Doubles are binary floating point numbers (not decimal fixed point). You can't control the number of digits directly. You can round them to the closest value but it will still not be exact because they are binary and not decimal.
You can however, display or print them to fixed decimal places when using DoubleToString(), but that is only for display purposes and should never be used for calculations.
Also read the following ...
Forum on trading, automated trading systems and testing trading strategies
Heavy "Feature" with doubles produces unpredictable errors in EAs
William Roeder, 2019.07.16 14:13
NormalizeDouble, It's use is usually wrong.
- Floating point has infinite number of decimals, it's your not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopedia- Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
- SL/TP (stops) need to be normalized to tick size (not Point.) (On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum) and abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum
- Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on Metals. So do it right: Trailing Bar Entry EA - MQL4 programming forum or Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum
- Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
- MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - General - MQL5 programming forum
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum
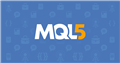
- www.mql5.com
Doubles are binary floating point numbers (not decimal fixed point). You can't control the number of digits directly. You can round them to the closest value but it will still not be exact because they are binary and not decimal.
You can however, display or print them to fixed decimal places when using DoubleToString(), but that is only for display purposes and should never be used for calculations.
Also read the following ...
DoubleToString() provides a string to 8 decimal places unless manually specified. I am trying to dynamically get the number of decimal places that corresponds with what a pair displays on the chart.
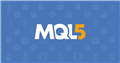
- www.mql5.com
Number of decimal places |
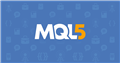
- www.mql5.com
I am doing a number of calculations involving OHLC of candles. I want all my calculations to be reduced to the number of decimal places that appear on a given pair. For example, GBPUSD shows chart prices to 5 decimal places (eg, 1.12333) so, I would want my double values to be formatted to 5 decimal places, also GBPJPY shows chart prices to 3 decimal places (eg. 160.080) so I'd want doubles to be formatted to 3 decimal places and so on.
So I am trying to get my doubles to be formatted to the number of decimal places that corresponds to a given pair. How can I do this?
If you face strange issues with doubles (and mostly, you will), you can try my library on the codebase.
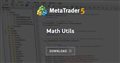
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am doing a number of calculations involving OHLC of candles. I want all my calculations to be reduced to the number of decimal places that appear on a given pair. For example, GBPUSD shows chart prices to 5 decimal places (eg, 1.12333) so, I would want my double values to be formatted to 5 decimal places, also GBPJPY shows chart prices to 3 decimal places (eg. 160.080) so I'd want doubles to be formatted to 3 decimal places and so on.
So I am trying to get my doubles to be formatted to the number of decimal places that corresponds to a given pair. How can I do this?