rsi2[0] > -rsiRF) trp1=-2; if(rsi1[0]-rsi2[0] > rsiEF && trp1 == 2) trp1=1; if(rsi1[0]-rsi2[0] < -rsiEF && trp1 == -2) trp1=-1; trp3=0; if(ma2[0]<ma1[0] && ma1[0]<SymbolInfoDouble(_Symbol,SYMBOL_BID)) trp3=1; if(ma2[0]>ma1[0] && ma1[0]>SymbolInfoDouble(_Symbol,SYMBOL_ASK)) trp3=-1; // Current position information openPosition = PositionSelect(_Symbol); positionType = PositionGetInteger(POSITION_TYPE); position_ticket=PositionGetTicket(0); pos_magic=PositionGetInteger(POSITION_MAGIC); //buy if(trp1==1 || trp1==2) { if(positionType==POSITION_TYPE_SELL && pos_magic==s_21magic) m_trade.PositionClose(position_ticket); } if(trp1==1 && trp3==1 && Time_Check) { openPosition = PositionSelect(_Symbol); positionType = PositionGetInteger(POSITION_TYPE); position_ticket=PositionGetTicket(0); if(openPosition == NULL) { ZeroMemory(request); request.magic = s_21magic; request.action = TRADE_ACTION_DEAL; request.type = ORDER_TYPE_BUY; request.symbol = _Symbol; request.volume = TradeVolume; request.type_filling = ORDER_FILLING_IOC; request.price = SymbolInfoDouble(_Symbol,SYMBOL_ASK); request.sl =0; request.tp = 0; request.deviation = 50; request.type_time = ORDER_TIME_GTC; OrderSend(request,result); trp1=0; } // Modify SL/TP if(result.retcode == TRADE_RETCODE_PLACED || result.retcode == TRADE_RETCODE_DONE) { request.action = TRADE_ACTION_SLTP; do Sleep(100); while(PositionSelect(_Symbol) == false); double positionOpenPrice = PositionGetDouble(POSITION_PRICE_OPEN); if(StopLoss > 0) request.sl = positionOpenPrice - (StopLoss * _Point); if(request.sl > 0) OrderSend(request,result); } } //sell if(trp1==-1 || trp1==-2) { if(positionType==POSITION_TYPE_BUY && pos_magic==s_21magic) m_trade.PositionClose(position_ticket); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ if(trp1==-1 && trp3==-1 && Time_Check) { openPosition = PositionSelect(_Symbol); positionType = PositionGetInteger(POSITION_TYPE); position_ticket=PositionGetTicket(0); if(openPosition == NULL) { ZeroMemory(request); request.magic = s_21magic; request.action = TRADE_ACTION_DEAL; request.type = ORDER_TYPE_SELL; request.symbol = _Symbol; request.volume = TradeVolume; request.type_filling = ORDER_FILLING_IOC; request.price = SymbolInfoDouble(_Symbol,SYMBOL_ASK); request.sl =0; request.tp = 0; request.deviation = 50; request.type_time = ORDER_TIME_GTC; OrderSend(request,result); trp1=0; } // Modify SL/TP if(result.retcode == TRADE_RETCODE_PLACED || result.retcode == TRADE_RETCODE_DONE) { request.action = TRADE_ACTION_SLTP; do Sleep(100); while(PositionSelect(_Symbol) == false); double positionOpenPrice = PositionGetDouble(POSITION_PRICE_OPEN); if(StopLoss > 0) request.sl = positionOpenPrice + (StopLoss * _Point); if(request.sl > 0) OrderSend(request,result); } } string s = "openPosition=" + (string)openPosition +"\n" + "\n"; s = s + "positionType=" + (string)positionType + "\n" + "\n"; s = s + "position_ticket=" + (string)position_ticket + "\n" + "\n"; s = s + "trp1(rsi)" + (string)trp1 + "\n" + "\n"; s = s + "trp3(MA)" + (string)trp3 + "\n" + "\n"; s = s + "rsi1" + (string)rsi1[0] + "\n" + "\n"; s = s + "rsi2" + (string)rsi2[0] + "\n" + "\n"; s = s + "ma1" + (string)ma1[0] + "\n" + "\n"; s = s + "ma2" + (string)ma2[0] + "\n" + "\n"; s = s + "s_21magic" + (string)s_21magic + "\n" + "\n"; s = s + "pos_magic" + (string)pos_magic + "\n" + "\n"; s = s + (string)TimeCurrent(); Comment(s); }
few lines are just left for easy modification like variable twt has no use here...nor does this one use sl or tp. Thanks
few lines are just left for easy modification like variable twt has no use here...nor does this one use sl or tp. Thanks
All values are obtained without any delay - the tester is working correctly.
Your mistakes:
- You probably (99% probability) ran the test at maximum speed - at maximum speed the 'Comment' function slows down the interface a lot - you need to know.
- You haven't used 'ChartRedraw'
All values are obtained without any delay - the tester is working correctly.
Your mistakes:
- You probably (99% probability) ran the test at maximum speed - at maximum speed the 'Comment' function slows down the interface a lot - you need to know.
- You haven't used 'ChartRedraw'
this is an issue during Live testing. During every tick or every tick based on real ticks or 1min OHLC there is no lag in tester. with Open prices only modelling i see this lag in tester.
but this shouldn't be happening during live testing but it is.
I just added a chartredraw() function as the last line in ontick() fn. It didn't help.
I will stop this comparison if you can still confirm that the EA is trading based on the real values of indicator and not the garbage values printed on screen. but this shouldn't be correct as the value used for calculation must be the one being printed.
this is an issue during Live testing. During every tick or every tick based on real ticks or 1min OHLC there is no lag in tester. with Open prices only modelling i see this lag in tester.
but this shouldn't be happening during live testing but it is.
I just added a chartredraw() function as the last line in ontick() fn. It didn't help.
I will stop this comparison if you can still confirm that the EA is trading based on the real values of indicator and not the garbage values printed on screen. but this shouldn't be correct as the value used for calculation must be the one being printed.
If you get a value, you must immediately use it.
Thanks thanks......issue resolved
By the way, can you help me with this pls.....
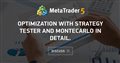
- 2021.08.10
- www.mql5.com
If you get a value, you must immediately use it.
Also can you help me pave my future steps to writing advanced EAs?
I am currently learning about custom indicators, specially those which are not inbuilt in mt5 cause I feel like the inbuilt ones are already being exploited to the limit where they cant be as profitable.
money management modules don't entertain me.
what could the other things be i should learn about in this field???

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
My EA has an RSI indicator whose vale is updated onscreen via print function.
However i just noticed that the value of RSI is vague when compared to that shown in indicator window. Perhaps it is lagging.
My best guess is that it is happening because the ontick function is not continuously updating it. Is it true? If so then how can it be solved? can the ontimer function in this situation updating the RSI value each second or millisecond? or any other way?
I have decent i7 8gb desktop so there is no question of these 100 lines EA's stressing my processor.
this lag is more in strategy tester and around 0.5 in live EA. Moreover i don't know if this is severely affecting my backtests.How to solve this.?