You will have to elaborate a bit more on this, set to what? if you are referring to the expiration time, its in line 2. Im not sure what you want.
https://www.mql5.com/en/docs/constants/structures/mqltraderequest
datetime expiration=TimeTradeServer()+PeriodSeconds(PERIOD_M20);//Here you change the minutes trade.BuyStop(0.10,Ask+100*_Point,_Symbol,buySl,Ask+300*_Point,ORDER_TIME_SPECIFIED,expiration,0);
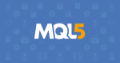
- www.mql5.com
You will have to elaborate a bit more on this, set to what? if you are referring to the expiration time, its in line 2. Im not sure what you want.
https://www.mql5.com/en/docs/constants/structures/mqltraderequest
Id like to make original buy stop function because I'd like to setting slippage
Hi,
you can try this:
datetime SetOrderExpirationTime(int hourExpiry) { MqlDateTime today; //-- TimeToStruct(iTime(_Symbol,PERIOD_M1,1),today); today.hour+=hourExpiry; //-- return(StructToTime(today)); }
for example, expiration time is 1 hour:
request.expiration = SetOrderExpirationTime(1);
Thank you for your help. I tried it but it doesnt work.
I have never got correct time on this code.
What do you mean 'correct time' ?
Here is a result :
//+------------------------------------------------------------------+ //| TimeExpiration.mq5 | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2024, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" //--- input parameters input int exp_hour=1; bool firstcall; //+------------------------------------------------------------------+ datetime SetOrderExpirationTime(int hourExpiry) { MqlDateTime today; //-- TimeToStruct(iTime(_Symbol,PERIOD_M1,1),today); today.hour+=hourExpiry; //-- return(StructToTime(today)); } //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- firstcall=true; //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- if(firstcall) { firstcall=false; Print("Time bar 1 (M1): ",TimeToString(iTime(_Symbol,PERIOD_M1,1))," + ",exp_hour," hour = ", TimeToString(SetOrderExpirationTime(exp_hour)) ); } } //+------------------------------------------------------------------+
No I mean if I use Buy Stop Function I couldn't return correct time.
request.expiration = SetOrderExpirationTime(1);
Probably that code is not correct.
SetOrderExpirationTime is returned correct time but if I use that code and if I order buy stop that is not returned expiration time.
Buy Stop Function is returned this time "1970.01.01 00:00:00".
No I mean if I use Buy Stop Function I couldn't return correct time.
Probably that code is not correct.
SetOrderExpirationTime is returned correct time but if I use that code and if I order buy stop that is not returned expiration time.
Buy Stop Function is returned this time "1970.01.01 00:00:00".
You may have forgotten this part:
https://www.mql5.com/en/docs/constants/tradingconstants/orderproperties#enum_order_type_time
struct MqlTradeRequest { ENUM_TRADE_REQUEST_ACTIONS action; // Trade operation type ulong magic; // Expert Advisor ID (magic number) ulong order; // Order ticket string symbol; // Trade symbol double volume; // Requested volume for a deal in lots double price; // Price double stoplimit; // StopLimit level of the order double sl; // Stop Loss level of the order double tp; // Take Profit level of the order ulong deviation; // Maximal possible deviation from the requested price ENUM_ORDER_TYPE type; // Order type ENUM_ORDER_TYPE_FILLING type_filling; // Order execution type ENUM_ORDER_TYPE_TIME type_time; // Order expiration type datetime expiration; // Order expiration time (for the orders of ORDER_TIME_SPECIFIED type) string comment; // Order comment ulong position; // Position ticket ulong position_by; // The ticket of an opposite position };
and you can also look for some examples at:
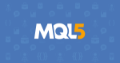
- www.mql5.com
You may have forgotten this part:
https://www.mql5.com/en/docs/constants/tradingconstants/orderproperties#enum_order_type_time
and you can also look for some examples at:
Thank you so much. It is working.
request.type_time = ORDER_TIME_SPECIFIED; request.expiration = SetOrderExpirationTime(1);
If you struggle and no one can give you some advice, you may always look at the MQL5 reference manual, you will always find a solution there, there are so many ways to do things. I am happy you found a solution

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use