Read Help OnTimer:
Note
The Timer event is periodically generated by the client terminal for an EA, which activated the timer using the EventSetTimer() function. Usually, this function is called in the OnInit() function. When the EA stops working, the timer should be eliminated using EventKillTimer(), which is usually called in the OnDeinit() function.
Example:
Sample EA with the OnTimer() handler
Sample EA with the OnTimer() handler //+------------------------------------------------------------------+ //| OnTimer_Sample.mq5 | //| Copyright 2018, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2018, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property description "Example of using the timer for calculating the trading server time" #property description "It is recommended to run the EA at the end of a trading week before the weekend" //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- create a timer with a 1 second period EventSetTimer(1); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- destroy the timer after completing the work EventKillTimer(); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| Timer function | //+------------------------------------------------------------------+ void OnTimer() { //--- time of the OnTimer() first call static datetime start_time=TimeCurrent(); //--- trade server time during the first OnTimer() call static datetime start_tradeserver_time=0; //--- calculated trade server time static datetime calculated_server_time=0; //--- local PC time datetime local_time=TimeLocal(); //--- current estimated trade server time datetime trade_server_time=TimeTradeServer(); //--- if a server time is unknown for some reason, exit ahead of time if(trade_server_time==0) return; //--- if the initial trade server value is not set yet if(start_tradeserver_time==0) { start_tradeserver_time=trade_server_time; //--- set a calculated value of a trade server Print(trade_server_time); calculated_server_time=trade_server_time; } else { //--- increase time of the OnTimer() first call if(start_tradeserver_time!=0) calculated_server_time=calculated_server_time+1;; } //--- string com=StringFormat(" Start time: %s\r\n",TimeToString(start_time,TIME_MINUTES|TIME_SECONDS)); com=com+StringFormat(" Local time: %s\r\n",TimeToString(local_time,TIME_MINUTES|TIME_SECONDS)); com=com+StringFormat("TimeTradeServer time: %s\r\n",TimeToString(trade_server_time,TIME_MINUTES|TIME_SECONDS)); com=com+StringFormat(" EstimatedServer time: %s\r\n",TimeToString(calculated_server_time,TIME_MINUTES|TIME_SECONDS)); //--- display values of all counters on the chart Comment(com); }
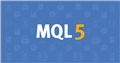
- www.mql5.com
Thank you for your answer Vladimir,
Through my troubleshoot to pinpoint the exact issue, the EventSetTimer got mistakenly moved in the OnTick. But even when calling it in the OnTick, my problem remains the same. Generally, if I make a change in the EA, and run the optimizer, i get proper result EI: A trade opens immediately and never closes. If I restart the platform, then try to run the optimizer again, on different inputs or on a different pair. It doesn't open a trade.
Here's my fixed code. It works fine on the tester. And will usually work fine on the optimizer, until I restart Metatrader 5. The EA had been on the optimizer before, and everything worked fine until build 2345. I tried using older version of my EA that I had run previously without any issue, and it doesn't work anymore. I am trying to learn what changed, so that I can make my EA working again in the optimizer. My only input is TimeSet. Start: 15, Step:5, Stop:20. But even this tiny little EA doesn't enter a trade.
#include <Trade\Trade.mqh> CTrade trade; #include<Trade\SymbolInfo.mqh> CSymbolInfo symbol_; input int TimeSet = 15; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { EventSetTimer(TimeSet); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- destroy timer EventKillTimer(); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- } //+------------------------------------------------------------------+ //| Timer function | //+------------------------------------------------------------------+ void OnTimer() { //--- static bool first = true; if(first) { symbol_.RefreshRates(); double price = symbol_.Ask(); double sl = price - .1; if(trade.Buy(1,NULL,price,sl)) first = false; } }
in the first picture, is the Optimizer run of that EA I have the code above.
the second one is if I click one one of the runs to "Run Single Test"
Yes, there is an error: in build 2345, OnTimer () does not work during optimization.
2020.03.04 09:54:35.404 Terminal MetaTrader 5 x64 build 2345 started for MetaQuotes Software Corp. 2020.03.04 09:54:35.406 Terminal Windows 10 build 19041, Intel Core i3-3120M @ 2.50GHz, 3 / 7 Gb memory, 56 / 415 Gb disk, IE 11, UAC, GMT+2 2020.03.04 09:54:35.406 Terminal C:\Users\barab\AppData\Roaming\MetaQuotes\Terminal\D0E8209F77C8CF37AD8BF550E51FF075
How to check: simple code - in which there are two checks:
//+------------------------------------------------------------------+ //| ProjectName | //| Copyright 2018, CompanyName | //| http://www.companyname.net | //+------------------------------------------------------------------+ #include <Trade\Trade.mqh> //--- CTrade m_trade; // object of CTrade class //--- input parameters input int TimeSet = 15; //--- bool m_first = true; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { if(!EventSetTimer(TimeSet)) { Print("Timer ERROR: ",GetLastError()); return(INIT_FAILED); } m_first=true; //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- destroy timer EventKillTimer(); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- int d=0; } //+------------------------------------------------------------------+ //| Timer function | //+------------------------------------------------------------------+ void OnTimer() { ExpertRemove(); //--- if(m_first) if(m_trade.Buy(1.0,Symbol())) m_first=false; } //+------------------------------------------------------------------+
Optimization:
And the result:
Please note that in OnTimer stands ExpertRemove - if OnTimer worked, then the result picture would be like this:
Forum on trading, automated trading systems and testing trading strategies
OnTimer not working since build 2345
Vladimir Karputov, 2020.03.04 09:06
Yes, there is an error: in build 1222, OnTimer () does not work during optimization.
I assume you mean 2345?
How to check: simple code - in which there are two checks:
//+------------------------------------------------------------------+ //| ProjectName | //| Copyright 2018, CompanyName | //| http://www.companyname.net | //+------------------------------------------------------------------+ #include <Trade\Trade.mqh> //--- CTrade m_trade; // object of CTrade class //--- input parameters input int TimeSet = 15; //--- bool m_first = true; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { if(!EventSetTimer(TimeSet)) { Print("Timer ERROR: ",GetLastError()); return(INIT_FAILED); } m_first=true; //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- destroy timer EventKillTimer(); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- int d=0; } //+------------------------------------------------------------------+ //| Timer function | //+------------------------------------------------------------------+ void OnTimer() { ExpertRemove(); //--- if(m_first) if(m_trade.Buy(1.0,Symbol())) m_first=false; } //+------------------------------------------------------------------+
I understand the purpose of the ExpertRemove()/InItFailed(), as it allows to pinpoint if the issue with the timer, at creation vs Event Calling, I used similar ways to narrow it down. But isn't the Print() already disabled in optimization mode?
Thanks a lot for helping me with the final troubleshoot and getting the developers in on it, very appreciated. Hopefully this will see a fix soon!
I assume you mean 2345?
Yes thank you. Of course, we are talking about build 2345.
If anyone is interested, the issue seems to be solved as of build 2360.
Thanks Vladimir and the devs team, I'm very happy to see the timer work again!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone,
Ever since build 2345, I have not been able to use the optimizer with my EA. The tester works fine, but if I select "Slow complete algo" or "All Symbol in Market Watch" there is no trading at all. I finally got around to explore why that was the case. And after some troubleshooting, I came up to the conclusion that the timer no longer works. It used to before that build. Is there something different I have to do with that build in order to make it work?
The code below doesn't enter a trade. if I copy/paste the code into OnTick, in enters a trade. This is the most dumbed down version of an EA that I could create to show the issue. Am I missing something?