Buffers in custom indicators can be accessed in EAs and scripts.
You should create a buffer for your boolean variable. Buffers can only be of double type. So you set the buffer to +1 for true and 0 for false.
To access the buffer values you should create the indicator handle in your code use CopyBuffer to access its values.
Buffers in custom indicators can be accessed in EAs and scripts.
You should create a buffer for your boolean variable. Buffers can only be of double type. So you set the buffer to +1 for true and 0 for false.
To access the buffer values you should create the indicator handle in your code use CopyBuffer to access its values.
That sounds like the best approach. I will use 1 and 0 instead of true/false for the double buffers. I guess I can use iCustom then in the EA.
After further thinking, I decided not to use iCustom, as this is designed for obtaining data from the indicator buffers. Instead I used "GlobalVariableGet".
https://www.mql5.com/en/docs/globals/globalvariableget
My indicator is set up like this:
// global variables for signals double gLongSignal = 0.0; double gShortSignal = 0.0; // conditions if (longSignalCondition){ gLongSignal = 1.0; gShortSignal = 0.0; } else if(shortSignalCondition){ gLongSignal = 0.0; gShortSignal = 1.0; }
now my EA looks like this:
#include <Trade/Trade.mqh> //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ CTrade trade; // Access global variable values double longSignalValue; double shortSignalValue; int OnInit() { return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { longSignalValue = GlobalVariableGet("gLongSignal"); shortSignalValue = GlobalVariableGet("gShortSignal"); if(shortSignalValue == 1.0){ for(int i=PositionsTotal()-1; i>=0; i--){ ulong posTicket = PositionGetTicket(i); if(posTicket>0){ if(PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_BUY){ trade.PositionClose(posTicket); // close position } } } if(PositionsTotal() == 0){ trade.Sell(0.03); //make one position with selected lot size } } if(longSignalValue == 1.0){ for(int i=PositionsTotal()-1; i>=0; i--){ ulong posTicket = PositionGetTicket(i); if(posTicket>0){ if(PositionGetInteger(POSITION_TYPE) == POSITION_TYPE_SELL){ trade.PositionClose(posTicket); // close position } } } if(PositionsTotal() == 0){ trade.Buy(0.03); //make one position with selected lot size } } } //+------------------------------------------------------------------+
However I can't test this in the strategy tester as it doesn't work, perhaps because I need the real-time connection between the indicator and EA on a live chart. I can't test it right now as the markets are closed
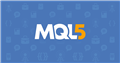
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I wanted to evolve one of my indicators into an EA just to see if it would be possible. My indicator makes one bool variable turn true when a certain condition is met in OnCalculate, and makes another bool variable turn true with another condition is met. I wanted to use these boolean values for generation of long signals and short signals. I tried making a custom signal script, but have gotten lost trying to figure out how to get the global variable data from the indicator. I don't think what I want to achieve can be done with iCustom as this is for obtaining data generated by the indicator itself, but not for getting separate boolean vals. There could be a few alternative ways to make this work such as writing out data to a file..but I want to know if you know of any simplified way of achieving this.