- Invalid volume EA
- PolyFitScalper
- Scalp_net
-
Why can't you fix your code?
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
-
Compute the new value, then check that you are moving the existing value at least a tick.
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum 2014.08.03 - ERR_NO_RESULT
You Server Change the SL to X It is at X! Change the SL to X It is at X! Change the SL to X You are insane Insanity: doing the same thing over and over again and expecting different results.
Unknown
Hey, most probably the issue comes from your trail function. As William Roeder said, the issue is because you try to modify the SL to a certain level, but the SL is already at this level and the server returns an error, because there is nothing to modify.
What you can do is just add one more condition to the function to check whether the SL is already at this level, if it is you dont modify it.
I hope i made it easy to understand
Best Regards mate
Stanislav Ivanov thank you
I will look into this solution
Let me be brief...
On your code add:
extern double MinPipsToModify=1; /* This value should never be less than 1. It's the number of pips that must change before OrderModify() is called upon. */
Below external inputs put...
double MPTM=MinPipsToModify*10*Point;
On your code where you have...
if (Bid-OrderOpenPrice()>TrailingStop*10*Point){ //Modify modify order... }
AND where you have...
if (OrderOpenPrice()-Ask>TrailingStop*10*Point){ //Modify modify order... }
Replace with, respectively,
if (Bid-OrderOpenPrice()>((TrailingStop*10*Point)+MPTM){ //Modify modify order... }
and...
if (OrderOpenPrice()-Ask>((TrailingStop*10*Point)+MPTM){ //Modify modify order... }
This has solved the OrderModify () ERROR=1 on my EA. THIS SHOULD HELP ALL THOSE QUERIES I HAVE SEEN ONLINE.
To understand it more:
Let's say EURUSD is at 1.17501 and then price rises to 1.17503, the 1.17503 is greater than 1.17501 but OrderModify () does not recognise fractional pips. As such it looks at 1.17501 as equal to 1.17503. Even if price rises to 1.17509 the function still equates 1.17501==1.17509. It will continue to throw the error until price hits 1.1751.
Consequently, 1.17513==1.17515==1.17518 according to OrderModify().
GIVE ME A HIGH FIVE ONCE IT SOLVES YOUR QUERY.
Thank you ,my friend !
You are the best
You solve a problem that faces many of us !
Good day !
Gerard
Here is the complete program.
I corrected it, according to your effective and educational guidelines.
The program is now working perfectly.
Error 1 is finally gone.
Thank you very much for your kindness
Gerard
//+------------------------------------------------------------------+ //| Scalper-GG-14.mq4 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict //+------------------------------------------------------------------+ extern string mn="Scalper-GG-14"; double lot; extern int shifthighrecent=5; extern int shiftlowrecent=2; extern int shifthigh=5; extern int shiftlow=7; extern bool trail=true; extern int TrailingStop=2; extern int barnumber =24; extern int barnumberrecent=33; extern int MagicNumber=14072020; //extern double TakeProfit=53; extern double atrMultiple = 3; extern int TrailingStart=21; double stoplevel=(MarketInfo(Symbol(),MODE_STOPLEVEL))/10; int TS=TrailingStart-TrailingStop; extern int volume1=1; extern int volume0=0; extern int adxperiod= 31; extern int adxthreshold=23; extern int rsiperiod=21; extern int rsilower =48; extern int rsiupper =80; extern int Slippage=3; extern int Indicatorperiod = 14; input int MaxSpreadAllow = 10; // Enter Maximum allowed spread extern double LotFactor =60; //lotsize factor extern int ecartask=2; extern int ecartbid=10; extern int Start_Time = 0; // Time to allow trading to start ( hours of 24 hr clock ) 0 for both disables extern int Finish_Time = 0; // Time to stop trading ( hours of 24 hr clock ) 0 for both disables extern double bidbid =600; extern double askask =300; extern int AddPriceGap=1; extern double MinPipsToModify=1; //+------------------------------------------------------------------+ // expert start function //+------------------------------------------------------------------+ int start() { if(TimeHour(TimeCurrent())>=Start_Time && TimeHour(TimeCurrent())<=Finish_Time )return(0); double MyPoint=Point; if(Digits==3 || Digits==5) MyPoint=Point*10; double TheStopLoss=0; double TheTakeProfit=0; double booster = Volume[volume1]> Volume[volume0] &&iADX(Symbol(),0,adxperiod,PRICE_CLOSE,MODE_MAIN,0)> adxthreshold &&iRSI(Symbol(),0,rsiperiod,PRICE_CLOSE,0)>rsilower && iRSI(Symbol(),0,rsiperiod,PRICE_CLOSE,0)<rsiupper ; static datetime tmeBar0; bool newBar = false; if ( tmeBar0 != Time[0] ) { tmeBar0 = Time[0]; newBar = true; } // Get Ask and Bid for the currency double ask = MarketInfo ( Symbol(), MODE_ASK ); double bid = MarketInfo ( Symbol(), MODE_BID ); double Spread = MarketInfo(Symbol(), MODE_SPREAD); // This will Obtain broker Spread for current pair if(Spread > 0 && Spread <= MaxSpreadAllow) // This part compares broker spread with maximum allowed spread, and refuse trade if maxSpread is exceeded if ( OrdersTotal () == 0 ) if(AccountFreeMargin()>(1000*(AccountEquity() * 0.01 /LotFactor))) if(booster) //+------------------------------------------------------------------+ // Dynamic stoploss and takeprofit //+------------------------------------------------------------------+ double ema = iMA(NULL, 0, 300, 0, MODE_EMA, PRICE_CLOSE, 0); double atr = iATR(NULL, 0, 3, 0); double StopLoss = (atr * atrMultiple / Point); double TakeProfit = StopLoss; //+------------------------------------------------------------------+ //Here is how Keltner Channels are calculated: //Upper Band = EMA + (ATR x multiplier) //Middle Band = EMA //Lower Band = EMA - (ATR x multiplier) //The EMA period can be set to anything you want. For day trading, an EMA of 15 to 40 is typical. //A common multiplier for the ATR is 2, meaning the upper band will be plotted 2 x ATR above the EMA, and the lower band will be plotted 2 x ATR below the EMA. // //UpperBand=ema+(atr*atrmultiplier); //MiddleBand=ema; //LowerBand=ema-(atr*atrmultiplier); // // // //+------------------------------------------------------------------+ new_del () ; if( TotalOrdersCount()==0 ) { int result=0; //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ // Here is your open buy rule//Ici votre strategie d'achat double HighOfLastBarsrecent = iHigh ( Symbol (), PERIOD_M1 , iHighest ( Symbol (), PERIOD_M1 , MODE_HIGH, barnumberrecent , shifthighrecent )); double LowOfLastBarsrecent = iLow ( Symbol (), PERIOD_M1 , iLowest ( Symbol (), PERIOD_M1 , MODE_LOW, barnumberrecent , shiftlowrecent )); double HighOfLastBars = iHigh ( Symbol (), PERIOD_M1 , iHighest ( Symbol (), PERIOD_M1 , MODE_HIGH, barnumber , shifthigh )); double LowOfLastBars = iLow ( Symbol (), PERIOD_M1 , iLowest ( Symbol (), PERIOD_M1 , MODE_LOW, barnumber , shiftlow )); double AverageBars=(HighOfLastBars +LowOfLastBars)/2 ; double PartialLow=((LowOfLastBars+AverageBars)/2); double PartialHigh = ((HighOfLastBars+AverageBars)/2); // Calculate a channel on Moving Averages, and check the price double iMaLow = iMA ( Symbol(), PERIOD_M1, Indicatorperiod, 0, MODE_LWMA, PRICE_LOW, 0 ); double iMaHigh = iMA ( Symbol(), PERIOD_M1, Indicatorperiod, 0, MODE_LWMA, PRICE_HIGH, 0 ); double iMaAverage = (iMaHigh + iMaLow)/2; double PartialiMalow = (iMaLow + iMaAverage) / 2.0; double PartialiMahigh =(iMaHigh + iMaAverage) / 2.0; double UpperPart=(PartialiMahigh+PartialHigh)/2; double LowerPart= ( PartialiMalow+ PartialLow)/2; if (((bid>= PartialiMahigh ) &&(bid>= PartialHigh ) &&(booster))) if (bid>= HighOfLastBars ) if (HighOfLastBarsrecent>= HighOfLastBars) //+------------------------------------------------------------------+ // Dynamic stoploss and takeprofit //+------------------------------------------------------------------+ double ema = iMA(NULL, 0, 300, 0, MODE_EMA, PRICE_CLOSE, 0); double ema = iMA(NULL, 0, 300, 0, MODE_EMA, PRICE_CLOSE, 0); if (Ask + askask * Point < ema) //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ { result=OrderSend(Symbol(),OP_BUYSTOP, NR(Lot_Volume()),Ask +ecartask*Point,5,PartialLow -StopLoss*Point-AddPriceGap,PartialHigh +TakeProfit*Point+AddPriceGap,"Scalper-GG-9",MagicNumber,0,clrLimeGreen); if(result>0) { TheStopLoss=0; TheTakeProfit=0; if(TakeProfit>0) TheTakeProfit=Ask+TakeProfit*MyPoint; if(StopLoss>0) TheStopLoss=Ask-StopLoss*MyPoint; if( OrderSelect(result,SELECT_BY_TICKET)) bool modif1= OrderModify(OrderTicket(),OrderOpenPrice(),NormalizeDouble(TheStopLoss,Digits),NormalizeDouble(TheTakeProfit,Digits),0,Green); } return(0); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ // Here is your open Sell rule//Ici votre strategie de vente if (((PartialiMalow <= bid)&&(PartialLow <= bid)&&(booster))) if (LowOfLastBars<= bid) if( LowOfLastBarsrecent<=LowOfLastBars ) //+------------------------------------------------------------------+ // Dynamic stoploss and takeprofit //+------------------------------------------------------------------+ if (Bid - bidbid * Point > ema) //+------------------------------------------------------------------+ { result=OrderSend(Symbol(),OP_SELLSTOP, NR(Lot_Volume()),Bid-ecartbid*Point,5,PartialHigh +StopLoss*Point+AddPriceGap,PartialLow -TakeProfit*Point-AddPriceGap,"Scalper-GG-14",MagicNumber,0,clrRed); if(result>0) { TheStopLoss=0; TheTakeProfit=0; if(TakeProfit>0) TheTakeProfit=Bid-TakeProfit*MyPoint; if(StopLoss>0) TheStopLoss=Bid+StopLoss*MyPoint; if( OrderSelect(result,SELECT_BY_TICKET)) bool modif2= OrderModify(OrderTicket(),OrderOpenPrice(),NormalizeDouble(TheStopLoss,Digits),NormalizeDouble(TheTakeProfit,Digits),0,Green); } return(0); } } //+------------------------------------------------------------------+ // Trailing Start //+------------------------------------------------------------------+ double Pip=Point*10; if(TS<stoplevel) TrailingStart=(int)stoplevel+TrailingStop; if(!IsTradeAllowed()){ MessageBox("This Expert Advisor requires Auto Trading. Please reload the EA or right click on"+ "\nthe chart to bring up the inputs window. Under the common tab check the box to"+ "\nallow live trading"); Sleep(50000); } if(!IsExpertEnabled()){ MessageBox("This Expert Advisor requires Auto Trading. Please click the button at the top"); Sleep(50000); } int ticket=0,buy_ticket=0,sell_ticket=0; for(int i=OrdersTotal()-1; i>=0; i--) if(OrderSelect(i,SELECT_BY_POS) && OrderSymbol()==Symbol()){ ticket++; if(OrderType()==OP_BUY) buy_ticket=OrderTicket(); if(OrderType()==OP_SELL) sell_ticket=OrderTicket(); } if(OrderType()==OP_BUY){ if(OrderSelect(buy_ticket,SELECT_BY_TICKET)) { if(Bid - OrderOpenPrice() > TrailingStop *10* MarketInfo(OrderSymbol(),MODE_POINT)) if((Bid-OrderOpenPrice())>(TrailingStart*Pip)) { if(((OrderStopLoss() < Bid - TrailingStop *10* MarketInfo(OrderSymbol(), MODE_POINT))&& ((Bid-OrderOpenPrice())>(Point*TrailingStop)))|| (OrderStopLoss()==0)) { bool modify1=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT),OrderTakeProfit(),clrGreenYellow); Print("Buy = ",GetLastError()); return(0); RefreshRates(); } } } } if(OrderType()==OP_SELL) { if(OrderSelect(sell_ticket,SELECT_BY_TICKET)) { if(OrderOpenPrice()-Ask>TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT)) if((OrderOpenPrice()-Ask)>(TrailingStart*Pip)) { if(((OrderStopLoss()>Ask+TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT))&& ((OrderOpenPrice()-Ask)>(Point*TrailingStop)))|| (OrderStopLoss()==0)) bool