int x=0; int y=0; double z=0; //Why is this a double? double Array[x][y][z]; //[Bars][SwingNr][Price]
This is the same as
double Array[0][0][0];
You resize the first index
ArrayResize(Array,Bars);
So you have
Array[Bars][0][0];
The 2nd and 3rd dimensions size are still zero
Array[x]=value; Array[y]=othervalue; Array[z]=price;
This is the same as
Array[0]=value; Array[0]=othervalue; Array[0]=price;
You are trying to assign values to a 3D array using only 1 dimension.
"All I want to do is declare a 2D array with three variables stored in it."
double Array[x][y][z];
The above is not a 2d array with three variables in it.
double Array[][3];
This is the same as
You resize the first index
So you have
The 2nd and 3rd dimensions size are still zero
This is the same as
You are trying to assign values to a 3D array using only 1 dimension.
I'm guessing this makes a lot more sense. :)
double Array[3][1]; ArrayInitialize (Array,0); ArrayResize(Array,Bars); //So how do I assign the following values to the array? double value = 125; //this is bars value and has the largest amount of double othervalue = 2; //this is an integer (the number of the "zigzag" at the bar value double price = 1.2345; //this is the price at the bar / swing
So when I think of an array, this is what I see in my mind: (obviously without the headers)
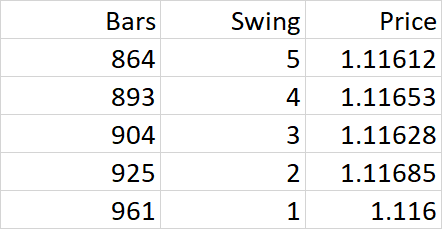
But I'm guessing I'm wrong there...
Am I correct that if I wanted to get the value for the price at swing 4 (1.11653), I would enter:
double Price = array[2][3];
But how do I get about getting all three values?
For example, how would I get the values associated with bar number 864?
Apologies for the very n00b questions, but for some reason I'm struggling with wrapping my head around arrays.
Thanks for the help. It's much appreciated. :)
So I think I just came up with the answer to my own question.
Very helpful! Thank you.
One thing I do not understand though is how to access singular values or series of values from within the array. It's just spitting 0.0's at me.
My eventual goal is to get a range of values from the array and then perform calculations with them.
So for example, I want to get swing 3 to 7, get the associated close prices and average them.
I would expect to just go
Double Average = ((Array[3][2]+Array[4][2]+Array[5][2]+Array[6][2])/2); (or something to that end), but guessing it's not that simple.
Here's the code I have.... Hope you can give me a pointer in the right direction.
if(NewBar()==true) { ObjectsDeleteAll(0); DrawSwingPoints(); double ZZValue[]; ArrayResize(ZZValue,sBars); //======================Me visualising array data================================================= //Array[Bars][2] //[0] Bars [961] [925] [904] [893] [864] [839] [820] [806] [801] [791] //[1] Swing [ 1 ] [ 2 ] [ 3 ] [ 4 ] [ 5 ] [ 6 ] [ 7 ] [ 8 ] [ 9 ] [ 10] //[2] Price[1.116][1.11685][1.11628][1.11653][1.11612][1.11644][1.11624][1.11671][1.11652][1.11666] //============================================================= double SwingArray[][3]; //[Bars][SwingNr][Price] ArrayInitialize (SwingArray,0); ArrayResize(SwingArray,sBars); //for( int i = 0; i<Bars; i++) //FROM MOST RECENT BAR TO OLDEST for( int i = Bars-1; i>0; i--) //FROM OLDEST BAR/SWING TO LATEST { double ZigZag=iCustom(Symbol(),_Period,"ZigZag",ExtDepth,ExtDeviation,ExtBackstep,0,i); if(ZigZag !=0) { int x; //y, z; BarNr = i; SwingNr++; ZZValue[SwingNr] = ZigZag; SwingArray[x][0] = BarNr; //integer (bar number) SwingArray[x][1] = SwingNr; //integer (basically also bar number) SwingArray[x][2] = ZigZag; //This is a double (price value) //ArraySort(SwingArray,WHOLE_ARRAY,0,MODE_DESCEND); //Arraysort does not seem to have the desired effect. //Print("BarNr - "+BarNr+" SwingNr - "+SwingNr+" --- "+ZZValue[SwingNr]); //Returns all 3 numbers as advertised //Print(SwingArray[x][0]); //returns bar numbers //Print(SwingArray[x][1]); //returns swing numbers //Print(SwingArray[x][2]); //returns price //Print(SwingArray[0][0]); //Returns all PRICES?? Only 1 Bar number (961) expected? Why do I get all of them? //Print(SwingArray[0][1]); //Returns all swing numbers. Again, Why all of them? //Print(SwingArray[0][2]); //Returns all prices. ... and again... why all of them? (1.116 expected) //Print(SwingArray[1][0]); //Returns all 0.0 //From here on out, no matter what combination I use. All 0.0 values. //Print(SwingArray[1][1]); //Returns all 0.0 //Print(SwingArray[1][2]); //Returns all 0.0 //Print(SwingArray[2][0]); //Returns all 0.0 //Print(SwingArray[2][1]); //Returns all 0.0 //Print(SwingArray[2][2]); //Retunrs all 0.0
I have mostly solved the issues I had and think I am slowly starting to understand what happening. :)
One thing I do not understand though is how to access singular values or series of values from within the array. It's just spitting 0.0's at me.
My eventual goal is to get a range of values from the array and then perform calculations with them.
So for example, I want to get swing 3 to 7, get the associated close prices and average them.
I would expect to just go
Double Average = ((Array[3][2]+Array[4][2]+Array[5][2]+Array[6][2])/2); (or something to that end), but guessing it's not that simple.
Here's the code I have.... Hope you can give me a pointer in the right direction.
You use variable "x" as the array index but no value is assigned to it.
int x; //y, z; BarNr = i; SwingNr++; ZZValue[SwingNr] = ZigZag; SwingArray[x][0] = BarNr; //integer (bar number) SwingArray[x][1] = SwingNr; //integer (basically also bar number) SwingArray[x][2] = ZigZag; //This is a double (price value)
//Array[Bars][2] //[0] Bars [961] [925] [904] [893] [864] [839] [820] [806] [801] [791] //[1] Swing [ 1 ] [ 2 ] [ 3 ] [ 4 ] [ 5 ] [ 6 ] [ 7 ] [ 8 ] [ 9 ] [ 10] //[2] Price[1.116][1.11685][1.11628][1.11653][1.11612][1.11644][1.11624][1.11671][1.11652][1.11666]
First thing you must do is decide what your search value or index value is, versus what you are storing.
Ignoring bar index for now, your index value is swing and you are storing price. That would be a one-dimensional array, not two dimensions. You use swing index in and get price out.
In your case, you still use swing index in and get either bar or price out. Therefor, you need a two-dimensional array, not three. The first index is swing index, and the second selects price or bar index out.
I wouldn't use that, as you have two different data types. I would create a single dimension array of a structure of what you are actually storing.
struct Data{ int iBar; double price }; Data ZZvalue[]; ArrayResize(ZZvalue,SwingNr+1); ZZvalue[SwingNr].price = ZigZag; ZZvalue[SwingNr].iBar = i; SwingNr++;
It's easy to overlook stuff isn't it? Especially when tired and have been looking at the screen for way too long.
Thanks. :)
I'll see if I continue with this "version" or try William Roeder's suggestion, which look like it will simplify things a bit. (once I get my head around structures)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The bar number at a particular swing (from zigzag) and the price associated with that bar/swing
(if you want, I can post the actual code, but figured this is a bit clearer)