May be 'Applay to First indicator's data' applays ma not to the close buffer.
Try to change this part:
for(int i=start_1;i<rates_total;i++) { data_1[i]=BoxCloseBuffer[i];
}
Try BoxOpenBuffer, BoxHighBuffer, BoxLowBuffer, BoxCloseBuffer.
So you will know to what buffer applays ma when you drag it into subwindow.
May be 'Applay to First indicator's data' applays ma not to the close buffer.
Try to change this part:
Try BoxOpenBuffer, BoxHighBuffer, BoxLowBuffer, BoxCloseBuffer.
So you will know to what buffer applays ma when you drag it into subwindow.
thanks to reply,
using " BoxOpenBuffer " get the same value, but must refresh or change chart, and after some time they have diff.
?!
and still have 2 problem .
thanks to reply,
using " BoxOpenBuffer " get the same value, but must refresh or change chart, and after some time they have diff.
?!
and still have 2 problem .
For ma you use proper cicle (recalculating only one new bar). But for renko on each tick recalculated several bars.
So we need to explore the renko cicle and recalculate ma in the same bars.
Solve start_1 и start_2 in another way and calling ma1.Solve and ma2.Solve set prev_calculated=0. But it is not good, it is impossible to limit ma solve range (ma will be solved for all bars every tick)
Yet it is necessary to clean the arrays for ma when the indicator is reset.
if(prev_calculated==0){ ArrayInitialize(MABuffer_1,0); ArrayInitialize(MABuffer_2,0); }-
For ma you use proper cicle (recalculating only one new bar). But for renko on each tick recalculated several bars.
So we need to explore the renko cicle and recalculate ma in the same bars.
Solve start_1 и start_2 in another way and calling ma1.Solve and ma2.Solve set prev_calculated=0. But it is not good, it is impossible to limit ma solve range (ma will be solved for all bars every tick)
Yet it is necessary to clean the arrays for ma when the indicator is reset.
THANK YOU,
i will try another method, ...
Hi
I would also like to add EMA in indicator buffer data to renko.mq5 ...
I'm also trying to use "BoxOpenBuffer" but just fucniona correctly the moment I compile. After some time, when the chart updates, I'm having problems ...
I believe the problem is that the MA is recalculated every bar for 1 minute, but the Renko is recalculated on several bars.
Already have a solution?
Thanks
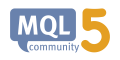
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi
i want to add EMA13 AND SMA50 on indicator buffer's data for renko.mq5 , https://www.mql5.com/en/code/1299
i use this : incmaonarray.mqh , test_maonarray.mq5 ( https://www.mql5.com/en/code/623 )
and i modify the renko as attached " renko_2.mq5 " , as below :
high lighted line add or changed.
and there is 2 problem i have:
1) there is some different in value between it above code " renko_2 " and when i use renko with my setting (3 pip) " renko_1 " and attach to subwindow 2 " Moving Average " Applay to First indicator's data as below :
&
if you use them will see the diff. in value.
-----
2) in both case after some time they have problem if dont refresh the chart or dont change time fram.
can you please help,