hi guys,
I'm trying to write an EA. I have a problem with setting fixed sl and tp on my code. here's me code
I want to set TP at 300 pipets and SL at 100 pipet. I used NormalizeDouble function but it does'nt work.
any ideas?
SL and TP must be PRICE values, not pips/points.
You are passing the wrong information.
For BUY orders TP, you need the get the current ASK Price, add the desired 300 points to the ASK Price respecting its decimal digits and normalize it.
The resulting Price is the TP price.
Example: you want TP to be 300 points. Considering EURUD as an example. Considering an Ask Price of 1.87654
300 must be converted to the correct decimal digits, which will be: 0.00300
Then add: 0.00300 + 1.87654 = 1.87954
Since TP parameter must be PRICE, in this example, TP will be 1.87954
Here is a code to get you started, which does the decimal adjust, sum, and normalization.
int TP_Points = 300; double norm_tp; norm_tp = NormalizeDouble(Symbol.Ask() + (TP_Points * _Point), _Digits);
-
TP/SL are prices as @rrocchi says.
-
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit and open at the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close to a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes. My GBPJPY (OANDA) shows average spread = 26 points, but average maximum spread = 134 (your broker will be similar).
-
SL and TP must be PRICE values, not pips/points.
You are passing the wrong information.
For BUY orders TP, you need the get the current ASK Price, add the desired 300 points to the ASK Price respecting its decimal digits and normalize it.
The resulting Price is the TP price.
Example: you want TP to be 300 points. Considering EURUD as an example. Considering an Ask Price of 1.87654
300 must be converted to the correct decimal digits, which will be: 0.00300
Then add: 0.00300 + 1.87654 = 1.87954
Since TP parameter must be PRICE, in this example, TP will be 1.87954
Here is a code to get you started, which does the decimal adjust, sum, and normalization.
Thank you for your reply but i'm getting the same error from before: failed instant sell [Invalid request]
I'm new with mql5 so maybe you can help me a little more. here is my code:
//+------------------------------------------------------------------+ //| NewExp.mq5 | //| Yaser Amini | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Yaser Amini" #property link "https://www.mql5.com" #property version "1.00" int RSIH; double RSI[]; int STOCH; double Stoch[]; double DPer[]; // d% int MACDH; double MACD[]; double SIGNAL[]; double histogram_before; double histogram_now; bool firstBuy=1; int tp_points=300; double norm_tp; int sl_points=100; double norm_sl; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- RSIH=iRSI(_Symbol, _Period, 14, PRICE_CLOSE); STOCH=iStochastic(_Symbol, _Period, 21, 5, 7, MODE_SMA, STO_CLOSECLOSE); MACDH=iMACD(_Symbol, _Period, 12, 26, 9, PRICE_CLOSE); ArraySetAsSeries(RSI, 1); ArraySetAsSeries(Stoch, 1); ArraySetAsSeries(DPer, 1); ArraySetAsSeries(MACD, 1); ArraySetAsSeries(SIGNAL, 1); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { MqlTick Tick; MqlRates Rates[]; MqlTradeRequest TradeRequest; MqlTradeResult TradeResult; CopyRates(_Symbol, _Period, 0, 2, Rates); ArraySetAsSeries(Rates, 1); SymbolInfoTick(_Symbol, Tick); CopyBuffer(RSIH, 0, 0, 5, RSI); CopyBuffer(STOCH, 0, 0, 5, Stoch); CopyBuffer(STOCH, 1, 0, 5, DPer); CopyBuffer(MACDH, 0, 0, 5, MACD); CopyBuffer(MACDH, 1, 0, 5, SIGNAL); histogram_before=MACD[2]-SIGNAL[2]; histogram_now=MACD[0]-SIGNAL[0]; bool