Try with a magic wand.
Hi Alain. Good to see you still here.
Seriously, nobody can help you without seeing the code.
Seriously, nobody can help you without seeing the code.
Almost forgot the most important part
//| CS Scanner.mq4 |
//| doshur |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "doshur"
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
#property show_inputs
extern bool ShowChart = false;
//+------------------------------------------------------------------+
//| Script program start function |
//+------------------------------------------------------------------+
void OnStart()
{
//---
string FX_Pairs[] = {"AUDCAD",
"AUDCHF",
"AUDNZD",
"AUDJPY",
"AUDUSD",
"CADCHF",
"CADJPY",
"CHFJPY",
"EURAUD",
"EURCAD",
"EURCHF",
"EURGBP",
"EURNZD",
"EURJPY",
"EURUSD",
"GBPAUD",
"GBPCAD",
"GBPCHF",
"GBPNZD",
"GBPJPY",
"GBPUSD",
"USDCAD",
"USDCHF",
"USDJPY",
"NZDCAD",
"NZDCHF",
"NZDJPY",
"NZDUSD",
};
double Curr_H, Curr_L, Curr_C, Curr_O,
Prev_H, Prev_L, Prev_C, Prev_O,
Past_H, Past_L, Past_C, Past_O,
Curr_HL, Curr_UT, Curr_LT, Curr_BD,
Prev_HL,
Past_HL, Past_BD,
Curr_AR;
bool TradeSig;
long Chart;
for(int i = 0; i < ArraySize(FX_Pairs); i++)
{
Curr_L = iLow(FX_Pairs[i], PERIOD_CURRENT, 1);
Curr_H = iHigh(FX_Pairs[i], PERIOD_CURRENT, 1);
Curr_O = iOpen(FX_Pairs[i], PERIOD_CURRENT, 1);
Curr_C = iClose(FX_Pairs[i], PERIOD_CURRENT, 1);
Prev_L = iLow(FX_Pairs[i], PERIOD_CURRENT, 2);
Prev_H = iHigh(FX_Pairs[i], PERIOD_CURRENT, 2);
Prev_O = iOpen(FX_Pairs[i], PERIOD_CURRENT, 2);
Prev_C = iClose(FX_Pairs[i], PERIOD_CURRENT, 2);
Past_L = iLow(FX_Pairs[i], PERIOD_CURRENT, 3);
Past_H = iHigh(FX_Pairs[i], PERIOD_CURRENT, 3);
Past_O = iOpen(FX_Pairs[i], PERIOD_CURRENT, 3);
Past_C = iClose(FX_Pairs[i], PERIOD_CURRENT, 3);
Curr_HL = Curr_H - Curr_L;
Curr_AR = iATR(FX_Pairs[i], PERIOD_CURRENT, 20, 1);
Curr_BD = MathMax(Curr_C, Curr_O) - MathMin(Curr_C, Curr_O);
Past_BD = MathMax(Past_C, Past_O) - MathMin(Past_C, Past_O);
Prev_HL = Prev_H - Prev_L;
Past_HL = Past_H - Past_L;
TradeSig = false;
//---- INSIDE BAR
if(Curr_HL < Curr_AR)
{
if(Prev_H > Curr_H && Prev_L < Curr_L)
{
Print("Inside Bar - ", FX_Pairs[i]);
TradeSig = true;
}
}
if(ShowChart && TradeSig)
{
Chart = ChartOpen(FX_Pairs[i], PERIOD_D1);
ChartApplyTemplate(Chart, "kiss.tpl");
}
}
//---
}
//+------------------------------------------------------------------+
I would recommend to you to use
MqlRates rates[];
//ArraySetAsSeries(rates,true);
enum IdxCR { i4,i3,i2,i1,i0 }; // only valid in this case as i4==0 and with this you don't need ArraySetAsSeries
...
int copied=CopyRates(FX_Pairs[i],0,0,4,rates);
if(copied>0) {
...
Prev_H = rates[i2].high;
...
}
I think that
- CopyRates(..) will give you the latest quotes - without an additional RefreshRates
- it is faster - I think copy only one array instead of 4*3 different function calls with data requests
- it is mql5-ready
- enum-values can be handled by the debugger #define not :(
I would recommend to you to use
MqlRates rates[];
//ArraySetAsSeries(rates,true);
enum IdxCR { i4,i3,i2,i1,i0 }; // only valid in this case as i4==0 and with this you don't need ArraySetAsSeries
...
int copied=CopyRates(FX_Pairs[i],0,0,4,rates);
if(copied>0) {
...
Prev_H = rates[i2].high;
...
}
I think that
- CopyRates(..) will give you the latest quotes - without an additional RefreshRates
- it is faster - I think copy only one array instead of 4*3 different function calls with data requests
- it is mql5-ready
- enum-values can be handled by the debugger #define not :(
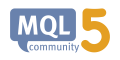
- www.mql5.com
Hi all,
I have tried copyrates but first run still do not have the latest data. Only when I run it the second time it gives me the correct output.
Any idea?
Try make loop to get all data, then scan all.
I guess so. Have to make to loops instead of one.
Thanks for the suggestion.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I have a script to scan candlestick pattern on multiple symbols. When I run the script in the morning, it always gives me candlestick pattern the day before.
After I run it again, it gives me today's pattern. Seems that the first run did not download the symbols price data.
How do I force download all symbols data in script?
Thanks