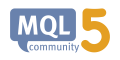
- www.mql5.com
//+------------------------------------------------------------------+ // //+------------------------------------------------------------------+ static datetime gsLastBarTime; //+------------------------------------------------------------------+ bool newBar( datetime _currentTime, int _period, bool _period_in_second = false, int _advance = 0, bool _advanced_in_second = false ) { int iAdvance = (_advance * 60); if ( _advanced_in_second ) iAdvance = _advance; datetime iCurrentTime = _currentTime += iAdvance; int iPeriod = (_period * 60); if ( _period_in_second ) iPeriod = _period; if ( (iCurrentTime - gsLastBarTime) >= iPeriod ) { gsLastBarTime = lastBarTime(_currentTime, _period, _period_in_second, _advance, _advanced_in_second); return(true); } return(false); }
//+------------------------------------------------------------------+ // //+------------------------------------------------------------------+ datetime lastBarTime( datetime _currentTime, int _period, bool _period_in_second = false, int _advance = 0, bool _advanced_in_second = false ) { int iAdvance = (_advance * 60); if ( _advanced_in_second ) iAdvance = _advance; datetime iCurrentTime = _currentTime += iAdvance; int iPeriod = (_period * 60); if ( _period_in_second ) iPeriod = _period; switch( _period ) { case PERIOD_W1: return( (iCurrentTime - (datetime) MathMod(iCurrentTime, (PERIOD_D1 * 60))) - (((TimeDayOfWeek(iCurrentTime) - 1) * PERIOD_D1) * 60) ); break; case PERIOD_MN1: return( (iCurrentTime - (datetime) MathMod(iCurrentTime, (PERIOD_D1 * 60))) - (((TimeDay(iCurrentTime) - 1) * PERIOD_D1) * 60) ); break; default: if ( _period > 0 ) return( iCurrentTime - (datetime) MathMod(iCurrentTime, iPeriod) ); else return( iCurrentTime ); break; } return(EMPTY); }
this is my complete function for manage the start of bar
the input of newBar function:
datetime _currentTime, ....TimeCurrent() or Time[0] for function in Tester mode
int _period,....any period in minutes or seconds
bool _period_in_second = false, .....not required, specifies if period are seconds or minutes, false = minutes, true = seconds
int _advance = 0,.....not required, any value that specifies if you want alert in advance
Example: PERIOD_H1 and advance 3 with _advanced_in_second = false....the alert is run 3 minutes before the natural start of bar
Example 1: PERIOD_M1 and advance 10 with _advanced_in_second = true....the alert is run 10 seconds before the natural start of bar
bool _advanced_in_second = false.....not required, specifies if _advance are seconds or minutes, false = minutes, true = seconds
return true if new bar is started otherwise false
the input of lastBarTime function:
datetime _currentTime, ....TimeCurrent() or Time[0] for function in Tester mode
int _period,....any period in minutes or seconds
bool _period_in_second = false, .....not required, specifies if period are seconds or minutes, false = minutes, true = seconds
int _advance = 0,.....not required, any value that specifies if you want alert in advance
bool _advanced_in_second = false.....not required, specifies if _advance are seconds or minutes, false = minutes, true = seconds
return the last time of started bar otherwise EMPTY
Eugenio
Thanks I'll look into this further!!
Thanks again.
Just tested it and it works a treat.
Thanks VERY much, you're a star!
This is one of the most flexible and concise Methods for detecting a New Bar. I especially like the way it implements the option of detection before the New bar forms
Excellent

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm trying to figure out how to create a isNewBar function that works across multiple timeframes.
What I've created is this but it doesn't seem to be working and I can't work out why!
BUT,
Thanks!