Your code | if(AccountInfoDouble(ACCOUNT_BALANCE) <= 9000) // See if in a drawdown { safemode = true; } else { safemode = false; } |
Simplified | safemode = AccountInfoDouble(ACCOUNT_BALANCE) <= 9000; // See if in a drawdown |
Your code | |
Simplified |
Thank you. I will update my code that way.
It doesn't change the error code problem, tho.
What a trade request needs is written here: https://www.mql5.com/en/docs/constants/structures/mqltraderequest
If your broker uses Request or Instant Execution you need to set the price value. The current Bid-value for Sells and the Ask-value for Buys.
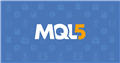
- www.mql5.com
What a trade request needs is written here: https://www.mql5.com/en/docs/constants/structures/mqltraderequest
If your broker uses Request or Instant Execution you need to set the price value. The current Bid-value for Sells and the Ask-value for Buys.
Yes, I read that page several times and I still not find what wrong with my request. I try to add the price in the request, doesn't change a thing. I check the value of SL/TP/Size, all correct.
I still don't see what I miss…
Yes, I read that page several times and I still not find what wrong with my request. I try to add the price in the request, doesn't change a thing. I check the value of SL/TP/Size, all correct.
I still don't see what I miss…
Change-
MqlTradeRequest request; MqlTradeResult result;
To-
MqlTradeRequest request = {}; MqlTradeResult result = {};

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I stuck with this error, I'm looking where the mistake is for the last 24h, and I'm completely blind.
The error appear in the strategy tester log like this : "invalid request" and with the retcode 10013.
Below is the full code. It's a training code where I try different and basic trading logic, I don't except it to be profitable, I just want to see it work correctly without error...