How can I get my code to implement the symbol map from input while getting signals from telegram. I want the broker's suffixes to not be an issue.
This snippets tries to get the map ready from input during initialization.
Then here tries to replace texts for easy reading and processing of signal parameters.
The rest of the code is attached here.
Assuming that both you and the signal provider are trading forex or CFD's and you want to remove the suffixes of the provider:
StringSubstr("provider's symbol", 0, 6);
This turns EURUSD-FX into EURUSD.
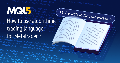
- www.mql5.com
Assuming that both you and the signal provider are trading forex or CFD's and you want to remove the suffixes of the provider:
This turns EURUSD-FX into EURUSD.
Yeah but consider a case where a signal provider issues a trade call on cable (gbpusd) or uses some other names on it. I need to make sure the provider's symbol name gets replaced by it's real name as indicated on input. E.g
How can I get my code to implement the symbol map from input while getting signals from telegram. I want the broker's suffixes to not be an issue.
This snippets tries to get the map ready from input during initialization.
Then here tries to replace texts for easy reading and processing of signal parameters.
The rest of the code is attached here.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
How can I get my code to implement the symbol map from input while getting signals from telegram. I want the broker's suffixes to not be an issue.
This snippets tries to get the map ready from input during initialization.
Then here tries to replace texts for easy reading and processing of signal parameters.
The rest of the code is attached here.