commission which I don't yet know where to get from.
Форум по трейдингу, автоматическим торговым системам и тестированию торговых стратегий
Вопросы от начинающих MQL5 MT5 MetaTrader 5
fxsaber, 2016.10.29 17:09
{
double Commission = ::PositionGetDouble(POSITION_COMMISSION);
// На случай, если POSITION_COMMISSION не работает
if (Commission == 0)
{
const ulong Ticket = GetPositionDealIn();
if (Ticket > 0)
{
const double LotsIn = ::HistoryDealGetDouble(Ticket, DEAL_VOLUME);
if (LotsIn > 0)
Commission = ::HistoryDealGetDouble(Ticket, DEAL_COMMISSION) * ::PositionGetDouble(POSITION_VOLUME) / LotsIn;
}
}
return(Commission);
}
Форум по трейдингу, автоматическим торговым системам и тестированию торговых стратегий
Как узнать размер комиссии не открывая позицию по инструменту?
fxsaber, 2016.11.08 20:30
{
ulong Ticket = 0;
if ((PositionIdentifier == 0) ? ::HistorySelectByPosition(::PositionGetInteger(POSITION_IDENTIFIER)) : ::HistorySelectByPosition(PositionIdentifier))
{
const int Total = ::HistoryDealsTotal();
for (int i = 0; i < Total; i++)
{
const ulong TicketDeal = ::HistoryDealGetTicket(i);
if (TicketDeal > 0)
if ((ENUM_DEAL_ENTRY)::HistoryDealGetInteger(TicketDeal, DEAL_ENTRY) == DEAL_ENTRY_IN)
{
Ticket = TicketDeal;
break;
}
}
}
return(Ticket);
}
thanks fxsaber, but this way you are getting commision from previous deals which were closed. What if I don't have them yet, I'm trading multiple instruments with this EA, so there may be no done deals yet at the time I want to close my first one.
Besides, the question about how get "net profit" remains still open. Any other ideas, inputs, clarifications, much welcome.
Thanks in advance
thanks fxsaber, but this way you are getting commision from previous deals which were closed. What if I don't have them yet, I'm trading multiple instruments with this EA, so there may be no done deals yet at the time I want to close my first one.
First try!
Thanks I will, and will report back. In the meantime do you know what is included/excluded in
PositionGetDouble(POSITION_PROFIT);
Much thanks again for your help.
Thanks I will, and will report back. In the meantime do you know what is included/excluded in
PositionGetDouble(POSITION_PROFIT);
Much thanks again for your help.
Ok fxsaber was right, I can find deals from my current position, once I have the first deal, I can get it's commision and calculate commision on my position that way, cool.
I still haven't managed to find out about PositionGetDouble(POSITION_PROFIT), what it actually contans, I'll do some sample trades with my EA to clear this.
Thanks again.
POSITION_PROFIT = POSITION_VOLUME * SYMBOL_TRADE_TICK_VALUE * (POSITION_PRICE_CURRENT - POSITION_PRICE_OPEN) / SYMBOL_TRADE_TICK_SIZE
ACCOUNT_PROFIT = ACCOUNT_EQUITY - ACCOUNT_BALANCE = Sum(POSITION_PROFIT[i] + POSITION_SWAP[i] + POSITION_COMMISSION[i])
POSITION_PROFIT = POSITION_VOLUME * SYMBOL_TRADE_TICK_VALUE * (POSITION_PRICE_CURRENT - POSITION_PRICE_OPEN) / SYMBOL_TRADE_TICK_SIZE
ACCOUNT_PROFIT = ACCOUNT_EQUITY - ACCOUNT_BALANCE = Sum(POSITION_PROFIT[i] + POSITION_SWAP[i] + POSITION_COMMISSION[i])
Thanks fxsaber, I haven't checked that yet, but from the docs, I see that
SYMBOL_TRADE_TICK_VALUE=Value of SYMBOL_TRADE_TICK_VALUE_PROFIT
SYMBOL_TRADE_TICK_VALUE_PROFIT=Calculated tick price for a profitable position
What is now the a profitable position? Ok in other words, to make long story short, I can reduce question to this:
When I'm calculating net profit for a position which is still open, I use PositionGetDouble(POSITION_PROFIT), but I have to subtract Commision and Swaps? Is this right? Ok, Swaps can also be positive, but just I need just the principle.
Thanks again.
POSITION_PROFIT = POSITION_VOLUME * SYMBOL_TRADE_TICK_VALUE * (POSITION_PRICE_CURRENT - POSITION_PRICE_OPEN) / SYMBOL_TRADE_TICK_SIZE
ACCOUNT_PROFIT = ACCOUNT_EQUITY - ACCOUNT_BALANCE = Sum(POSITION_PROFIT[i] + POSITION_SWAP[i] + POSITION_COMMISSION[i])
I have 40 open positions now.
10 positions has same magic number.
I want calculate sum profit of these 10 positions; if was more than 70$, then i want close all 10 positions.
It was simple in MT4
double POSITIONS_TOTAL_PROFIT() { double Total_Profit=0; int total=OrdersTotal(); for(int pos=0; pos<total; pos++) { if(OrderSelect(pos,SELECT_BY_POS)==true && OrderMagicNumber()==magic) { if(OrderType()==OP_BUY || OrderType()==OP_SELL) Total_Profit+=OrderProfit()+OrderSwap()+OrderCommission(); } } return(Total_Profit); }
But in MT5, it's complicating.
I tried this:
Total_Profit = POSITION_PROFIT+ POSITION_COMMISSION + POSITION_SWAP+ POSITION_FEE
I can't get POSITION_COMMISSION and POSITION_FEE
I know I should use "DEAL_COMMISSION" and "DEAL_FEE", but i don't know how.
I can't find any relationship between DEAL and own POSITION
note: these positions are open (no in history)
I'm thankful of your answers.
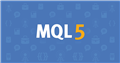
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi there, the title says it all..In my EA (MT5) when the robot is closing position I want to check the profit of current position properly. I know there is a function "PositionGetDouble" but am not sure about how to use it properly. On my ECN account I pay commisions for each position opening cca. $2/lot + each position closing, totaling $4/lot. Besides that there may be positive or negative swap calculated.
So my question is how can I calculate my net profit for a certain position?
double x = PositionGetDouble(POSITION_PROFIT);
double y = PositionGetDouble(POSITION_SWAP);
Is variable "x" my net profit, or should I subtract variable "y" and commission which I don't yet know where to get from.
Please suggest, much thanks in advance for your help.