You need to limit the lot size to me always >= Min lot that broker allow and <= Max lot.
Also, always normalize lot size to lot digits as following ...
double maxlot = MarketInfo(Symbol(),MODE_MAXLOT); double minlot = MarketInfo(Symbol(),MODE_MINLOT); if(minlot==0.001) LotDigits=3; if(minlot==0.01) LotDigits=2; if(minlot==0.1) LotDigits=1; if(minlot==1) LotDigits=0; if (Lot>=maxlot) Lot = maxlot; if (Lot<=minlot) Lot = minlot; if(!UseMM) Lot = Lots; return(NormalizeDouble(Lot,LotDigits));
The above is not a ready code to use, but as you are a coder you will understand what I mean :)
Hi, everyone,
I am developing expert advisor.
But there is an "invalid volume" problem in my coding.
Below is the method I learn and think at mql5 articles.
I am going to put above code into "on Init" function of my expert advisor.
Lot size of order at my expert advisor is 0.1 and volume is 0.01.
Is it right?
Please help me.
Thanks to everyone.
Your code just do the check volume (Lots Size), but did not make the correction volume size, if CheckVolumeValue() return false.
You should add the code:
//---------- For example --------// if(!CheckVolumeValue(vol,descript)) { if(vol<SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MIN)) vol=NormalizeDouble(SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MIN),2); if(vol>SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MAX)) vol=NormalizeDouble(SymbolInfoDouble(Symbol(),SYMBOL_VOLUME_MAX),2); } //------------------------
You need to limit the lot size to me always >= Min lot that broker allow and <= Max lot.
Also, always normalize lot size to lot digits as following ...
The above is not a ready code to use, but as you are a coder you will understand what I mean :)
Thanks very much.
I am editing my expert advisor in accordance with your valuable advice.
Your code just do the check volume (Lots Size), but did not make the correction volume size, if CheckVolumeValue() return false.
You should add the code:
You need to limit the lot size to me always >= Min lot that broker allow and <= Max lot.
Also, always normalize lot size to lot digits as following ...
The above is not a ready code to use, but as you are a coder you will understand what I mean :)
Thanks to your valuable advice.
I am editing my expert advisor in accordance with your advice.
Then I have another guestion.
How can I confirm on whether or not there are or no "invalid volume"error?
Below is the journal at strategy tester visualization.
And This is MT5 platform journal at random delay, every tick based real ticks, $1 deposit and leverage 100:1.
But I can't find "invalid volume".
How can I confirm "invalid volume" error?
Please let me know.
Thanks very much.
God bless you!
Thanks to your valuable advice.
I am editing my expert advisor in accordance with your advice.
Then I have another guestion.
How can I confirm on whether or not there are or no "invalid volume"error?
Below is the journal at strategy tester visualization.
And This is MT5 platform journal at random delay, every tick based real ticks, $1 deposit and leverage 100:1.
But I can't find "invalid volume".
How can I confirm "invalid volume" error?
Please let me know.
Thanks very much.
God bless you!
Error on your EA is about problem ACCOUNT_FREEMARGIN and error There is not enough money to complete the request (TRADE_RETCODE_NO_MONEY = error 10019)
You should check the documentation MQL5 about ENUM_SYMBOL_CALC_MODE
https://www.mql5.com/en/docs/constants/environment_state/marketinfoconstants
https://www.mql5.com/en/docs/account/accountinfodouble
https://www.mql5.com/en/docs/constants/errorswarnings/enum_trade_return_codes
We can not help without seeing your EA code.
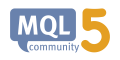
- www.mql5.com
Error on your EA is about problem ACCOUNT_FREEMARGIN and error There is not enough money to complete the request (TRADE_RETCODE_NO_MONEY = error 10019)
You should check the documentation MQL5 about ENUM_SYMBOL_CALC_MODE
https://www.mql5.com/en/docs/constants/environment_state/marketinfoconstants
https://www.mql5.com/en/docs/account/accountinfodouble
https://www.mql5.com/en/docs/constants/errorswarnings/enum_trade_return_codes
We can not help without seeing your EA code.
Your information is very useful to me.
I will study and review the documentation you mentioned.
Thanks very much.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi, everyone,
I am developing expert advisor.
But there is an "invalid volume" problem in my coding.
Below is the method I learn and think at mql5 articles.
I am going to put above code into "on Init" function of my expert advisor.
Lot size of order at my expert advisor is 0.1 and volume is 0.01.
Is it right?
Please help me.
Thanks to everyone.