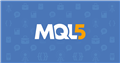
- www.mql5.com
- Ask!
- Different Buffers = No Data Window Labels and Values
- How to hide lot size of my trade on chart ?
Don't set the array as a buffer.
I want to hide/show my indicator plot based on a criteria. If I set the color to clrNONE, I still get a pop-up on mouseover of the invisible plot. That's still the simplest way to hide the indicator though so I wonder if there is a similarly simple solution to this or if I should just forget about the mouseover popup issue and stick to changing the color to show/hide the plot? Thanks for any advice you can share.
#property indicator_chart_window #property indicator_buffers 1 #property indicator_plots 1 input bool inpShowBuf=true; //--- int hnd; double buffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping hnd=iMA(_Symbol,_Period,100,0,MODE_SMA,PRICE_CLOSE); if(hnd<0) return INIT_FAILED; SetIndexBuffer(0,buffer,INDICATOR_DATA); if(inpShowBuf) { PlotIndexSetInteger(0, PLOT_SHOW_DATA,true); PlotIndexSetInteger(0, PLOT_LINE_COLOR,clrRed); PlotIndexSetInteger(0, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0, PLOT_DRAW_TYPE,DRAW_LINE); PlotIndexSetInteger(0, PLOT_LINE_WIDTH,1); } else { PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(0,PLOT_SHOW_DATA,false); } ArraySetAsSeries(buffer,true); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- double val[1]; //--- running indicator start formula int limit=rates_total-1-prev_calculated; for(int iBar = limit; iBar >= 0 && !IsStopped(); --iBar) { CopyBuffer(hnd,0,iBar,1,val); buffer[iBar]=val[0]; } //--- return value of prev_calculated for next call return(rates_total-1); }
The suggestion from Amir only seems to work well if you don't have other plots being drawn with the same indicator or if the plot you want to hide only uses one buffer.
Not really
#property indicator_chart_window #property indicator_buffers 3 #property indicator_plots 3 input bool inpShowBufLower=true; input bool inpShowBufMiddle=true; input bool inpShowBufUpper=true; //--- int hnd_bb; double middle_buffer[]; double upper_buffer[]; double lower_buffer[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping hnd_bb=iBands(_Symbol,_Period,50,0,2,PRICE_CLOSE); if(hnd_bb==INVALID_HANDLE) return INIT_FAILED; SetIndexBuffer(0,middle_buffer,INDICATOR_DATA); SetIndexBuffer(1,upper_buffer,INDICATOR_DATA); SetIndexBuffer(2,lower_buffer,INDICATOR_DATA); if(inpShowBufMiddle) { PlotIndexSetInteger(0, PLOT_SHOW_DATA,true); PlotIndexSetInteger(0, PLOT_LINE_COLOR,clrYellow); PlotIndexSetInteger(0, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0, PLOT_DRAW_TYPE,DRAW_LINE); PlotIndexSetInteger(0, PLOT_LINE_WIDTH,1); } else { PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(0,PLOT_SHOW_DATA,false); } if(inpShowBufUpper) { PlotIndexSetInteger(1, PLOT_SHOW_DATA,true); PlotIndexSetInteger(1, PLOT_LINE_COLOR,clrGreen); PlotIndexSetInteger(1, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(1, PLOT_DRAW_TYPE,DRAW_LINE); PlotIndexSetInteger(1, PLOT_LINE_WIDTH,1); } else { PlotIndexSetInteger(1,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(1,PLOT_SHOW_DATA,false); } if(inpShowBufLower) { PlotIndexSetInteger(2, PLOT_SHOW_DATA,true); PlotIndexSetInteger(2, PLOT_LINE_COLOR,clrRed); PlotIndexSetInteger(2, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(2, PLOT_DRAW_TYPE,DRAW_LINE); PlotIndexSetInteger(2, PLOT_LINE_WIDTH,1); } else { PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(2,PLOT_SHOW_DATA,false); } ArraySetAsSeries(upper_buffer,true); ArraySetAsSeries(lower_buffer,true); ArraySetAsSeries(middle_buffer,true); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- double bb_low[1],bb_mid[1],bb_high[1]; //--- running indicator start formula int limit=rates_total-1-prev_calculated; for(int iBar = limit; iBar >= 0 && !IsStopped(); --iBar) { CopyBuffer(hnd_bb,BASE_LINE,iBar,1,bb_mid); middle_buffer[iBar]=bb_mid[0]; CopyBuffer(hnd_bb,UPPER_BAND,iBar,1,bb_high); upper_buffer[iBar]=bb_high[0]; CopyBuffer(hnd_bb,LOWER_BAND,iBar,1,bb_low); lower_buffer[iBar]=bb_low[0]; } //--- return value of prev_calculated for next call return(rates_total-1); }