BuyPos=false; bool SellPos=false; if(PositionSelect(_Symbol)){ if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_BUY){ BuyPos=true; } else if(PositionGetInteger(POSITION_TYPE)==POSITION_TYPE_SELL){ SellPos=true; } } bool BuyStoch=Stoch[2]<DPer[2] && Stoch[0]>DPer[0]; bool BUYRSI=RSI[0]>55; bool BUYMACD=MACD[2]<SIGNAL[2] && MACD[0]>SIGNAL[0]; if(BuyStoch && BUYRSI && BUYMACD) { if(BuyPos){ return; } norm_tp=NormalizeDouble(Tick.ask + (tp_points* _Point), _Digits); //norm_sl=NormalizeDouble(Tick.ask - (sl_points* _Point), _Digits); TradeRequest.action=TRADE_ACTION_DEAL; TradeRequest.price=Tick.ask; TradeRequest.symbol=_Symbol; TradeRequest.volume=0.01; TradeRequest.type=ORDER_TYPE_BUY; TradeRequest.magic=65245; TradeRequest.type_filling=SYMBOL_FILLING_IOC; TradeRequest.tp=norm_tp; //TradeRequest.sl=norm_sl; OrderSend(TradeRequest, TradeResult); if(TradeResult.retcode==10009 || TradeResult.retcode==10008){ Print("Order Done!"); if(firstBuy){ firstBuy=0; } } else{ Print(TradeResult.retcode); } } if(!firstBuy){ bool SellStoch=Stoch[2]>DPer[2] && Stoch[0]<DPer[0]; bool SellRSI=RSI[0]<=48; bool SellMACD=MACD[2]>SIGNAL[2] && MACD[0]<SIGNAL[0]; if(SellStoch && SellRSI && SellMACD){ if(SellPos){ return; } norm_sl=NormalizeDouble(Tick.ask - (sl_points* _Point), _Digits); TradeRequest.action=TRADE_ACTION_DEAL; TradeRequest.price=Tick.bid; TradeRequest.symbol=_Symbol; TradeRequest.volume=0.01; TradeRequest.type=ORDER_TYPE_SELL; TradeRequest.magic=65246; TradeRequest.type_filling=SYMBOL_FILLING_IOC; //TradeRequest.tp=300; TradeRequest.sl=norm_sl; OrderSend(TradeRequest, TradeResult); if(TradeResult.retcode==10009 || TradeResult.retcode==10008){ Print("Order Done!"); } else{ Print(TradeResult.retcode); } } } } //+------------------------------------------------------------------+
As you can see i'm using ordersend() to my buy and sell executions. I'm not quite sure how to use sl and tp in this code. should I use sl and tp in both types of orders(buy and sell)?
Thank you for your reply but i'm getting the same error from before: failed instant sell [Invalid request]
I'm new with mql5 so maybe you can help me a little more. here is my code:
As you can see i'm using ordersend() to my buy and sell executions. I'm not quite sure how to use sl and tp in this code. should I use sl and tp in both types of orders(buy and sell)?
Hmm.. your are doing it raw, via the hardest way to program. :)
I Suggest you use Ctrade to do everything. It facilitates a lot, and it does the hard job of validating and making sure everything is done correctly.
Since you are new on MQL5, start by learn how to use CTrade functions, because this is the best road ahead for programming EAs.
And its a lot more easy to program.
Link below:
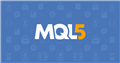
- www.mql5.com
Hmm.. your are doing it raw, via the hardest way to program. :)
I Suggest you use Ctrade to do everything. It facilitates a lot, and it does the hard job of validating and making sure everything is done correctly.
Since you are new on MQL5, start by learn how to use CTrade functions, because this is the best road ahead for programming EAs.
And its a lot more easy to program.
Link below:
Thank you so much, I will do.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi guys,
I'm trying to write an EA. I have a problem with setting fixed sl and tp on my code. here's me code
I want to set TP at 300 pipets and SL at 100 pipet. I used NormalizeDouble function but it does'nt work.
any ideas?