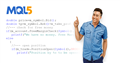
- 2023.02.10
- www.mql5.com
Hi.
I'm still new when it comes to MQL5 code and I'd like some help with creating an MQL5 EA function that will return the risk needed to be placed on a trade this is what it needs to follow.
On the first trade risk 1% of the starting account balance(not the account equity), if the trade was a winner risk 2.5% on the next trade,
if we have 2 wins in a row go back and risk 1% on the next trade, then if that trade was a winner risk 2.5%, if that was a winner risk 1% make this a continues loop.
Thank you.
Hi , risk % as in the amount lost if SL is hit or as the margin for the trade ?
You will also need to maintain tabs on the open trades , do you have that code or is that also required .Hi , risk % as in the amount lost if SL is hit or as the margin for the trade ?
You will also need to maintain tabs on the open trades , do you have that code or is that also required.Hi Lorentzos yes risk % in terms of when SL is hit.
This is the dummy code I have at the moment I'm just not sure how exactly I can make it work.
If possible could you show me code or send me a link on how one can store the previous account balance in an array and then store the new account balance after a trade is closed in a separate array
and use the two arrays to compare the balance
//Dummy code double GetRiskpercent() { //get the starting balance. then risk 1% on the first trade if trade is won and account is up 1.5%. //then risk 2.5% on the next tarde. if the account is up 3.75%. go back to risking 1% and continue that loop. //but if account is down -1% or -2.5% risk only 1% on the next trade. double Risk = 0.01; double accountPrevious = AccountInfoDouble( ACCOUNT_BALANCE ); //previous account double accountCurrent = AccountInfoDouble(ACCOUNT_BALANCE); //current account for (int i = 0; i < OrdersTotal(); i++) { if (accountCurrent >= (accountPrevious * 1.5 / 100.0)) { Risk = 2.5; } else if (accountCurrent >= ( accountPrevious * 3.75 / 100.0)) { Risk = 1.0; } else if (accountCurrent <= ( accountPrevious * -1.0 / 100.0)) { Risk = 1.0; } } return Risk; }
Please edit your post (don't create a new post) and replace your code properly (with "</>" or Alt-S), or attach the original file directly with the "+ Attach file" button below the text box.
NB! Very important! DO NOT create a new post. EDIT your original post.
You still have a long way to go in MQL programming before you will be able to implement asymmetric compounding.
I suggest you start learning to code in MQL with much simpler programs. Study the CodeBase examples and only once you fully understand and can program simpler goals can you then slowly grow the complexity.
Either that or hire someone to code it for you.
Hi Lorentzos yes risk % in terms of when SL is hit.
This is the dummy code I have at the moment I'm just not sure how exactly I can make it work.
If possible could you show me code or send me a link on how one can store the previous account balance in an array and then store the new account balance after a trade is closed in a separate array
and use the two arrays to compare the balance
**Dummy code**
//but if account is down -1% or -2.5% risk only 1% on the next trade.
try this :
#property copyright "read the discussion" #property link "https://www.mql5.com/en/forum/441527" #property version "1.00" /* kay let's create a "gearbox" structure for risk It will work like this : when the balance changes it will register a win or a loss Flaws : -If more than one trades close in between the check of the change , it wont see it -If you deposit / withdraw in the account it will see it and shift gear -If it dead loads with a big gap in between (meaning trades have occured) it will aggregate all of them in one balance change So , what we need is a risk list for wins and one for losses , a counter for wins , one for losses and a balance value. We create a structure that has these values */ struct risk_gearbox{ //the list of win risks double win_risk[]; double loss_risk[]; double default_risk; int wins,losses; double balance; bool last_was_win,last_was_loss; risk_gearbox(void){reset();} //first thing we need , reset void reset(){ ArrayFree(win_risk); ArrayFree(loss_risk); wins=0; losses=0; balance=0.0; default_risk=0.0; last_was_win=false; last_was_loss=false; } //then , add an item in the list private: int add_to_list(double &list[],//which list , & double value){//the new value int new_size=ArraySize(list)+1;//find new list size (existing + 1) ArrayResize(list,new_size,0);//change list size (add 1) list[new_size-1]=value;//the last (newest) list item is sitting at [new_size-1] because arrays (lists) start from [0] return(new_size-1);//we send the location of the newest item to the caller (new_size-1) }//easy //then , add to win list and add to loss list , we will