Immediately a mistake.
Read help:
Returns the requested order property, pre-selected using OrderGetTicket or OrderSelect. Order property must be of the datetime, int type. There are 2 variants of the function.
The function returns the requested property of an open position, pre-selected using PositionGetSymbol or PositionSelect. The position property should be of datetime, int type. There are 2 variants of the function.
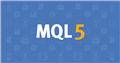
Documentation on MQL5: Trade Functions / OrderGetInteger
- www.mql5.com
Returns the requested order property, pre-selected using OrderGetTicket or OrderSelect. Order property must be of the datetime, int type. There are 2 variants of the function. 2. Returns true or false depending on the success of the function. If successful, the value of the property is...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello I am learning mql5 language. I have designed a code to setup pending orders in gird when a candle is bigger than the predefined range. If candle is bullish then place 1 sell trade and then set a specific amount of sell limit orders based on open price of the Sell trade and opposite for buys.
When anyone of the trade reaches the TP I want to close all orders and positions. Please check my code and help me improve it.