Maximilian Goldbecker :
Please use the button
.
This time I fixed and inserted the code correctly. Next time - I will delete.
Maximilian Goldbecker :
Hi all,
for the last couple of days I tried to code an EA for my manuel trading system which is based on the Kalman Velocity indicator. I dont get any error or warnings in Metaeditor, but the EA doesnt open any position.
Could someone help me here?
The idea behind the EA is a simple cross over the zero line of the indicator - Close Indicator+2 < 0 and Close Indicator +1 > 0 = Buy Market next Bar/Candelstick.
Hi all,
for the last couple of days I tried to code an EA for my manuel trading system which is based on the Kalman Velocity indicator. I dont get any error or warnings in Metaeditor, but the EA doesnt open any position.
Could someone help me here?
The idea behind the EA is a simple cross over the zero line of the indicator - Close Indicator+2 < 0 and Close Indicator +1 > 0 = Buy Market next Bar/Candelstick.
You made a mistake: you CREATE a new indicator handle on EVERY TIC! This is a gross mistake!
void OnTick() { double Kalman[]; int KalmanVelocity = iCustom(NULL, PERIOD_CURRENT,"Kalman"); CopyBuffer(KalmanVelocity,0,0,1,Kalman); if(Kalman[0] == 0) return; if ((Kalman[+1] > 0)&& Kalman[+2] < 0){ Print(Kalman[0]); if(!isLongTrade) isLongTrade = executeLong();} else if ((Kalman[+1] < 0) && Kalman[+2] > 0) { Print(Kalman[0]); if(!isShortTrade) isShortTrade = executeShort(); } }
The indicator head MUST BE CREATED ONCE (this is done in OnInit)
Vladimir Karputov:
Hi Vladimir,You made a mistake: you CREATE a new indicator handle on EVERY TIC! This is a gross mistake!
The indicator head MUST BE CREATED ONCE (this is done in OnInit)
thanks a lot for your quick help!
I completely coded the EA in a new way with some help. Everything works fine, but I get a warning (return value of "OrderSend" should be checked). I could not find any solution that works so far, maybe someone could help me with this? I highlighted the code line below.
void TechnicalAnalysis2x6() { if ((GetIndicator(handle3,0,current+1) < 0) && (GetIndicator(handle4,0,current+2) > 0)) { SellOrder8(); } } void SellOrder8() { //--- prepare a request MqlTradeRequest request; ZeroMemory(request); request.action = TRADE_ACTION_DEAL; // setting a deal order request.magic = 1; // ORDER_MAGIC request.symbol = Symbol(); // symbol request.volume = Lots8; // volume in lots request.price = Bid(); request.sl = Bid() + Stoploss8*PipValue*Point(); // Stop Loss specified request.tp = Bid() - Takeprofit8*PipValue*Point(); // Take Profit specified request.deviation = 10; // deviation in points request.type = ORDER_TYPE_SELL; request.comment = "Order"; MqlTradeResult result; ZeroMemory(result); OrderSend(request,result); // check the result if (!IsError(result, __FUNCTION__)) { }
Maximilian Goldbecker :
Hi Vladimir,
thanks a lot for your quick help!
I completely coded the EA in a new way with some help. Everything works fine, but I get a warning (return value of "OrderSend" should be checked). I could not find any solution that works so far, maybe someone could help me with this? I highlighted the code line below.
Hi Vladimir,
thanks a lot for your quick help!
I completely coded the EA in a new way with some help. Everything works fine, but I get a warning (return value of "OrderSend" should be checked). I could not find any solution that works so far, maybe someone could help me with this? I highlighted the code line below.
Show your function GetIndicator.
double GetIndicator(int handle, int buffer_num, int index) { //--- array for the indicator values double arr[]; //--- obtain the indicator value in the last two bars if (CopyBuffer(handle, buffer_num, 0, index+1, arr) <= 0) { Sleep(200); for(int i=0; i<100; i++) { if (BarsCalculated(handle) > 0) break; Sleep(50); } int copied = CopyBuffer(handle, buffer_num, 0, index+1, arr); if (copied <= 0) { Print("CopyBuffer failed. Maybe history has not download yet? Error = ", GetLastError()); return -1; } else return (arr[index]); } else { return (arr[index]); } return 0;
Maximilian Goldbecker:
Hi Vladimir,
thanks a lot for your quick help!
I completely coded the EA in a new way with some help. Everything works fine, but I get a warning (return value of "OrderSend" should be checked). I could not find any solution that works so far, maybe someone could help me with this? I highlighted the code line below.
Hi Vladimir,
thanks a lot for your quick help!
I completely coded the EA in a new way with some help. Everything works fine, but I get a warning (return value of "OrderSend" should be checked). I could not find any solution that works so far, maybe someone could help me with this? I highlighted the code line below.
Validation example in Trade Operation Types
#define EXPERT_MAGIC 123456 // MagicNumber of the expert //+------------------------------------------------------------------+ //| Opening Buy position | //+------------------------------------------------------------------+ void OnStart() { //--- declare and initialize the trade request and result of trade request MqlTradeRequest request={0}; MqlTradeResult result={0}; //--- parameters of request request.action =TRADE_ACTION_DEAL; // type of trade operation request.symbol =Symbol(); // symbol request.volume =0.1; // volume of 0.1 lot request.type =ORDER_TYPE_BUY; // order type request.price =SymbolInfoDouble(Symbol(),SYMBOL_ASK); // price for opening request.deviation=5; // allowed deviation from the price request.magic =EXPERT_MAGIC; // MagicNumber of the order //--- send the request if(!OrderSend(request,result)) PrintFormat("OrderSend error %d",GetLastError()); // if unable to send the request, output the error code //--- information about the operation PrintFormat("retcode=%u deal=%I64u order=%I64u",result.retcode,result.deal,result.order); } //+------------------------------------------------------------------+
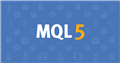
Documentation on MQL5: Constants, Enumerations and Structures / Trade Constants / Trade Operation Types
- www.mql5.com
Trading is done by sending orders to open positions using the OrderSend() function, as well as to place, modify or delete pending orders. Each trade order refers to the type of the requested operation. Trading operations are described in the ENUM_TRADE_REQUEST_ACTIONS enumeration...
Thanks it solved the compile error!
The EA still doesnt trade, but it should based on the indicator values. Do u think it could have something to do with the Indicator? I used the iCustom function, but no errors or warnings.Thanks in advance!

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
for the last couple of days I tried to code an EA for my manuel trading system which is based on the Kalman Velocity indicator. I dont get any error or warnings in Metaeditor, but the EA doesnt open any position.
Could someone help me here?
The idea behind the EA is a simple cross over the zero line of the indicator - Close Indicator+2 < 0 and Close Indicator +1 > 0 = Buy Market next Bar/Candelstick.