input int SmallSMA=5; input int BigSMA=20; void OnTick() { //we create a string variable for the signal string signal=""; //we calculate the small moving average double SmallMovingAverage1 = iMA(_Symbol, _Period, SmallSMA, 0, MODE_SMA, PRICE_CLOSE, 1); //we calculate the big moving average double BigMovingAverage1 = iMA(_Symbol, _Period, BigSMA, 0, MODE_SMA, PRICE_CLOSE, 1); //we calculate the small moving average double SmallMovingAverage2 = iMA(_Symbol, _Period, SmallSMA, 0, MODE_SMA, PRICE_CLOSE, 2); //we calculate the big moving average double BigMovingAverage2 = iMA(_Symbol, _Period, BigSMA, 0, MODE_SMA, PRICE_CLOSE, 2); // If BigMovingAverage > SmallMovingAverage if (BigMovingAverage1 > SmallMovingAverage1) // If BigMovingAverage < SmallMovingAverage before if (BigMovingAverage2 < SmallMovingAverage2) { //Set the signal variable to sell signal="sell"; } // If BigMovingAverage < SmallMovingAverage if (BigMovingAverage1 < SmallMovingAverage1) // If BigMovingAverage > SmallMovingAverage before if (BigMovingAverage2 > SmallMovingAverage2) { //Set the signal variable to buy signal="buy"; } // Buy Order if (signal=="buy" && OrdersTotal()==0) { RefreshRates(); int ticket = OrderSend(_Symbol,OP_BUY,Lots,Ask,3,0,Ask+150*_Point,NULL,0,0,Green); } else; // Sell Order if (signal=="sell" && OrdersTotal()==0) { RefreshRates(); int ticket =OrderSend(_Symbol,OP_SELL,Lots,Bid,3,0,Bid-150*_Point,NULL,0,0,Red); } // Chart Output for the signal Comment ("The Current Signal is: ",signal); } //+------------------------------------------------------------------+
Hi, before the OrderSend command you may recalculate "Lots" to a random number between 10 and 100. I would do that on several steps to make it easier to follow, but once you recognizes how the MathRand function works, you would just code it straightforward in a single line:
double getRandomFrom0to32767 = MathRand(); // Standard "random" output of 0 to 32767
double lotsFrom0to1 = getRandomFrom0to32767 / 32767.0; // Normalize it from 0 to 1
double lotsMultiplied90x = lotsFrom0to1 * 90; // Expand the range from 10 to 100 (that is 90 width)
double yourRandomLots = lotsMultiplied90x + 10; // Move the range to a minimum of 10
Lots = MathRound(yourRandomLots); // Round it to the closest integer (no decimal places)
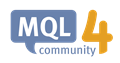
Hi, before the OrderSend command you may recalculate "Lots" to a random number between 10 and 100. I would do that on several steps to make it easier to follow, but once you recognizes how the MathRand function works, you would just code it straightforward in a single line:
double getRandomFrom0to32767 = MathRand(); // Standard "random" output of 0 to 32767
double lotsFrom0to1 = getRandomFrom0to32767 / 32767.0; // Normalize it from 0 to 1
double lotsMultiplied90x = lotsFrom0to1 * 90; // Expand the range from 10 to 100 (that is 90 width)
double yourRandomLots = lotsMultiplied90x + 10; // Move the range to a minimum of 10
Lots = MathRound(yourRandomLots); // Round it to the closest integer (no decimal places)
Oh. Hmmm....i'm still quite new to this though. But interested in EA. lol....was looking through MathRand but couldn't figure it out.
I'll give it a try. Thanks a lot.
Do u know anything about looping the OrderSend maybe 10 - 20 times?
Say i close the entry manual and the EA takes another entry. Look through quite a few examples but its all very complicated.
Hi, error 131 is "ERR_INVALID_TRADE_VOLUME" ... which means the number sent to the broker as the lot quantity does match the broker limits. i.e. If I use "0.01" but the broker minimum is "0.10". Or if I use "0.12" but the broker step increase for lots is "0.10" hence I have to go for "0.10" or "0.20". Or if I use "0.1111" but only 2 decimals are accepted. Or if I use "123.00" but the broker limit is "10.00". Try displaying in the log the amount used in the OrderSend command. i.e. Print("Lots=", Lots);
Oh. Hmmm....i'm still quite new to this though. But interested in EA. lol....was looking through MathRand but couldn't figure it out.
I'll give it a try. Thanks a lot.
Do u know anything about looping the OrderSend maybe 10 - 20 times?
Say i close the entry manual and the EA takes another entry. Look through quite a few examples but its all very complicated.
Hi, to the MathRand command, you may play a bit to get to learn .... i.e.
int OnInit()
{
for (int k=0; k<100; k++)
{
Print(MathRand());
// then you may add here whatever idea you would like to test to play how you can get what you have in mind
}
return(INIT_SUCCEEDED);
}
I always write quick EA's just with the On Init section to get to learn, or to assemble a new function where I could test it thru loops to make sure is returns the desired value for every possible scenario before I add it to my real EA.
To your question about "looping the OrderSend" ... yeah, I learnt it from a money manager, he suggested me rather than creating a single order of 0.20 lots, better create 4 of 0.05. Then it's easier to perform "partial closure" if he thinks it's time to take some Profit, but risking another part if it grows more. Then, I just split the former lot into 4 and put the "OrderSend" in a loop.
But .... programming validations ... don't forget to always check return code after an OrderSend. i.e. "int justChecking = GetLastError()" ... because there could be any circumstance where your price is no longer valid, market closed, or just internet delays / temporary disconnections, etc. .... you could take action on the error. Usually I just code a loop to attempt "OrderSend" 3 times. You may play with that in a demo account to get to learn more details that a real EA would need to take into account.
Hi, to the MathRand command, you may play a bit to get to learn .... i.e.
I always write quick EA's just with the On Init section to get to learn, or to assemble a new function where I could test it thru loops to make sure is returns the desired value for every possible scenario before I add it to my real EA.
To your question about "looping the OrderSend" ... yeah, I learnt it from a money manager, he suggested me rather than creating a single order of 0.20 lots, better create 4 of 0.05. Then it's easier to perform "partial closure" if he thinks it's time to take some Profit, but risking another part if it grows more. Then, I just split the former lot into 4 and put the "OrderSend" in a loop.
But .... programming validations ... don't forget to always check return code after an OrderSend. i.e. "int justChecking = GetLastError()" ... because there could be any circumstance where your price is no longer valid, market closed, or just internet delays / temporary disconnections, etc. .... you could take action on the error. Usually I just code a loop to attempt "OrderSend" 3 times. You may play with that in a demo account to get to learn more details that a real EA would need to take into account.
I see, thank you so much. I have teached me a lot.
I was interested in EA many years back. But real life work took too much of my time and I forgotten all about it.
Not really a programmer myself. Only able to understand simple stuff for MQL5.
Thanks again.!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
How do i do a random lot size script on a simple MA EA?
Lets say for the fun of back test 10 - 100 Lot size