When you are dealing with doubles in your array and the csv file, you should use FileReadNumber instead of FileReadString.
You are probably not reading enough values from the file. You don't check for end of file and are trying to access 2400 elements in your array regardless of its size.
I'd add a '#property strict' before going on.
By the way your code is poorly formatted and variables are not named consistently. You're probably having a lot of issues elsewhere.
Thanks for your reply.
I tried FileReadNumber() elsewhere in my code with no difference, but will try it here. I have also used FileIsEnding(), this never works for me, I've also read it has some bug.
I should mention that it reads fine as I can Print() any line number no problem, also when you Write, it deletes the file and overwrites, the file file is the same as before except
for a few updated scores below line 300. You are right there is a lot of room for improvement, but everything else works fine.
The thing is that everything works fine upto line 300, but not further, so that is the real issue.
If you don't initialize then next_c is 0 by default. Anyway Strict does not seem to care. It counts fine. That is not where my issue is.
Where do you get that idea from?
https://www.mql5.com/en/docs/basis/variables/initialization
Any variable can be initialized during definition. If a variable is not initialized explicitly, the value stored in this variable can be any. Implicit initialization is not used.
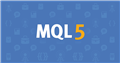
- www.mql5.com
Thanks Keith, "Missed initializing sequences are considered equal to 0." I did'nt read properly... relates to arrays!
Appreciate the advice. As far as I can tell this wont affect my actual problem, but will no doubt improve my code.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to write to a csv file, but can't write past 280 lines. I am trying to write upto 2400 or more lines, only one double per line.
The lines in the file are scores related to same line number in another file the same size.
I read in the scores, modify them then write them all back in. As far as I can tell I can read the whole file, I assume I am writing
the whole file back in as the file is replaced (overwritten) each time. I write the array then the array is written to the file
using a loop. Lines below 280 are updated fine, after that they remain unchanged at 0.07, the default number in the file.
Buy_Pattern_score[2400][1] and var_arrayB00[2400][1] are both int and declared in global scope.
Before you ask the array is a 2D array because I want one score per line in the scv file as they must correspond with the other csv file line number.
An example of six lines of the Buy_Pattern_score.csv file
I would really appreciate some help or advice. I have scoured the forum, searched the documentation, not understood some things.
I'm sure there is always an answer , mostly simple explanation and a solution to this.
I would like to read and write all 2400 lines in the file. I do this again in the EA a few times, different files.
Thanks in advance.