Skip the 2d method , go straight to classes + structs
so instead of having the 2nd dimension you have a structure , easier to code with .
class myTrade{ public: string symbol; int last_ticket; double last_open_price,next_open_price; double last_lot,next_lot; myTrade(void){reset();} ~myTrade(void){reset();} void reset(){ symbol=NULL; last_ticket=-1; last_open_price=0.0; next_open_price=0.0; last_lot=0.0; next_lot=0.0; } void save(int file_handle){ char text[]; int characters=StringToCharArray(symbol,text,0,StringLen(symbol),CP_ACP); FileWriteInteger(file_handle,characters,INT_VALUE); FileWriteArray(file_handle,text,0,characters); FileWriteInteger(file_handle,last_ticket,INT_VALUE); FileWriteDouble(file_handle,last_open_price); FileWriteDouble(file_handle,next_open_price); FileWriteDouble(file_handle,last_lot); FileWriteDouble(file_handle,next_lot); } void load(int file_handle){ reset(); char text[]; int characters_total=(int)FileReadInteger(file_handle,INT_VALUE); if(characters_total>0){ ArrayResize(text,characters_total,0); FileReadArray(file_handle,text,0,characters_total); symbol=CharArrayToString(text,0,characters_total,CP_ACP); } last_ticket=(int)FileReadInteger(file_handle,INT_VALUE); last_open_price=(double)FileReadDouble(file_handle); next_open_price=(double)FileReadDouble(file_handle); last_lot=(double)FileReadDouble(file_handle); next_lot=(double)FileReadDouble(file_handle); } }; myTrade Trades[];
Read the docs. of arrays: https://www.mql5.com/en/docs/array
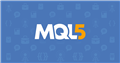
- www.mql5.com
Yeah , imagine there is a list of myTrades , that is the first dimension , and , each myTrade has 6 members , so that could be the second dimension but instead of index ([]) you call it with names (.symbol) , (.last_lot) etc and they can be of any type .
Please consider, that the advice given, is more correct than what your own understanding may be.
Your post clearly shows that the information you want to store should be in a structure and not an array, especially given that they are of different data-types.
The ticket number for example, is a ulong integer data-type and should really not be stored in a double data-type.
The previous example by @Lorentzos Roussos may be too complex for you to understand at first glance, so here is a simpler sample ...
In other words, you should be using a 1D array of a structure and not a 2D array.
// Declare the structure struct STradeData { ulong nTicket; // Ticket number of the previous open trade double dbOpenPrice, // Open Price of the previous open trade dbVolume, // Lot Size of the previous open trade dbNextPrice; // Next Trade price }; // Declare the array of the structure STradeData oTradeData[]; // Array of trade data // Usage example void myFunction(void) { // Set the trade data array size for 3 elements ArrayResize( oTradeData, 3 ); // Assign first element oTradeData[0].nTicket = nTicketPrevious; oTradeData[0].dbOpenPrice = 1.5000; // Previous open price oTradeData[0].dbVolume = 0.10; // Previous volume oTradeData[0].dbNextPrice = 1.5000 + 500 * _Point; // Next trade price = open + 500 points // ... etc. };
Double MyArray [] [];
MyArray [0] [0] = Here we will save the ticket number of the previous open trade.
MyArray [0] [1] = Here we will save the Open Price of the previous open trade.
MyArray [0] [2] = Here we will save the Lot Size of the previous open trade.
MyArray [0] [3] = Here we will save the Next Trade price by adding/subtracting the points to wait to previous trade open price.
Example : Last trade was opened at 1.5000
Next Trade = Open price of previous trade + PipsToWait;
Next Trade = 1.5000 + 500;
Next Trade = 1.5050;
MyArray [0] [4] = If the current market price has reached the next trade price then this bool will be true otherwise it will be false all the time .
then the [1][0] will start and keeps going on like this
If you insist ...
Forum on trading, automated trading systems and testing trading strategies
sd59, 2013.10.27 22:58
I am trying to generate a 2D array of high, low, open, close prices and I am totally lost as to how to fill the array.
double PriceArray[1000][4]; int init() { { for(int i=1;i<1000;i++) PriceArray[i][0] = iHigh(NULL,240,i); PriceArray[i][1] = iLow(NULL,240,i); PriceArray[i][2] = iOpen(NULL,240,i); PriceArray[i][3] = iClose(NULL,240,i); } Print(PriceArray[1][0]); Print(PriceArray[1][1]); Print(PriceArray[1][2]); Print(PriceArray[1][3]); return(0); }This doesn't look a great way to fill the array. Also for some reason the last print value is zero (i.e the iClose value for the first candle is zero - the first 3 values are correct.).
Is there a better way to do this?
thanks

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
what i want to achieve, kindly give me hint how to solve this task ?
Double PointsToWait = 500;
Double MyArray [] [];
MyArray [0] [0] = Here we will save the ticket number of the previous open trade.
MyArray [0] [1] = Here we will save the Open Price of the previous open trade.
MyArray [0] [2] = Here we will save the Lot Size of the previous open trade.
MyArray [0] [3] = Here we will save the Next Trade price by adding/subtracting the points to wait to previous trade open price.
Example : Last trade was opened at 1.5000
Next Trade = Open price of previous trade + PipsToWait;
Next Trade = 1.5000 + 500;
Next Trade = 1.5050;