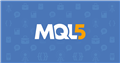
- www.mql5.com
- Learn how to design a trading system by Chaikin Oscillator
- MQL5 Wizard: Development of trading robots for MetaTrader 5
- Where to buy trading robots and indicators for MetaTrader 5
-
We can't verify the iCustom since you haven't provided the code/link.
-
Do you really expect an answer? There are no mind readers here and our crystal balls are cracked. Always post all relevant code (using Code button) or attach the source file.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problemWe can't see your broken code.
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010)
When you post code please use the CODE button (Alt-S)!
- Hannah Wanjiku Kimari - #: thank you i have reposted
You did not use the code button properly. if( reduce() || add() || creat() ) return true; return false;
See the difference? if( reduce() || add() || creat() ) return true; return false;
- Hannah Wanjiku Kimari - #: the indicator is free
What part of “we can't verify the iCustom since you haven't provided the indicator code/link.” was unclear?
the ea mises to take trades sometime exmple there will be sell arrow but ea mises to call for sell not all the time but sometimes so am not sure where the error is
You have provided no useful information for that.
Use the debugger or print out your variables, including _LastError and prices and find out why. Do you really expect us to debug your code for you?
Code debugging - Developing programs - MetaEditor Help
Error Handling and Logging in MQL5 - MQL5 Articles (2015)
Tracing, Debugging and Structural Analysis of Source Code - MQL5 Articles (2011)
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator - MQL5 Articles (2010)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use