You need to correct the check function like this:
bool CheckStopLoss_Takeprofit(ENUM_ORDER_TYPE type, double SL, double TP) { double price = SymbolInfoDouble(_Symbol, type == ORDER_TYPE_BUY ? SYMBOL_BID : SYMBOL_ASK); //--- get the SYMBOL_TRADE_STOPS_LEVEL level int stops_level = (int)SymbolInfoInteger(_Symbol,SYMBOL_TRADE_STOPS_LEVEL); if(stops_level != 0) { LOG(LOG_LEVEL_INFO, StringFormat("SYMBOL_TRADE_STOPS_LEVEL=%d: StopLoss and TakeProfit must"+ " not be nearer than %d points from the closing price", stops_level, stops_level)); } else LOG(LOG_LEVEL_INFO, "Symbol " + _Symbol + " has no trade stops level set");The check for SL/TP is done against the exit price for the trade (NOT its entry price).
Thank you all for replying. amrali, that's exactly what I pass into the function from the above code. Sell is called with the current bid value as the price.
I think I've narrowed it down to the spread and setting a SL that is under the current spread. So I'm working my code around to factor spread in now.
Thank you all for replying. amrali, that's exactly what I pass into the function from the above code. Sell is called with the current bid value as the price.
I think I've narrowed it down to the spread and setting a SL that is under the current spread. So I'm working my code around to factor spread in now.
Thank you for the reply!
I went back to the source page and noticed what you were saying. https://www.mql5.com/en/articles/2555
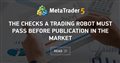
- www.mql5.com
Thank you for the reply!
I went back to the source page and noticed what you were saying. https://www.mql5.com/en/articles/2555
SL or TP is irrelevant. They are both related to the close price.
Are you thinking you have 2 different close prices depending if you are in profit or loss ?
Admittedly I just took the function (and butchered it) without really thinking about what it does. Now that I'm thinking about it, I'm not 100% sure I get what its doing. I thought its goal was to ensure you were not trying to place a Buy/Sell trade with a SL or TP that would violate the minimum distance required by the broker for a stop order. Reading the description from the reference section though I'm not so sure.
SYMBOL_TRADE_STOPS_LEVEL | Minimal indention in points from the current close price to place Stop orders |
Reading the above this seems to be a minimum distance from the Bid price (bid = close) for any stop loss to be set. So if I'm about to place a Sell, my thinking is that I would want to make sure that the SL is at least above the trade_stops level (and honestly above the ASK otherwise its gonna instant close anyways!) If I'm placing a buy I think the SL should be trade_stops level below the current bid price.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
All,
I've been slowly implementing all the things I can think of to prevent this error and I'm running out of ideas. Here is all the functions that do something leading up to a Buy/Sell. I check margins, I check Positions, I check for Trade Stop Levels (this symbol has none it seems). See attached pic for the errors/logs. The SL/TP calculation looks good to me, as in the price numbers chosen are accurate.