*edit* try this modified version of your code and you'll see what I mean:
#property indicator_chart_window #property indicator_buffers 6 #property indicator_plots 3 input bool inpShowBufLower=true; input bool inpShowBufMiddle=true; input bool inpShowBufUpper=true; //--- int hnd_bb; double middle_buffer1[], middle_buffer2[]; double upper_buffer1[], upper_buffer2[]; double lower_buffer1[], lower_buffer2[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- indicator buffers mapping hnd_bb=iBands(_Symbol,_Period,50,0,2,PRICE_CLOSE); if(hnd_bb==INVALID_HANDLE) return INIT_FAILED; SetIndexBuffer(0,middle_buffer1,INDICATOR_DATA); SetIndexBuffer(1,middle_buffer2,INDICATOR_DATA); SetIndexBuffer(2,upper_buffer1,INDICATOR_DATA); SetIndexBuffer(3,upper_buffer2,INDICATOR_DATA); SetIndexBuffer(4,lower_buffer1,INDICATOR_DATA); SetIndexBuffer(5,lower_buffer2,INDICATOR_DATA); if(inpShowBufMiddle) { PlotIndexSetInteger(0, PLOT_SHOW_DATA,true); PlotIndexSetInteger(0, PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(0, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0, PLOT_LINE_WIDTH,1); PlotIndexSetInteger(0, PLOT_LINE_COLOR,clrYellow); } else { PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(0,PLOT_SHOW_DATA,false); } if(inpShowBufUpper) { PlotIndexSetInteger(1, PLOT_SHOW_DATA,true); PlotIndexSetInteger(1, PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(1, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(1, PLOT_LINE_WIDTH,1); PlotIndexSetInteger(1, PLOT_LINE_COLOR,clrGreen); } else { PlotIndexSetInteger(1,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(1,PLOT_SHOW_DATA,false); } if(inpShowBufLower) { PlotIndexSetInteger(2, PLOT_SHOW_DATA,true); PlotIndexSetInteger(2, PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(2, PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(2, PLOT_LINE_WIDTH,1); PlotIndexSetInteger(2, PLOT_LINE_COLOR,clrRed); } else { PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_NONE); PlotIndexSetInteger(2,PLOT_SHOW_DATA,false); } ArraySetAsSeries(upper_buffer1,true); ArraySetAsSeries(lower_buffer1,true); ArraySetAsSeries(middle_buffer1,true); ArraySetAsSeries(upper_buffer2,true); ArraySetAsSeries(lower_buffer2,true); ArraySetAsSeries(middle_buffer2,true); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- double bb_low[1],bb_mid[1],bb_high[1]; //--- running indicator start formula int limit=rates_total-2-prev_calculated; for(int iBar = limit; iBar >= 0 && !IsStopped(); --iBar) { CopyBuffer(hnd_bb,BASE_LINE,iBar,1,bb_mid); middle_buffer1[iBar]=middle_buffer2[iBar+1]; middle_buffer2[iBar]=bb_mid[0]; CopyBuffer(hnd_bb,UPPER_BAND,iBar,1,bb_high); upper_buffer1[iBar]=upper_buffer2[iBar+1]; upper_buffer2[iBar]=bb_high[0]; CopyBuffer(hnd_bb,LOWER_BAND,iBar,1,bb_low); lower_buffer1[iBar]=lower_buffer2[iBar+1]; lower_buffer2[iBar]=bb_low[0]; } //--- return value of prev_calculated for next call return(rates_total-1); }