use the function above int add_to_win_risk(double value){ //we call the adder above , we send the win risk list and the new value to add return(add_to_list(win_risk,value)); } int add_to_loss_risk(double value){ //same for loss risk list return(add_to_list(loss_risk,value)); } /* awesome but how will the user (coder) start this ? we need a setup function We need to send a list of risk values for the wins and one for the losses We'll use text */ public: void setup(string comma_separated_win_risks, string comma_separater_loss_risks, double _default_risk){ //the user sends 2 texts with their desired "risk gears" for each outcome //we must split them and turn them to double values and place them in the arrays (lists) //so , we will separate by comma ushort separator=StringGetCharacter(",",0); //string for results of the split string split_parts[]; //separate the win risk text first //Get comma separated win risks text and split it by separator and place the parts in split parts , and give me the number of parts in total_win_risks int total_win_risks=StringSplit(comma_separated_win_risks,separator,split_parts); //so , are there any win risks ? if(total_win_risks>0){ //loop in each "part" and turn it to a double value and throw it in the list for(int i=0;i<total_win_risks;i++){ add_to_win_risk((double)StringToDouble(split_parts[i])); } } //similarly for the loss risks //throw the trash out , we don't need the split parts anymore ArrayFree(split_parts); //and do it again for the loss risks int total_loss_risks=StringSplit(comma_separater_loss_risks,separator,split_parts); if(total_loss_risks>0){ for(int i=0;i<total_loss_risks;i++){ add_to_loss_risk((double)StringToDouble(split_parts[i])); } } //cool now we have 2 lists with risks , our gears //the default risk default_risk=_default_risk; //we also grab the balance balance=AccountInfoDouble(ACCOUNT_BALANCE); //ready } /* getting there , what do we need now ? We need to monitor the balance and shift gears */ void check(bool reset_opposite=true){ //so first get the current balance double _new_balance=AccountInfoDouble(ACCOUNT_BALANCE); //if we detect a win if(_new_balance>balance){ //we set the last to win last_was_win=true; last_was_loss=false; //we increase the wins wins++; //if the wins is bigger than our list ! we go back to 1 if(wins>ArraySize(win_risk)){wins=1;} //and if the user wants to reset the opposite counter we do it here if(reset_opposite){losses=0;} } //same for the loss else if(_new_balance<balance){ last_was_loss=true; last_was_win=false; losses++; if(losses>ArraySize(loss_risk)){losses=1;} if(reset_opposite){wins=0;} } //and set the balance as the new one balance=_new_balance; } //awesome , but what if you want to reset without removing the gears? void reset_gear_state(){ wins=0; losses=0; last_was_win=false; last_was_loss=false; } //and lastly how on earth do we use this ? we ask for a risk value double what_is_the_risk(){ //case a : last was a win if(last_was_win){ //we return the risk in the gear slot wins-1 return(win_risk[wins-1]); } //case b : last was a loss else if(last_was_loss){ //we return the risk in the gear slot losses-1 return(loss_risk[losses-1]); } //case c : default risk return(default_risk); } }; //oof , now , create our gearbox variable risk_gearbox RISKGEARBOX;//type is risk_gearbox , name is RISKGEARBOX int OnInit() { //--- /* setup the gearbox up with : wins : 1st win 2.5% 2nd win 1.0% then if we win again 2.5% again because it will shift to the start losses : 1st loss 1.0% 1 loss gear Default risk 1% */ RISKGEARBOX.setup("2.5,1.0","1.0",1.0); //--- return(INIT_SUCCEEDED); } void OnTick() { //--- //maintain risk gearbox RISKGEARBOX.check(true); //and if you want to trade this is how you get the risk double to_risk=RISKGEARBOX.what_is_the_risk(); }
OHH yer like this yer yer yer
// Declare input parameters
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor
You still have a long way to go in MQL programming before you will be able to implement asymmetric compounding.
I suggest you start learning to code in MQL with much simpler programs. Study the CodeBase examples and only once you fully understand and can program simpler goals can you then slowly grow the complexity.
Either that or hire someone to code it for you.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi.
I'm still new when it comes to MQL5 code and I'd like some help with creating an MQL5 EA function that will return the risk needed to be placed on a trade this is what it needs to follow.
On the first trade risk 1% of the starting account balance(not the account equity), if the trade was a winner risk 2.5% on the next trade,
if we have 2 wins in a row go back and risk 1% on the next trade, then if that trade was a winner risk 2.5%, if that was a winner risk 1% make this a continues loop.
Thank you.