Hello.
I found this script online and I have a question.
for(int j = 2; j <= 10; j = j + 2)
{
Print("The value of j is " + (string) j + ".");
}
It is supposed to print 2,4,6,8,10.
However I am confused because j+2 is included within the loop logic.
So the way it looks to me is ( j = 2 (and if) j <= 10 (then) add 2 to j (then) print.
But this means on the first step it would add 2 to j and it would skip 2 and print 4,6,8,10.
So my question is, why is j+j=2 skipped on the first loop run?
What is preventing j=2 from resetting j to 2 on every loop?
I don't see any explicit ordering or keyword that prevents J from equaling 2 at the beginning of every loop.
I am having a difficult time understanding how the computer sees this logic.
Because this ...
for( int j = 2; j <= 10; j = j + 2 ) { Print( "The value of j is " + (string) j + "." ); }
... is short hand for this ...
int j = 2; while( j <= 10 ) { Print( "The value of j is " + (string) j + "." ); j = j + 2; }
Because this ...
... is short hand for this ...
Oh so the structure of for loops are always the same?
for(variable = x; x (operator) y; x (operator) z?
What is preventing j=2 from resetting j to 2 on every loop?
I don't see any explicit ordering or keyword that prevents J from equaling 2 at the beginning of every loop.
I am having a difficult time understanding how the computer sees this logic.
Maybe this will help clear it up. This ...
for(expression1; expression2; expression3) operator;
... is equivalent to this ...
expression1; while(expression2) { operator; expression3; }
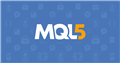
- www.mql5.com
j is not meant to be a constant, but a variable. It adds up and drives the loop forward.
Yeah but the part that is confusing me is that the beginning of the loop says j = 2.
So even if was j = 1000 before the loop began, it would be forced to become 2 as soon as it hits the j = 2 function.
So even was j = 1000 before the loop began, it would be forced to become 2 as soon as it hits the j = 2 function.
Nono the j=2 expression is only executed in the beginning. It won't go back down except if you put a j=j-2 expression in the body which is the part below the head that is ex ecuted on every run.
Okay so the first expression initializes, the second expression cancels the loop, and the third expression runs after body but before the next loop correct?

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello.
I found this script online and I have a question.
It is supposed to print 2,4,6,8,10.
However I am confused because j+2 is included within the loop logic.
So the way it looks to me is ( j = 2 (and if) j <= 10 (then) add 2 to j (then) print.
But this means on the first step it would add 2 to j and it would skip 2 and print 4,6,8,10.
So my question is, why is j+j=2 skipped on the first loop run?