double rsiValue = iRSI(NULL, 0, RSI_Period, PRICE_CLOSE, i);
That is a MT4 call, not MT5.
Perhaps you should read the manual, especially the examples.
How To Ask Questions The Smart Way. (2004)
How To Interpret Answers.
RTFM and STFW: How To Tell You've Seriously Screwed Up.
They all (including iCustom) return a handle (an int). You get that in OnInit. In OnTick/OnCalculate/OnStart (after the indicator has updated its buffers), you use the handle, shift and count to get the data.
Technical Indicators - Reference on algorithmic/automated trading language for MetaTrader 5
Timeseries and Indicators Access / CopyBuffer - Reference on algorithmic/automated trading language for MetaTrader 5
How to start with MQL5 - General - MQL5 programming forum - Page 3 #22 (2020)
How to start with MQL5 - MetaTrader 5 - General - MQL5 programming forum - Page 7 #61 (2020)
MQL5 for Newbies: Guide to Using Technical Indicators in Expert Advisors - MQL5 Articles (2010)
How to call indicators in MQL5 - MQL5 Articles (2010)
Rushing too far ahead. In programming, you have to get one thing working at a time. Once you have a valid handle, and you are able to print one RSI value (let's say the latest value), only then should you continue.
You can't write a full script before checking that everything is working correctly from bottom to top. You have to learn how to read an API as well. No programmer can escape that.
Let's break down the important details from the programmers manual:
iRSI The function returns the handle of the Relative Strength Index indicator. It has only one buffer. int iRSI( string symbol, // symbol name ENUM_TIMEFRAMES period, // period int ma_period, // averaging period ENUM_APPLIED_PRICE applied_price // type of price or handle );
First important point:
It has only one buffer.
Next important detail:
int iRSI( Return Value Returns the handle of a specified technical indicator, in case of failure returns INVALID_HANDLE. The computer memory can be freed from an indicator that is no more utilized, using the IndicatorRelease() function, to which the indicator handle is passed. |
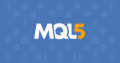
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Tried coding a script in mq5. But it is giving me 2 errors .
1. 'iRSI' - wrong parameters count SPIKE CATCHER.mq5 57 25
2. 'iRSI' - wrong parameters count SPIKE CATCHER.mq5 91 30
here is the code