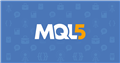
- www.mql5.com
- Machine learning
- Learn how to design a trading system by Accelerator Oscillator
- Custom Indicator is working fine on Strategy tester but not on price chart
This is an English language forum.
I have edited your title but in future please remember to only post in English.
Please edit your post and use the code button (Alt+S) when pasting code.
EDIT your original post, please do not just post the code correctly in a new post.
-
Please edit your (original) post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor -
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use