- Wir können hier deutsch reden :)
- Hast Du schon versucht mit dem Debugger herauszufinden wo, wie und warum die Koordinaten der Button veränder,kopiert bzw, verschoben werden?
Hier ist mehr dazu:
https://www.metatrader5.com/de/metaeditor/help/development/debug
https://www.mql5.com/de/articles/2041 // Error Handling and Logging in MQL5
https://www.mql5.com/de/articles/272
https://www.mql5.com/de/articles/35 // scrol down to: "Launching and Debuggin"
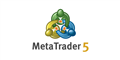
- www.metatrader5.com
Danke für die schnelle Antwort!
Habe mal ein Video aufgeommen um das Problem zu verdeutlichen.
Scheint so als würden die Koordinaten addiert werden. Je öfter man den Sub-tab entfernt und wieder hinzufügt, desto schneller bewegt er sich ;D
Werde mal versuchen mit dem Debugger nach dem Problem zu suchen, für mich sieht es aber so aus, als ob der Button beim Entfernen nicht vollständig aus dem Container des Panels entfernt wird bzw. die Koordinaten noch irgendwo gespeichert bleiben.
Schönen Sonntag noch!
Danke für die schnelle Antwort!
Habe mal ein Video aufgeommen um das Problem zu verdeutlichen.
Scheint so als würden die Koordinaten addiert werden. Je öfter man den Sub-tab entfernt und wieder hinzufügt, desto schneller bewegt er sich ;D
Werde mal versuchen mit dem Debugger nach dem Problem zu suchen, für mich sieht es aber so aus, als ob der Button beim Entfernen nicht vollständig aus dem Container des Panels entfernt wird bzw. die Koordinaten noch irgendwo gespeichert bleiben.
Schönen Sonntag noch!
Das: https://www.mql5.com/de/articles/9612 ist der bislang letzte Artikel eine Reihe, die sich mit solchen Problemen beschäftigen!
Ich denke, da kannst Du Dir viel kopieren und viel Zeit so ersparen.
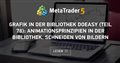
- www.mql5.com
Konnte das Problem beheben!
Der Button muss ein dynamisches Objekt sein damit dieser in ArrayObj gelöscht wird.
Folgendes habe ich am Code verändert:
#include <Controls\Dialog.mqh> #include <Controls\Button.mqh> class MyPanel : public CAppDialog { private: //--- variables CButton m_tabBtn1; CButton m_tabBtn2; CButton *m_tabSubBtn; //--- private methods void OnClickTab1(); void OnClickTab2(); void CreateTabButtons(); void CreateSubTabButton(); public: //--- constructor, initialization method void MyPanel(); void ~MyPanel(); //--- chart event handler virtual bool Create(const long chart,const string name,const int subwin,const int x1,const int y1,const int x2,const int y2); virtual bool OnEvent(const int id,const long &lparam,const double &dparam,const string &sparam); }; EVENT_MAP_BEGIN(MyPanel) ON_EVENT(ON_CLICK,m_tabBtn1,OnClickTab1) ON_EVENT(ON_CLICK,m_tabBtn2,OnClickTab2) EVENT_MAP_END(CAppDialog) void MyPanel::MyPanel(){ } void MyPanel::~MyPanel(){ delete m_tabSubBtn; } //+------------------------------------------------------------------+ //| Creation | //+------------------------------------------------------------------+ bool MyPanel::Create(const long chart,const string name,const int subwin,const int x1,const int y1,const int x2,const int y2) { if(!CAppDialog::Create(chart,name,subwin,x1,y1,x2,y2)) return(false); CreateTabButtons(); this.Run(); return(true); } // Creates main tab buttons void MyPanel::CreateTabButtons() { m_tabBtn1.Create(NULL,"buttonTab1",NULL,0,20,300,100); m_tabBtn1.Text("Tab 1"); m_tabBtn1.ColorBackground(clrGray); Add(m_tabBtn1); m_tabBtn2.Create(NULL,"buttonTab2",NULL,300,20,600,100); m_tabBtn2.Text("Tab 2"); m_tabBtn2.ColorBackground(clrWhite); Add(m_tabBtn2); } // Creates new sub tab void MyPanel::CreateSubTabButton() { m_tabSubBtn = new CButton(); m_tabSubBtn.Create(NULL,"tabSubBtn",NULL,0,100,200,150); m_tabSubBtn.Text("Tab 2 sub 1"); m_tabSubBtn.ColorBackground(clrGray); Add(m_tabSubBtn); } void MyPanel::OnClickTab1() { // set background color m_tabBtn1.ColorBackground(clrGray); m_tabBtn2.ColorBackground(clrWhite); // Delete sub tab button delete m_tabSubBtn; Print("tab 1 clicked"); } void MyPanel::OnClickTab2() { // set background color m_tabBtn1.ColorBackground(clrWhite); m_tabBtn2.ColorBackground(clrGray); // Create sub tab CreateSubTabButton(); Print("tab 2 clicked"); } MyPanel MyP(); int OnInit() { MyP.Create(NULL,"test",NULL,0,0,700,300); MyP.Run(); return(INIT_SUCCEEDED); } void OnDeinit(const int reason) { MyP.Destroy(); } void OnChartEvent(const int id, const long& lparam, const double& dparam, const string& sparam) { MyP.ChartEvent(id,lparam,dparam,sparam); }
Auf die Lösung bin ich durch die folgenden Forumeinträge gekommen:
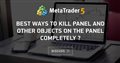
- 2015.11.02
- www.mql5.com

- Freie Handelsapplikationen
- Über 8.000 Signale zum Kopieren
- Wirtschaftsnachrichten für die Lage an den Finanzmärkte
Sie stimmen der Website-Richtlinie und den Nutzungsbedingungen zu.
Hi! When you add a button to a panel, it moves with the panel. If you delete it and add it again, it will still move with the panel, but twice as fast. I created the following simple panel to illustrate the problem.
If you click on tab 2, a sub-tab appears. When moving, it moves correctly with the panel. If you click on tab number 1, the sub-tab will be deleted. If you click on tab 2 again, the sub-tab is created again. Now it moves incorrectly when moving the panel.
Any idea to solve that problem ?
Thanks!