I'm using MT5 hedging account and I'm trying to open an opposite market order (hedging order). I'm using MT5 hedging platform. The problem is when I open the hedging order the existing order gets closed, why is that? Here is my order opening code.
request.action=TRADE_ACTION_CLOSE_BY; // type of trade operation request.position=position_ticket; // ticket of the position request.position_by=PositionGetInteger(POSITION_TICKET); // ticket of the opposite position
Please fill completely MqlTradeRequest before sending it. Double check your inputs. For instance:
request.price = SymbolInfoDouble(request.symbol,SYMBOL_BID);
is safer. Also, use the following BEFORE filling a trade request:
ZeroMemory(request);
And zeroing MqlTradeResult is also a nice idea just before OrderSend():
ZeroMemory(result);
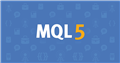
- www.mql5.com
I'm using MT5 hedging account and I'm trying to open an opposite market order (hedging order). I'm using MT5 hedging platform. The problem is when I open the hedging order the existing order gets closed, why is that? Here is my order opening code.
You think you're on a hedging account, but you're actually on a netted one. Open a new account that is a hedging account. If you're still in double then run this script...
//+------------------------------------------------------------------+ //| what_kind_of_account_am_i.mq5 | //| nicholishen | //| https://www.webs.com | //+------------------------------------------------------------------+ #property copyright "nicholishen" #property link "https://www.webs.com" #property version "1.00" //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ #include <trade/accountinfo.mqh> void OnStart() { CAccountInfo info; Print(info.MarginModeDescription()); }
You think you're on a hedging account, but you're actually on a netted one. Open a new account that is a hedging account. If you're still in double then run this script...
it says that on the platform itself see snapshot below.
it says that on the platform itself see snapshot below.
Then something somewhere is messed up in your code, which is why you should be working with the stdlib classes instead of trying to roll your own procedures using the low-level API. Run this script and step through it in the debugger. Compare it to your code and then see where yours went wrong.
#include <trade/trade.mqh> #include <trade/accountinfo.mqh> void OnStart() { CAccountInfo account; if (account.TradeMode() != ACCOUNT_TRADE_MODE_DEMO) return; Alert(account.MarginModeDescription()); CTrade trade; trade.Sell(0.1); trade.Buy(0.1); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm using MT5 hedging account and I'm trying to open an opposite market order (hedging order). I'm using MT5 hedging platform. The problem is when I open the hedging order the existing order gets closed, why is that? Here is my order opening code.