Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
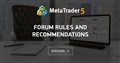
- 2023.03.12
- www.mql5.com
'TimeHour' - undeclared identifier Line 19 Column 28
'currentTime' - some operator expected Line 19 Column 37
'OP_BUY' - undeclared identifier Line 31 Column 46
'OrderSend' - wrong parameters count Line 31 Column 27
'OP_BUY' - undeclared identifier Line 50 Column 54
'OrderSend' - wrong parameters count Line 50 Column 35
Your code is MT4. You are compiling with MT5.
Your topic has been moved to the section: MQL4 and MetaTrader 4
Please consider which section is most appropriate — https://www.mql5.com/en/forum/172166/page6#comment_49114893
I'm trying to code for MT5. I need help with MT5. And my code is copyright free.
I have moved your topic into the MQL5 section: Expert Advisors and Automated Trading. However, please note that your code is not proper MQL5 and this has nothing to do with copyrights.
You probably generated using ChatGPT os some other generator, which mixes MQL4 and MQL5. If that is the case then we will not be able to help you because you seem not to realise the implications.
Errors:
'TimeHour' - undeclared identifier Line 19 Column 28
'currentTime' - some operator expected Line 19 Column 37
'OP_BUY' - undeclared identifier Line 31 Column 46
'OrderSend' - wrong parameters count Line 31 Column 27
'OP_BUY' - undeclared identifier Line 50 Column 54
'OrderSend' - wrong parameters count Line 50 Column 35
// Define input parameters input double stopLossPoints = 50; // Stop loss distance in points input double takeProfitPoints = 100; // Take profit distance in points input double martingaleMultiplier = 2.0; // Martingale multiplier input double initialLotSize = 0.01; // Initial lot size // Global variables bool tradeOpened = false; double entryPrice = 0.0; double stopLossPrice = 0.0; double takeProfitPrice = 0.0; double lotSize = initialLotSize; datetime lastTradeHour = 0; input long order_magic=55555; void OnInit() { if(AccountInfoInteger(ACCOUNT_TRADE_MODE)==ACCOUNT_TRADE_MODE_REAL) { Alert("Script operation is not allowed on a live account!"); return; } } //+------------------------------------------------------------------+ //| Receives the current number of orders with specified ORDER_MAGIC | //+------------------------------------------------------------------+ int GetOrdersTotalByMagic(long const magic_number) { ulong order_ticket; int total=0; //--- go through all pending orders for(int i=0; i<OrdersTotal(); i++) if((order_ticket=OrderGetTicket(i))>0) if(magic_number==OrderGetInteger(ORDER_MAGIC)) total++; //--- return(total); } // Define the OnTick function to check for hourly open void OnTick() { datetime currentTime = TimeLocal(); // Get the current time datetime currentHour = TimeHour(currentTime); // Get the hour component of the current time if (currentHour != lastTradeHour) { lastTradeHour = currentHour; if (!tradeOpened) { MqlTradeRequest request= {}; entryPrice = SymbolInfoDouble(_Symbol, SYMBOL_BID); stopLossPrice = entryPrice - stopLossPoints * _Point; takeProfitPrice = entryPrice + takeProfitPoints * _Point; // tradeOpened = OrderSend(_Symbol, 0, lotSize, entryPrice, 3, stopLossPrice, takeProfitPrice, "New Order", 0, 0, Green); request.action=TRADE_ACTION_PENDING; // setting a pending order request.magic=order_magic; // ORDER_MAGIC request.symbol=_Symbol; // symbol request.volume=lotSize; // volume in 0.1 lots request.sl=stopLossPrice; // Stop Loss is not specified request.tp=takeProfitPrice; // Take Profit is not specified //--- form the order type request.comment="New Order"; //--- form the price for the pending order request.price=entryPrice; // open price //--- send a trade request MqlTradeResult result= {}; OrderSend(request,result); if(result.retcode==10016) Print(result.bid,result.ask,result.price); //--- return code of the trade server } else { double currentPrice = SymbolInfoDouble(_Symbol, SYMBOL_BID); if (currentPrice >= takeProfitPrice || currentPrice <= stopLossPrice) { if (currentPrice >= takeProfitPrice) { tradeOpened = false; lotSize = initialLotSize; } else if (currentPrice <= stopLossPrice) { lotSize *= martingaleMultiplier; stopLossPrice = entryPrice - stopLossPoints * _Point; takeProfitPrice = entryPrice + takeProfitPoints * _Point; //tradeOpened = OrderSend(_Symbol, 0, lotSize, entryPrice, 3, stopLossPrice, takeProfitPrice, "New Order", 0, 0, Red); MqlTradeRequest request= {}; request.action=TRADE_ACTION_PENDING; // setting a pending order request.magic=order_magic; // ORDER_MAGIC request.symbol=_Symbol; // symbol request.volume=lotSize; // volume in 0.1 lots request.sl=stopLossPrice; // Stop Loss is not specified request.tp=takeProfitPrice; // Take Profit is not specified //--- form the order type request.comment="New Order"; //--- form the price for the pending order request.price=entryPrice; // open price //--- send a trade request MqlTradeResult result= {}; OrderSend(request,result); if(result.retcode==10016) Print(result.bid,result.ask,result.price); } } } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ int TimeHour(datetime date) { MqlDateTime tm; TimeToStruct(date,tm); return(tm.hour); } //+------------------------------------------------------------------+
You have to check documentation of OrderSend and fix yourself, I just added some code so you can learn however this code is neither tested nor complied
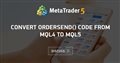
- 2020.12.18
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Errors:
'TimeHour' - undeclared identifier Line 19 Column 28
'currentTime' - some operator expected Line 19 Column 37
'OP_BUY' - undeclared identifier Line 31 Column 46
'OrderSend' - wrong parameters count Line 31 Column 27
'OP_BUY' - undeclared identifier Line 50 Column 54
'OrderSend' - wrong parameters count Line 50 Column 35