In this version, what happens is when you switch to DRAW_NONE, the number of buffers required for the plot changes from 2 to 1 and although I don't know how to describe this with more intelligent words, it simply "screws" up the way your buffer sequence is matched to each plot. I have wondered if there was a way to work around this with a simple edit, but I haven't found it. Which leads me to think that I might need to use 12 buffers instead, the first 6 are the same and the next 12 are INDICATOR_CALCULATION buffers. These last 6 are the ones who hold all values no matter if the plot is to be shown or not. Then what I could do is if a plot is set to be drawn, I copy values from its two INDICATOR_CALCULATIONS buffers to its two INDICATOR_DATA buffers. If the plot is to be hidden, I fill its two INDICATOR_DATA buffers with empty values. So instead of using DRAW_NONE to hide the plot, I would rely on empty values to "hide" the buffer, while the plot values are still stored in DATA_CALCULATIONS buffers in case other plots need these values for their calculations, so that these other plots aren't affected if the first plot is hidden. I don't know if this is the best solution, but it's the only one I understand so far.
PS: Like I mentioned in my first post, I could easily change the color of the plots to clrNONE as a simpler way of removing them. And honestly, for some setups, that could be fine. I just found that for some applications I sometimes struggle with this method as the chart starts being filled with invisible plots that I end up creating annoying pop-ups on mouseovers that sometimes prevent me from getting the pop-up on mouseover for the plot I actually want info from.
I think so, unfortunately. But only if like I said, the plot you want to hide uses more than one buffer and you're also printing other plots with the indicator (maybe I didn't say this clearly before). In the example you give, each plot uses only 1 buffer, so in this case, your method does work, and it's what I use for this type of indicator. But it seems like as soon as your plot requires multiple buffers, for example if you change all plots for DRAW_COLOR_LINES which requires 2 buffers per plot, then this technique won't work anymore. Correct me if I'm wrong but I haven't found how to get it to work, unless I use a different method.
*edit* try this modified version of your code and you'll see what I mean:
In this version, what happens is when you switch to DRAW_NONE, the number of buffers required for the plot changes from 2 to 1 and although I don't know how to describe this with more intelligent words, it simply "screws" up the way your buffer sequence is matched to each plot. I have wondered if there was a way to work around this with a simple edit, but I haven't found it. Which leads me to think that I might need to use 12 buffers instead, the first 6 are the same and the next 12 are INDICATOR_CALCULATION buffers. These last 6 are the ones who hold all values no matter if the plot is to be shown or not. Then what I could do is if a plot is set to be drawn, I copy values from its two INDICATOR_CALCULATIONS buffers to its two INDICATOR_DATA buffers. If the plot is to be hidden, I fill its two INDICATOR_DATA buffers with empty values. So instead of using DRAW_NONE to hide the plot, I would rely on empty values to "hide" the buffer, while the plot values are still stored in DATA_CALCULATIONS buffers in case other plots need these values for their calculations, so that these other plots aren't affected if the first plot is hidden. I don't know if this is the best solution, but it's the only one I understand so far.
PS: Like I mentioned in my first post, I could easily change the color of the plots to clrNONE as a simpler way of removing them. And honestly, for some setups, that could be fine. I just found that for some applications I sometimes struggle with this method as the chart starts being filled with invisible plots that I end up creating annoying pop-ups on mouseovers that sometimes prevent me from getting the pop-up on mouseover for the plot I actually want info from.
