-
This is a handle to the object executing. It can never be NULL, as non-objects can not run code.
-
CFoo *foo = NULL; Comment(foo.Check());
If you try to do that, your program crashes because the pointer is invalid.
But, if I change the 'Check()' function like this:
the script will run successful, and I see the 'OK' message on chart.
Note that the 'foo' variable is NULL, yet.
Is there a reason for setting the foo pointer equal to NULL?
If you delete the pointer using the delete operator, you can run CheckPointer on the pointer variable and compare it to POINTER_INVALID.
delete foo;
Comment(CheckPointer(foo) == POINTER_INVALID); // true
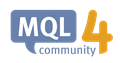
- docs.mql4.com
Yes, I have reason and this is only a sample example of my problem.
I want use the 'foo' variable for linked list of pointers and capsulate anything in a class.
The 'foo' initialed with NULL. If there is another initial value that I can check inside the 'Check()' function, let me know.
Since accessing an invalid pointer results in a crash, you can't run a check for an invalid pointer using the pointer in question.
In fact, I don't even know how you were able to call the Check() method because I copied and pasted your code and it always fails on Comment(foo.Check());
I don't know why the pointer is initialized to NULL, but any attempt to use that pointer, or call GetPointer with that pointer as a parameter, will crash.
You can, however, run CheckPointer on the foo pointer when it's initialized to NULL.
CFoo *foo = NULL; const bool isInvalidPtr = CheckPointer(foo) == POINTER_INVALID; Comment(isInvalidPtr); // trueIMO, it just sounds like you're going about it the wrong way, but only you can be certain of that.
My MT4 is:
LiteForex MT4 Terminal Version: 4.00 build 1353, 16 Dec 2021
and I run this script and include file successfully, and I see the 'OK' message on chart.
//+------------------------------------------------------------------+ //| TestFoo.mq4 | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict #include <Foo.mqh> void OnStart() { CFoo *foo = NULL; Comment(foo.Check()); }
//+------------------------------------------------------------------+ //| Foo.mqh | //| Copyright 2021, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property strict class CFoo { public: string Check() { return("OK"); } };
My MT4 is:
LiteForex MT4 Terminal Version: 4.00 build 1353, 16 Dec 2021
and I run this script and include file successfully, and I see the 'OK' message on chart.
That's weird. I left a short video showing what happens on my end. It's the same MT4 build as yours, just a different broker.
https://i.imgur.com/bfVvHgw.mp4
For what it's worth, I think it makes perfect sense for it to crash when attempting to execute foo.Check() since the pointer is invalid, so I'd say it's something with that particular version of MT4 that you're running.
I noticed that, there is a difference between run compiled script directly on chart vs run it in debug mode!! (you can see in below video)
Anyway, as Alexander said: "And, naturally, a check should be performed on the pointer before attempting to call any methods/members of that class pointer.", is the best solution.
Thanks everyone :)
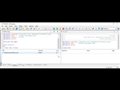
I noticed that, there is a difference between run compiled script directly on chart vs run it in debug mode!! (you can see in below video)
Anyway, as Alexander said: "And, naturally, a check should be performed on the pointer before attempting to call any methods/members of that class pointer.", is the best solution.
Thanks everyone :)
You are still using NULL so your code is wrong.
If your code is wrong you will get unpredictable results so just fix the code.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello mates,
I have an include file (named: Foo.mqh):
and a script file to test it:
In script file, there is a pointer variable to class 'CFoo' that named 'foo', and it initialized with NULL value.
Then I called the function 'Check()' of 'foo' variable. Method want to check if the 'foo' (caller variable) is NULL or not.
What is the correct if statement in 'Check()' function?
Any tips can be helpful.