Ziklag: How should I modify the "Moving Average Expert Advisor" so as to
(1) Signal (buy/sell) INTRA-BAR other that at close
(2) Signal only ONCE PER BAR
(3) BUY ONLY and/ or SELL ONLY but not both
-
When you post code please use the CODE button (Alt-S)!
(For large amounts of code, attach it.) Please edit your post.
General rules and best pratices of the Forum. - General - MQL5 programming forum
Messages Editor - Don't SHOUT at us, that is RUDE.
- Don't add new bar test.
if(rt[1].tick_volume>1)
Add a new bar test.For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart,) volume is unreliable (miss ticks,) Price is unreliable (duplicate prices and The == operand. - MQL4 and MetaTrader 4 - MQL4 programming forum.) Always use time.
I disagree with making a new bar function, because it can only be called once per tick. A variable can be tested multiple times.
New candle - MQL4 and MetaTrader 4 - MQL4 programming forum (adjust for MT5.)- Vague. A) never: comment out the other or add an input and test it. B) No hedging: Test if you have the opposite already open.
Hi My name is Alangi Lawrence and i'm new to mql5 and i have had sleepless nights trying to work on this my mql5 code.
For over a week now i have faced serious difficulties in making my code activate an order on my mt5 trader. Everything is okay. All indicators are functional but conditions hold and no order is placed according to the conditions... Please i did really love if someone can solve this pain of mine. Thank you all.
Here is the code
//+------------------------------------------------------------------+ //| Eternal Realm ER 1.0.mq5 | //| Copyright 2023, E.R 1.0 | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2023, E.R" #property link "https://www.mql5.com" #property version "1.00" enum STRATEGY_IN { ONLY_MA, // Only moving averages ONLY_RSI, // Only RSI MA_AND_RSI, // Moving averages plus RSI }; sinput string s0; //---------Strategy----------- input STRATEGY_IN strategy = ONLY_MA; //Trader Entry Strategy sinput string s1; //---------Moving Averages--------- input int ma_slow_period = 21; // Slow Moving Average Period input int ma_fast_period = 50; // Fast Moving Average Period input ENUM_TIMEFRAMES ma_time_graphic = PERIOD_CURRENT;// Graphic Time input ENUM_MA_METHOD ma_method = MODE_EMA; // Method input ENUM_APPLIED_PRICE ma_price = PRICE_CLOSE; // Price Applied sinput string s2; //---------RSI---------------- input int rsi_period = 5; // RSI Period input ENUM_TIMEFRAMES rsi_time_graphic = PERIOD_CURRENT; //Graphic Time input ENUM_APPLIED_PRICE rsi_price = PRICE_CLOSE; // Price Applied input int rsi_overbought = 70; //Level Overbought input int rsi_oversold = 30; //Level Oversold sinput string s3; //----------------------------- input int num_lots = 100; // Number Of Lots input double TP = 60; // Take Profits input double SL = 30; // Stop Loss sinput string s4; //----------------------------- input string limit_close_op ="17:40"; // Time Limit Close Position int ma_fast_Handled; // Handle Fast Moving Averages double ma_fast_Buffer[]; // Buffer Fast Moving Averages // SLOW - longer period int ma_slow_Handled; // Handle Slow Moving Averages double ma_slow_Buffer[]; // Buffer Slow Moving Averages //---RSI int rsi_Handled; // Handle for RSI double rsi_Buffer[]; // Buffer for RSI int OnInit() { ma_fast_Handled = iMA(_Symbol,ma_time_graphic,ma_fast_period,0,ma_method,ma_price); ma_slow_Handled = iMA(_Symbol,ma_time_graphic,ma_slow_period,0,ma_method,ma_price); rsi_Handled = iRSI(_Symbol,rsi_time_graphic,rsi_period,rsi_price); if(ma_fast_Handled<0 || ma_slow_Handled<0 || rsi_Handled<0) { Alert("Error trying to create Handles for indicator - error: ",GetLastError(),"!"); return(-1); } CopyRates(_Symbol,_Period,0,4,candle); ArraySetAsSeries(candle,true); // TO add the indicator to chart: ChartIndicatorAdd(0,0,ma_fast_Handled); ChartIndicatorAdd(0,0,ma_slow_Handled); ChartIndicatorAdd(0,1,rsi_Handled); return(INIT_SUCCEEDED); } void OnDeinit(const int reason) { //--- IndicatorRelease(ma_fast_Handled); IndicatorRelease(ma_slow_Handled); IndicatorRelease(rsi_Handled); } bool isNewBar() { //--- memorize the time of opening of the last bar in the static variable static datetime last_time=0; //--- current time datetime lastbar_time= (datetime) SeriesInfoInteger(Symbol(),Period(),SERIES_LASTBAR_DATE); //---if it is the first call of the function if(last_time==0) { //---set the time and exit last_time=lastbar_time; return (false); } //--- if the time differs if(last_time!=lastbar_time) { //---memorize the time and return true last_time=lastbar_time; return (true); } //--- if we passed to this line then the bar is not new; return false return (false); } void drawVerticalLine(string name, datetime dt, color cor = clrAliceBlue) { ObjectDelete(0,name); ObjectCreate(0,name,OBJ_VLINE,0,dt,0); ObjectSetInteger(0,name,OBJPROP_COLOR,cor); } int Magic_number = 123456; // Magic number MqlTick tick; // variables for strong tick //BUY TO MARKET void BuyAtMarket() { MqlTradeRequest request; // request MqlTradeResult response; // response ZeroMemory(request); ZeroMemory(response); //--- for buy order request.action = TRADE_ACTION_DEAL; // Trade Operation type request.magic = Magic_number; // Magic Number request.symbol = _Symbol; // Trade Symbol request.volume = num_lots; // Lots Number request.price = NormalizeDouble(tick.ask,_Digits); // Price to sell request.sl = NormalizeDouble(tick.ask - SL*_Point,_Digits); // Stop Lose Price request.tp = NormalizeDouble(tick.ask + TP*_Point,_Digits); // Take Profit request.deviation = 0; // Maximal possible deviation request.type = ORDER_TYPE_BUY; // Order Type request.type_filling = ORDER_FILLING_FOK; // Order Execution type //--- bool success = OrderSend(request,response); //--- if(response.retcode == 10008 || response.retcode == 10009) { Print("Order to Sell executed successfully!!"); } else { Print("Error sending order to sell. Error = ",GetLastError()); ResetLastError(); } } //SELL TO MARKET void SellAtMarket() { MqlTradeRequest request; // request MqlTradeResult response; // response ZeroMemory(request); ZeroMemory(response); //--- for sell order request.action = TRADE_ACTION_DEAL; // Trade Operation type request.magic = Magic_number; // Magic Number request.symbol = _Symbol; // Trade Symbol request.volume = num_lots; // Lots Number request.price = NormalizeDouble(tick.bid,_Digits); // Price to sell request.sl = NormalizeDouble(tick.bid + SL*_Point,_Digits); // Stop Lose Price request.tp = NormalizeDouble(tick.bid - TP*_Point,_Digits); // Take Profit request.deviation = 0; // Maximal possible deviation request.type = ORDER_TYPE_SELL; // Order Type request.type_filling = ORDER_FILLING_FOK; // Order Execution type //--- bool success = OrderSend(request,response); //--- if(response.retcode == 10008 || response.retcode == 10009) { Print("Order to Sell executed successfully!"); } else { Print("Error sending order to sell. Error =",GetLastError()); ResetLastError(); } } void CloseBuy() { MqlTradeRequest request; // request MqlTradeResult response; // response ZeroMemory(request); ZeroMemory(response); //--- For Sell Order request.action = TRADE_ACTION_DEAL; request.magic = Magic_number; request.symbol = _Symbol; request.volume = num_lots; request.price = 0; request.type = ORDER_TYPE_SELL; request.type_filling = ORDER_FILLING_FOK; //--- bool success = OrderSend(request,response); //--- if(response.retcode == 10008 || response.retcode == 10009) { Print("Orders Buy executed successfully!"); } else { Print("Error sending order to Sell. Error =", GetLastError()); ResetLastError(); } } void CloseSell() { MqlTradeRequest request; // request MqlTradeResult response; // response ZeroMemory(request); ZeroMemory(response); //--- For Buy Order request.action = TRADE_ACTION_DEAL; request.magic = Magic_number; request.symbol = _Symbol; request.volume = num_lots; request.price = 0; request.type = ORDER_TYPE_BUY; request.type_filling = ORDER_FILLING_RETURN; //--- bool success = OrderSend(request,response); //--- if(response.retcode == 10008 || response.retcode == 10009) { Print("Orders Buy executed successfully!!"); } else { Print("Error sending order to Buy. Error =", GetLastError()); ResetLastError(); } } MqlRates candle[]; void OnTick() { // Copy a three-dimensional vector to Buffer CopyBuffer(ma_fast_Handled,0,0,4,ma_fast_Buffer); CopyBuffer(ma_slow_Handled,0,0,4,ma_slow_Buffer); CopyBuffer(rsi_Handled,0,0,4,rsi_Buffer); //--- Feed candles Buffers with data: CopyRates(_Symbol,_Period,0,4,candle); ArraySetAsSeries(candle,true); // Sort the data vector: ArraySetAsSeries(ma_fast_Buffer,true); ArraySetAsSeries(ma_slow_Buffer,true); ArraySetAsSeries(rsi_Buffer,true); //--- //Feed with tick variable data SymbolInfoTick(_Symbol,tick); string signal=""; double Ask=SymbolInfoDouble(_Symbol,SYMBOL_ASK); double Bid=SymbolInfoDouble(_Symbol,SYMBOL_BID); // LOGIC TO ACTIVATE Buy bool buy_ma_cros = ma_fast_Buffer[0] > ma_slow_Buffer[0] && ma_fast_Buffer[2] < ma_slow_Buffer[2]; bool buy_rsi = rsi_Buffer[0] <=rsi_overbought; // LOGIC TO ACTIVATE SALE bool sell_ma_cros = ma_slow_Buffer[0] > ma_fast_Buffer[0] && ma_slow_Buffer[2] < ma_fast_Buffer[2]; bool sell_rsi = rsi_Buffer[0] >=rsi_overbought; bool Buy = false; // Can Buy? bool Sell = false; // Can Sell? if(strategy == ONLY_MA) { Buy = buy_ma_cros; Sell = sell_ma_cros; } else if(strategy == ONLY_RSI) { Buy = buy_rsi; Sell = sell_rsi; } else { Buy = buy_ma_cros && buy_rsi; Sell = sell_ma_cros && sell_rsi; } bool newBar = isNewBar(); // Every time there is a new candle enter this 'if' if(newBar) { // Buy condition: if(Buy && PositionSelect(_Symbol)==false) { drawVerticalLine("Buy",candle[1].time,clrBlue); BuyAtMarket(); } // Sell condition: if(Sell && PositionSelect(_Symbol)==false) { drawVerticalLine("Sell",candle[1].time,clrRed); SellAtMarket(); } } if(newBar && TimeToString(TimeCurrent(),TIME_MINUTES) == limit_close_op && PositionSelect(_Symbol)==true) { Print("=====> End of Operating Time: End of Open Position!"); drawVerticalLine("Limit_OP",candle[0].time,clrYellow); if(PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY) { CloseBuy(); } if(PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL) { CloseSell(); } } }
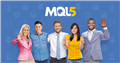
Discover new MetaTrader 5 opportunities with MQL5 community and services
- 2023.05.26
- www.mql5.com
MQL5: language of trade strategies built-in the MetaTrader 5 Trading Platform, allows writing your own trading robots, technical indicators, scripts and libraries of functions

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
HELLO, Am new to MetaTrader;
How should I modify the "Moving Average Expert Advisor" so as to
(1) Signal (buy/sell) INTRA-BAR other that at close
(2) Signal only ONCE PER BAR
(3) BUY ONLY and/ or SELL ONLY but not both
Thanks