Yes, the cause of buffers mapping confusion is from the line
PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_NONE);
which overrides the draw type of DRAW_ZIGZAG of the hidden plot, thus causing the 2 buffers saved for zigzag to turn into one buffer of DRAW_NONE.
so it needs to be replaced with CLR_NONE for the plot but with PLOT_SHOW_DATA = false in order to avoid the mouse popups and to not display the values in the data window.
#property indicator_chart_window #property indicator_buffers 6 #property indicator_plots 3 input bool inpShowBufLower=true; input bool inpShowBufMiddle=true; input bool inpShowBufUpper=true; //--- int hnd_bb; double middle_buffer1[], middle_buffer2[]; double upper_buffer1[], upper_buffer2[]; double lower_buffer1[], lower_buffer2[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { hnd_bb=iBands(_Symbol,_Period,50,0,2,PRICE_CLOSE); if(hnd_bb==INVALID_HANDLE) return INIT_FAILED; //--- PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(1,PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_ZIGZAG); //--- plot labeling PlotIndexSetString(0,PLOT_LABEL,"buf0;buf1"); PlotIndexSetString(1,PLOT_LABEL,"buf2;buf3"); PlotIndexSetString(2,PLOT_LABEL,"buf4;buf5"); //--- indicator buffers mapping SetIndexBuffer(0,middle_buffer1,INDICATOR_DATA); SetIndexBuffer(1,middle_buffer2,INDICATOR_DATA); SetIndexBuffer(2,upper_buffer1,INDICATOR_DATA); SetIndexBuffer(3,upper_buffer2,INDICATOR_DATA); SetIndexBuffer(4,lower_buffer1,INDICATOR_DATA); SetIndexBuffer(5,lower_buffer2,INDICATOR_DATA); if(inpShowBufMiddle) { PlotIndexSetInteger(0,PLOT_SHOW_DATA,true); PlotIndexSetInteger(0,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(0,PLOT_LINE_COLOR,clrYellow); } else { PlotIndexSetInteger(0,PLOT_SHOW_DATA,false); PlotIndexSetInteger(0,PLOT_LINE_COLOR,clrNONE); } if(inpShowBufUpper) { PlotIndexSetInteger(1,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(1,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(1,PLOT_LINE_COLOR,clrGreen); PlotIndexSetInteger(1,PLOT_SHOW_DATA,true); } else { PlotIndexSetInteger(1,PLOT_SHOW_DATA,false); PlotIndexSetInteger(1,PLOT_LINE_COLOR,clrNONE); } if(inpShowBufLower) { PlotIndexSetInteger(2,PLOT_SHOW_DATA,true); PlotIndexSetInteger(2,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(2,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(2,PLOT_LINE_COLOR,clrRed); } else { PlotIndexSetInteger(2,PLOT_SHOW_DATA,false); PlotIndexSetInteger(2,PLOT_LINE_COLOR,clrNONE); } ArraySetAsSeries(upper_buffer1,true); ArraySetAsSeries(lower_buffer1,true); ArraySetAsSeries(middle_buffer1,true); ArraySetAsSeries(upper_buffer2,true); ArraySetAsSeries(lower_buffer2,true); ArraySetAsSeries(middle_buffer2,true); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- double bb_low[1],bb_mid[1],bb_high[1]; //--- running indicator start formula int limit=rates_total-2-prev_calculated; for(int iBar = limit; iBar >= 0 && !IsStopped(); --iBar) { CopyBuffer(hnd_bb,BASE_LINE,iBar,1,bb_mid); middle_buffer1[iBar]=middle_buffer2[iBar+1]; middle_buffer2[iBar]=bb_mid[0]; CopyBuffer(hnd_bb,UPPER_BAND,iBar,1,bb_high); upper_buffer1[iBar]=upper_buffer2[iBar+1]; upper_buffer2[iBar]=bb_high[0]; CopyBuffer(hnd_bb,LOWER_BAND,iBar,1,bb_low); lower_buffer1[iBar]=lower_buffer2[iBar+1]; lower_buffer2[iBar]=bb_low[0]; } //--- return value of prev_calculated for next call return(rates_total-1); }
Yes, the cause of buffers mapping confusion is from the line
which overrides the draw type of DRAW_ZIGZAG of the hidden plot, thus causing the 2 buffers saved for zigzag to turn into one buffer of DRAW_NONE.
