Can't seem to find the original mt4 Fibonacci source code anywhere in codebase or any article.
I want to play around with the source code and amend to my own use.
Does anyone know where I can find this code (original mt4 application, not auto).
Or is this not allowed?
what you mean by "not auto"?
however, hope the following will answer your question
//+------------------------------------------------------------------+ //| Fibo.mq4 | //| Copyright 2017, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2016, Soubra2003" #property link "https://www.mql5.com/en/users/soubra2003/seller" #property version "1.00" #property strict #property indicator_chart_window input ENUM_TIMEFRAMES TF = PERIOD_CURRENT; //Time frame input color InpColor = clrRed; //Line color input ENUM_LINE_STYLE InpStyle = STYLE_DASH; //Line style input int InpWidth = 1; //Line width //--- /*input*/ string InpName="Fibo"; //Line name /*extern*/ double InpPrice=0.01; //Line price /*input*/ bool InpBack=false; //Background line /*input*/ bool InpSelection=true; //Highlight to move /*input*/ bool InpHidden=true; //Hidden in the object list /*input*/ long InpZOrder=0; //Priority for mouse click //--- double OPEN, HIGH, LOW, CLOSE, candlesize; //+------------------------------------------------------------------+ //| Expert initialization function //+------------------------------------------------------------------+ int OnInit() { for(int i=0;i<6;i++) { HLineCreate(0,InpName+(string)i,0,InpPrice,InpColor,InpStyle,InpWidth,InpBack,InpSelection,InpHidden,InpZOrder); HLineMove(0,InpName+(string)i,InpPrice); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function //+------------------------------------------------------------------+ void OnDeinit(const int reason) { for(int i=0;i<6;i++) ObjectDelete(ChartID(),InpName+(string)i); //--- Print("Bye."); } //+------------------------------------------------------------------+ //| Custom indicator iteration function //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- OPEN = iOpen(NULL,TF,1); HIGH = iHigh(NULL,TF,1); LOW = iLow(NULL,TF,1); CLOSE = iClose(NULL,TF,1); // candlesize = HIGH-LOW; if(candlesize < 0) candlesize=candlesize*(-1); candlesize = NormalizeDouble(candlesize,Digits); //--- HLineMove(0,InpName+(string)0,LOW); HLineMove(0,InpName+(string)5,HIGH); // if(CLOSE > OPEN) //UP { HLineMove(0,InpName+(string)1,HIGH-candlesize*0.236); HLineMove(0,InpName+(string)2,HIGH-candlesize*0.382); HLineMove(0,InpName+(string)3,HIGH-candlesize*0.50); HLineMove(0,InpName+(string)4,HIGH-candlesize*0.618); } else if(CLOSE < OPEN) //DOWN { HLineMove(0,InpName+(string)1,LOW+candlesize*0.236); HLineMove(0,InpName+(string)2,LOW+candlesize*0.382); HLineMove(0,InpName+(string)3,LOW+candlesize*0.50); HLineMove(0,InpName+(string)4,LOW+candlesize*0.618); } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| Create the horizontal line | //+------------------------------------------------------------------+ bool HLineCreate(const long chart_ID=0, // chart's ID const string name="HLine", // line name const int sub_window=0, // subwindow index double price=0, // line price const color clr=clrRed, // line color const ENUM_LINE_STYLE style=STYLE_SOLID, // line style const int width=1, // line width const bool back=false, // in the background const bool selection=true, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- if the price is not set, set it at the current Bid price level if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- create a horizontal line if(!ObjectCreate(chart_ID,name,OBJ_HLINE,sub_window,0,price)) { Print(__FUNCTION__, ": failed to create a horizontal line! Error code = ",GetLastError()); return(false); } //--- set line color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set line display style ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style); //--- set line width ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,width); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the line by mouse //--- when creating a graphical object using ObjectCreate function, the object cannot be //--- highlighted and moved by default. Inside this method, selection parameter //--- is true by default making it possible to highlight and move the object ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Move horizontal line | //+------------------------------------------------------------------+ bool HLineMove(const long chart_ID=0, // chart's ID const string name="HLine", // line name double price=0) // line price { //--- if the line price is not set, move it to the current Bid price level if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- move a horizontal line if(!ObjectMove(chart_ID,name,0,0,price)) { Print(__FUNCTION__, ": failed to move the horizontal line! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //Bye
Now, you are lucky by finding someone to reply with source code.
Next time you have to open a new job in the freelance service to hire a developer.
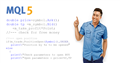
- www.mql5.com
Now, you are lucky by finding someone to reply with source code.
Next time you have to open a new job in the freelance service to hire a developer.
Most source codes for MT4 are available in CodeBase or through general articles eg MACD
codes, Moving Average Codes etc. I was only asking the question of why I could not find a source for Fibonacci indicator.
Most source codes for MT4 are available in CodeBase or through general articles eg MACD
codes, Moving Average Codes etc. I was only asking the question of why I could not find a source for Fibonacci indicator.
I do thank you for your kind response and do appreciate it!!
I do thank you for your kind response and do appreciate it!!
any time
welcome
Can't seem to find the original mt4 Fibonacci source code anywhere in codebase or any article.
There is no original Fibonacci source code.
There may be various indicators that use Fibonacci.
Fibonacci Expansion etc is an object, not an indicator.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Can't seem to find the original mt4 Fibonacci source code anywhere in codebase or any article.
I want to play around with the source code and amend to my own use.
Does anyone know where I can find this code (original mt4 application, not auto).
Or is this not allowed?