thanks for your quick reply. Yes thats solve the error problem!
Do u have any idea why it doenst open any trades? Based on the indicator seetings it should?! Its a simple logic -> check H4 if H4 is positive and H1 gives a buy signal = buy (vice verca)
Thansk in advance,
Max
I have no idea why it doesn't work.
You should check if you read correct values from the indicators, and if you do, check if your decision making logic is correct.
Add series of Print() statements in your code and print out values from the indicator and decision making code and/or run it through debugger using history data.
The best way is to watch Journal.
I had Critical Error ... it makes me crazy
but Journal exactly indicate for you what is the error source.
I have no idea why it doesn't work.
You should check if you read correct values from the indicators, and if you do, check if your decision making logic is correct.
Add series of Print() statements in your code and print out values from the indicator and decision making code and/or run it through debugger using history data.
handle1 = iCustom(NULL, PERIOD_H4, "Kalman velocity (mtf)","Current time frame",1,PRICE_CLOSE,"Interpolate data when in multi time frame"); handle2 = iCustom(NULL, PERIOD_CURRENT, "Kalman velocity (mtf)","Current time frame",1,PRICE_CLOSE,"Interpolate data when in multi time frame");The CopyBuffer error comes from this part of the code, but the history is there:
double GetIndicator(int handle, int buffer_num, int index) { //--- array for the indicator values double arr[]; //--- obtain the indicator value in the last two bars if (CopyBuffer(handle, buffer_num, 0, index+1, arr) <= 0) { Sleep(200); for(int i=0; i<100; i++) { if (BarsCalculated(handle) > 0) break; Sleep(50); } int copied = CopyBuffer(handle, buffer_num, 0, index+1, arr); if (copied <= 0) { Print("CopyBuffer failed. Maybe history has not download yet? Error = ", GetLastError()); return -1; } else return (arr[index]); } else { return (arr[index]); } return 0; }
I could not find a possible solution so far. Any tips how to solve/adjust it?
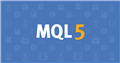
- www.mql5.com
I think you were right with the indicator values. I didnt use the iCustom function in a correct way, but now I have a CopyBuffer error, becasue I changed the iCustom function to: The CopyBuffer error comes from this part of the code:
I could not find a possible solution so far. Any tips how to solve/adjust it?
What error?
What error?
2020.05.20 17:24:58.000 Core 1 2019.04.18 18:14:52 CopyBuffer failed. Maybe history has not download yet? Error = 4807
From the documentation:
ERR_INDICATOR_WRONG_HANDLE |
4807 |
Wrong indicator handle |
You probably have an error in iCustom() call.
Check if handle is INVALID_HANDLE. Read documentation on iCustom()
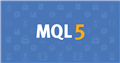
- www.mql5.com
From the documentation:
ERR_INDICATOR_WRONG_HANDLE |
4807 |
Wrong indicator handle |
You probably have an error in iCustom() call.
Check if handle is INVALID_HANDLE. Read documentation on iCustom()
double GetIndicator(int handle, int buffer_num, int index) { //--- array for the indicator values double arr[]; //--- obtain the indicator value in the last two bars if (CopyBuffer(handle, buffer_num, 0, index+1, arr) <= 0) { Sleep(200); for(int i=0; i<100; i++) { if (BarsCalculated(handle) > 0) break; Sleep(50); } int copied = CopyBuffer(handle, buffer_num, 0, index+1, arr); if (copied <= 0) { Print("CopyBuffer failed. Maybe history has not download yet? Error = ", GetLastError()); return -1; } else return (arr[index]); } else { return (arr[index]); } return 0; }
Maybe because it calls only handle and not a specific one, because I have 2 handles (H1 and H4).
Could that
be the reason?
You created handles for the H4 and current (presumably not H4) timeframe.
- your code calls GetIndicator() with value of the index variable set to "current" and "current+1"
- variable current is set to 0 and I didn't find any place where it is modified
- that means that you read current value on H4 timeframe and value of the previous bar on the current timeframe
- Is that what you want?
- For debugging purposes, print value of the arr[index] before you return it and check if you got the value you expected
- Check that you read all values from the same end - check that you consistently use xxxAsSeries() functions

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi all,
I try to (partly) code my manuel trading strategy to be automated, but
1) it doesnt open any trades
2) with the backtest it opens two charts H1 and H4, but for the stragey it only needs to check the value of the indicator on a H4 chart
3) during the backtest I get the Errorcode "OnTick critical error"
Does anyone have an idea why do I have these problems with my EA?
I really appreciate your help!