I have bought data (in ASCII format) of some symbols not listed in my MT4. I can use History Center to import data to existing symbols, but how can I add my new symbols ?
- history center import
- HELP ME
- History Center
Open offline
"Open offline" ?!
How can I browse for my ascii data ? Or may I first conwert it to .hst format (how ?) and save it to appropriate directory ?
How can I browse for my ascii data ? Or may I first conwert it to .hst format (how ?) and save it to appropriate directory ?
HST format was published.
Period_converter script is sample of hst-files creating.
You can modify old csv2hst converter
Period_converter script is sample of hst-files creating.
You can modify old csv2hst converter
//+------------------------------------------------------------------+ //| CSV to HST converter | //| Copyright © 2003, MetaQuotes Software Corp. | //| http://www.metaquotes.ru | //+------------------------------------------------------------------+ #include <string.h> #include <stdlib.h> #include <stdio.h> #include <time.h> #pragma pack(1) struct RateInfo { time_t ctm; // current time in seconds since 01.01.1970 double open; double low; double high; double close; int vol; }; #pragma pack(8) struct HistoryHeader { int version; char copyright[64]; char symbol[8]; int period; int unused[16]; }; //+------------------------------------------------------------------+ //| usage 1: csv2hst SSSSSSSP.csv | //| usage 2: csv2hst file.csv SSSSSSSP.hst | //+------------------------------------------------------------------+ int main(int argc, char* argv[]) { FILE *in, *out; char *cp; int i, period; char inputfilename[256] = ""; char outputfilename[256] = ""; char symbol[8]; char buffer[256]; char *delimiter = ","; tm tt; RateInfo ri; HistoryHeader hdr; if (argc < 2) return -1; strcpy(inputfilename,argv[1]); strcpy(outputfilename,argv[1]); if ((cp = strstr(outputfilename, ".csv")) == NULL) return -2; if (argc > 2) strcpy(outputfilename,argv[2]); else { *cp=0; strcat(outputfilename, ".hst"); } if (stricmp(inputfilename, outputfilename) == 0) return -3; if ((cp = strstr(outputfilename, ".hst")) == NULL) return -4; if ((in = fopen(inputfilename, "ri")) == NULL) return -5; if ((out = fopen(outputfilename, "wb")) == NULL) { fclose(in); return -6; } // take period and symbol from output file name *cp=0; period=0; for (i=strlen(outputfilename)-1; i>=0; i--) { if ((outputfilename[i]<'0' || outputfilename[i]>'9') && period == 0) { period = atoi(outputfilename+i+1); outputfilename[i+1]=0; } if (outputfilename[i]=='\\' || outputfilename[i]=='/') break; } strncpy(symbol, outputfilename+i+1, 7); symbol[7] = 0; // prepare and write hst header memset(&hdr, 0, sizeof(HistoryHeader)); hdr.version = 1; hdr.period = period; strcpy(hdr.symbol, symbol); strcpy(hdr.copyright, "Copyright © 2003, MetaQuotes Software Corp."); fwrite(&hdr, sizeof(HistoryHeader), 1, out); // prepare and write RateInfo records i=0; while (fgets(buffer, 255, in) != NULL) { buffer[255] = 0; cp = buffer; //---- date DD.MM.YYYY tt.tm_mday = atoi(cp); if (tt.tm_mday < 1 || tt.tm_mday > 31) continue; if ((cp = strstr(cp, ".")) == NULL) continue; tt.tm_mon = atoi(cp+1) - 1; if (tt.tm_mon < 0 || tt.tm_mon > 11) continue; if ((cp = strstr(cp+1, ".")) == NULL) continue; tt.tm_year = atoi(cp+1); if (tt.tm_year > 1900) tt.tm_year -= 1900; if (tt.tm_year < 50) tt.tm_year += 100; //---- time ,HH:MI if ((cp = strstr(buffer, delimiter)) == NULL) continue; tt.tm_hour = atoi(cp+1); if (tt.tm_hour < 0 || tt.tm_hour > 23) continue; if ((cp = strstr(cp+1, ":")) == NULL) continue; tt.tm_min = atoi(cp+1); if (tt.tm_min < 0 || tt.tm_min > 59) continue; if ((ri.ctm = mktime(&tt)) == 0) continue; ri.ctm -= _timezone; //---- open,high,low,close,volume if ((cp = strstr(cp, delimiter)) == NULL) continue; if ((ri.open = atof(cp+1)) <= 0) continue; if ((cp = strstr(cp+1, delimiter)) == NULL) continue; if ((ri.high = atof(cp+1)) <= 0) continue; if ((cp = strstr(cp+1, delimiter)) == NULL) continue; if ((ri.low = atof(cp+1)) <= 0) continue; if ((cp = strstr(cp+1, delimiter)) == NULL) continue; if ((ri.close = atof(cp+1)) <= 0) continue; if ((cp = strstr(cp+1, delimiter)) == NULL) continue; if ((ri.vol = atoi(cp+1)) < 0) continue; //----- check prices if (ri.low > ri.open || ri.low > ri.close || ri.low > ri.high) continue; if (ri.high < ri.open || ri.high < ri.close || ri.high < ri.low) continue; if (gmtime(&ri.ctm) == NULL) continue; i++; fwrite(&ri,sizeof(RateInfo),1,out); } fclose(in); fclose(out); printf("%d records written\n", i); return 0; }
Hi Slawa,
I have no C background (C# & VB.NET), but would like to use your awesome trading platform to analyse and backtest other markets than the FX, so I would need to add new symbols and prepare hst files FROM ASCII data.
Would you please clarify on those two questions:
1. Should "Copyright © 2003, MetaQuotes Software Corp." be the only text in the header immediately followed by the data? No Sumbol & time period(eg. AUDUSD1440).
2. Are you writing the whole data block (datetime,0:00,open,high,low,close,volume) without any other separators (besides the COMMA)?
I tried to compile the code but hit an error on line "tm tt;" : variable 'tt' not found.
Can you please help me decypher the C code above?
Thanks in advanve for your help!
I have no C background (C# & VB.NET), but would like to use your awesome trading platform to analyse and backtest other markets than the FX, so I would need to add new symbols and prepare hst files FROM ASCII data.
Would you please clarify on those two questions:
1. Should "Copyright © 2003, MetaQuotes Software Corp." be the only text in the header immediately followed by the data? No Sumbol & time period(eg. AUDUSD1440).
2. Are you writing the whole data block (datetime,0:00,open,high,low,close,volume) without any other separators (besides the COMMA)?
I tried to compile the code but hit an error on line "tm tt;" : variable 'tt' not found.
Can you please help me decypher the C code above?
Thanks in advanve for your help!
bbzz,
the simplest way for you i think (also the quickest/dirtiest), is going to Tools/History center
then just pick one pair/period that you are not going to use.
press import, BROWSE, choose "ascii" file type and pick your file.
as for the format, you can just pick a pair with existing data and use the export button (.csv)
watch it with notepad and you will know the format
m
the simplest way for you i think (also the quickest/dirtiest), is going to Tools/History center
then just pick one pair/period that you are not going to use.
press import, BROWSE, choose "ascii" file type and pick your file.
as for the format, you can just pick a pair with existing data and use the export button (.csv)
watch it with notepad and you will know the format
m
How can I add new symbols in mt5?
By Default it’s only showing 4/4 in market watch
TariSingh:
How can I add new symbols in mt5?
By Default it’s only showing 4/4 in market watch
There are no any symbols by default.
Because MT5 is the software, and the brokers are using it to propose the symbols to trade.
There are a lot of the brokers, and they all are proposing the different symbols to trade.
----------------
Find a Server by the Broker's Name:
https://www.mql5.com/en/forum/9950/page88#comment_3981953
https://www.mql5.com/en/forum/214820
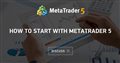
How to Start with Metatrader 5
- 2017.01.05
- www.mql5.com
I decided to create this thread to help to myself and to the others to start with Metatrader 5...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register