You can't "extend" a structure, especially not one used internally by the built-in functions. You can however define your own "similar" structures for your own functionality.
You can't "extend" a structure, especially not one used internally by the built-in functions. You can however define your own "similar" structures for your own functionality.
Yes you can inherit from a structure.
struct CustomTick:MqlTick { string symbol; int account; };
However with MqlTick, you will not be able to use it in function like SymbolInfoTick().
- Sure you can, just like you can pass a subclass object to a function taking the superclass.
Right it works for SymbolInfoTick().
But it doesn't with MqlRates and CopyRates() (compiles but gives runtime error 4029: Invalid Array). So I would suggest anyone to check before relying on such solution for internal mql structure.
I am using this code to save and load tick to file memory. It expects MqlTick and throws parameter conversion error with the custom struct. Any solution?
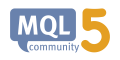
- www.mql5.com
...
Forum on trading, automated trading systems and testing trading strategies
Hello,
Please EDIT your post and use the SRC button when you post code.
Thank you.
You just do it. Structs can only extend structs and classes only classes.
- Sure you can, just like you can pass a subclass object to a function taking the superclass.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello,
I need to extend MqlTick struct to include additional info such as symbol and AccountPlatform.
How can it be done?