so it needs to be replaced with CLR_NONE for the plot but with PLOT_SHOW_DATA = false in order to avoid the mouse popups and to not display the values in the data window.
Yeah that's pretty much the problem. But I tried this method and it doesn't get rid of the mouse popups on the chart when the mouse is over the invisible plots unfortunately. If it does in your MT5, maybe there's an MT5 setting that's responsble for the pop-ups that I'm not aware of. Are you sure you're not getting the mouse over pop-ups when the plots are hidden?
Like I said before, it's often the case that I'll just settle for this. But I have many indicators that I show/hide (using labels that I click on to hide/show each of their plots) on the chart and sometimes it's a little annoying getting pop-ups when I'm trying to get a candles's open high low close or trying to double click on an indicator to open its settings and it opens the wrong one... it's not a major issue, but since I'm new to this I was thinking that maybe there's a simple way around this, one that would be even more efficient as it would free up some CPU and stop drawing the plot when I'm not showing it on the chart. Other than filling the buffers with empty values I'm not sure anything else can be done.
Yeah that's pretty much the problem. But I tried this method and it doesn't get rid of the mouse popups on the chart when the mouse is over the invisible plots unfortunately. If it does in your MT5, maybe there's an MT5 setting that's responsble for the pop-ups that I'm not aware of. Are you sure you're not getting the mouse over pop-ups when the plots are hidden?
Like I said before, it's often the case that I'll just settle for this. But I have many indicators that I hide with labels on the chart and sometimes it's a little annoying getting pop-ups when I'm trying to get a candles's open high low close... maybe instead of changing the code though I'll just get in the habbit of using the data window more often as that allows me to get the OHLC without even needing to be exactly on the candle. But sometimes I still think I should take the time to code a different solution, one that would have the added benefit of saving memory and CPU by not calculating the buffer values at all when I switch timeframes and the plot is hidden.
Sorry, I noticed after I published.
Fixed it with dynamic buffer allocation in that version with return to
PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_NONE);
Code
#property indicator_chart_window #property indicator_buffers 6 #property indicator_plots 3 input bool inpShowBufLower=true; input bool inpShowBufMiddle=true; input bool inpShowBufUpper=true; //--- int hnd_bb; double middle_buffer1[], middle_buffer2[]; double upper_buffer1[], upper_buffer2[]; double lower_buffer1[], lower_buffer2[]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { hnd_bb=iBands(_Symbol,_Period,50,0,2,PRICE_CLOSE); if(hnd_bb==INVALID_HANDLE) return INIT_FAILED; //--- PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(1,PLOT_DRAW_TYPE,DRAW_ZIGZAG); PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_ZIGZAG); //--- plot labeling PlotIndexSetString(0,PLOT_LABEL,"buf0;buf1"); PlotIndexSetString(1,PLOT_LABEL,"buf2;buf3"); PlotIndexSetString(2,PLOT_LABEL,"buf4;buf5"); AttachBuffers(); if(inpShowBufMiddle) { PlotIndexSetInteger(0,PLOT_SHOW_DATA,true); PlotIndexSetInteger(0,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(0,PLOT_LINE_COLOR,clrYellow); } else { PlotIndexSetInteger(0,PLOT_SHOW_DATA,false); PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_NONE); } if(inpShowBufUpper) { PlotIndexSetInteger(1,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(1,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(1,PLOT_LINE_COLOR,clrGreen); PlotIndexSetInteger(1,PLOT_SHOW_DATA,true); } else { PlotIndexSetInteger(1,PLOT_SHOW_DATA,false); PlotIndexSetInteger(1,PLOT_DRAW_TYPE,DRAW_NONE); } if(inpShowBufLower) { PlotIndexSetInteger(2,PLOT_SHOW_DATA,true); PlotIndexSetInteger(2,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(2,PLOT_LINE_WIDTH,1); PlotIndexSetInteger(2,PLOT_LINE_COLOR,clrRed); } else { PlotIndexSetInteger(2,PLOT_SHOW_DATA,false); PlotIndexSetInteger(2,PLOT_DRAW_TYPE,DRAW_NONE); } ArraySetAsSeries(upper_buffer1,true); ArraySetAsSeries(lower_buffer1,true); ArraySetAsSeries(middle_buffer1,true); ArraySetAsSeries(upper_buffer2,true); ArraySetAsSeries(lower_buffer2,true); ArraySetAsSeries(middle_buffer2,true); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- double bb_low[1],bb_mid[1],bb_high[1]; //--- running indicator start formula int limit=rates_total-2-prev_calculated; for(int iBar = limit; iBar >= 0 && !IsStopped(); --iBar) { CopyBuffer(hnd_bb,BASE_LINE,iBar,1,bb_mid); middle_buffer1[iBar]=middle_buffer2[iBar+1]; middle_buffer2[iBar]=bb_mid[0]; CopyBuffer(hnd_bb,UPPER_BAND,iBar,1,bb_high); upper_buffer1[iBar]=upper_buffer2[iBar+1]; upper_buffer2[iBar]=bb_high[0]; CopyBuffer(hnd_bb,LOWER_BAND,iBar,1,bb_low); lower_buffer1[iBar]=lower_buffer2[iBar+1]; lower_buffer2[iBar]=bb_low[0]; } //--- return value of prev_calculated for next call return(rates_total-1); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void AttachBuffers() { int buf_ind=0,buf_none=0; //--- indicator buffers mapping SetIndexBuffer(buf_ind++,middle_buffer1,INDICATOR_DATA); if(inpShowBufMiddle) SetIndexBuffer(buf_ind++,middle_buffer2,INDICATOR_DATA); else SetIndexBuffer(indicator_buffers-(++buf_none),middle_buffer2,INDICATOR_DATA); SetIndexBuffer(buf_ind++,upper_buffer1,INDICATOR_DATA); if(inpShowBufUpper) SetIndexBuffer(buf_ind++,upper_buffer2,INDICATOR_DATA); else SetIndexBuffer(indicator_buffers-(++buf_none),upper_buffer2,INDICATOR_DATA); SetIndexBuffer(buf_ind++,lower_buffer1,INDICATOR_DATA); if(inpShowBufLower) SetIndexBuffer(buf_ind++,lower_buffer2,INDICATOR_DATA); else SetIndexBuffer(indicator_buffers-(++buf_none),lower_buffer2,INDICATOR_DATA); }
*-
As per not calculating at all when the plot is hidden, that of course makes sense in addition.
You can just skip calculation in OnCalculate for the hidden parts.
In case you skip calculations, you can omit the else part from AttachBuffers(), which just takes care of alocating the buffers that are still calculated even though they are hidden.
Sorry, I noticed after I published.
Fixed it with dynamic buffer allocation in that version with return to
Code
*-
As per not calculating at all when the plot is hidden, that of course makes sense in addition.
You can just skip calculation in OnCalculate for the hidden parts.
Thanks a lot! I will look at that in detail later today when I have more time. I thought about re-arranging the buffer mapping when I first ran into this issue but it seemed too complicated and I wasn't sure I could do it without getting confused. But looking at the way you did it, it seems pretty simple and logical, and less confusing than I imagined. I think this is going to help for some of the things I'm doing. Thanks again! Will update my reply later when I have time to do more tests.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use