modify2=OrderModify(OrderTicket(),OrderOpenPrice(), Ask+TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT),OrderTakeProfit(),clrGreenYellow); if(OrderModify(OrderTicket(),OrderOpenPrice(),Ask+(TrailingStop*Pip),OrderTakeProfit(),Red)) Print("Sell = ",GetLastError()); return(0); RefreshRates(); } } } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ return(0); }//start int TotalOrdersCount() { int result=0; for(int i=0;i<OrdersTotal();i++) { if(OrderSelect(i,SELECT_BY_POS ,MODE_TRADES)) if (OrderMagicNumber()==MagicNumber) result++; } return (result); } //+------------------------------------------------------------------+ // ( to close pending order) //+------------------------------------------------------------------+ int new_del() { if(trail) trail(); int i,a; int total = OrdersTotal(); string comentario,par; for (i=total-1; i >=0; i--) { if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)) if (OrderType()==OP_BUY || OrderType()==OP_SELL) { for (a=total-1; a >=0; a--) { if(OrderSelect(a,SELECT_BY_POS,MODE_TRADES)) if(OrderType()==OP_SELLSTOP) { bool modify1= OrderDelete(OrderTicket()); Print("Deleting SELL_STOP"," Ordertype:",OrderType()); return(1); } if(OrderType()==OP_BUYSTOP) { bool modify2= OrderDelete(OrderTicket()); Print("Deleting BUY_STOP"," Ordertype:",OrderType()); return(1); } } } } return ( 0 ); } //+------------------------------------------------------------------+ //| Trailing Stoploss after Breakeven | //+------------------------------------------------------------------+ void trail() { for (int i = OrdersTotal()-1; i >= 0; i --) { if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)) if(OrderSymbol()==Symbol()) { if(OrderType()==OP_BUY) { double MPTM=MinPipsToModify*10*Point; if(Bid - OrderOpenPrice() > TrailingStop *10* MarketInfo(OrderSymbol(),MODE_POINT)+ MPTM) { if(((OrderStopLoss() < Bid - TrailingStop *10* MarketInfo(OrderSymbol(), MODE_POINT))&& ((Bid-OrderOpenPrice())>(Point*TrailingStop)))|| (OrderStopLoss()==0)) { bool modify1=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT),OrderTakeProfit(),clrGreenYellow); } } } else if(OrderType()==OP_SELL) { double MPTM=MinPipsToModify*10*Point; if(OrderOpenPrice()-Ask>TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT)+MPTM) { if(((OrderStopLoss()>Ask+TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT))&& ((OrderOpenPrice()-Ask)>(Point*TrailingStop)))|| (OrderStopLoss()==0)) { bool modify2=OrderModify(OrderTicket(),OrderOpenPrice(), Ask+TrailingStop*10*MarketInfo(OrderSymbol(),MODE_POINT),OrderTakeProfit(),clrGreenYellow); } } } } } } //+------------------------------------------------------------------+ //Calculates Lot Size based on balance and factor //+------------------------------------------------------------------+ double NR(double thelot) { double maxlots=MarketInfo(Symbol(),MODE_MAXLOT), minilot=MarketInfo(Symbol(),MODE_MINLOT), lstep=MarketInfo(Symbol(),MODE_LOTSTEP); double lots=lstep*NormalizeDouble(thelot/lstep,0); lots=MathMax(MathMin(maxlots,lots),minilot); return (lots); } //+------------------------------------------------------------------+ double Lot_Volume() { lot=AccountEquity() * 0.01 /LotFactor ; return(lot); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+
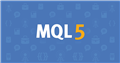
Hey, most probably the issue comes from your trail function. As William Roeder said, the issue is because you try to modify the SL to a certain level, but the SL is already at this level and the server returns an error, because there is nothing to modify.
What you can do is just add one more condition to the function to check whether the SL is already at this level, if it is you dont modify it.
I hope i made it easy to understand
Best Regards mate
thank you stanislav
you solved my problem.god bless you

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use