1. Increase the percentage of risk. You probably get a very small lot size (all answers are inside the library)
//+------------------------------------------------------------------+ //| Getting lot size for open long position. | //+------------------------------------------------------------------+ double CExpertMoney::CheckOpenLong(double price,double sl) { if(m_symbol==NULL) return(0.0); //--- double lot; if(price==0.0) lot=m_account.MaxLotCheck(m_symbol.Name(),ORDER_TYPE_BUY,m_symbol.Ask(),m_percent); else lot=m_account.MaxLotCheck(m_symbol.Name(),ORDER_TYPE_BUY,price,m_percent); if(lot<m_symbol.LotsMin()) return(0.0); //--- return(m_symbol.LotsMin()); } //+------------------------------------------------------------------+ //| Getting lot size for open short position. | //+------------------------------------------------------------------+ double CExpertMoney::CheckOpenShort(double price,double sl) { if(m_symbol==NULL) return(0.0); //--- double lot; if(price==0.0) lot=m_account.MaxLotCheck(m_symbol.Name(),ORDER_TYPE_SELL,m_symbol.Bid(),m_percent); else lot=m_account.MaxLotCheck(m_symbol.Name(),ORDER_TYPE_SELL,price,m_percent); if(lot<m_symbol.LotsMin()) return(0.0); //--- return(m_symbol.LotsMin()); }
2. I created such an advisor for tests a long time ago: Money Fixed Risk
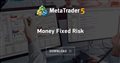
- www.mql5.com
Thanks Vladimir, I didn't think about looking at the code in the library.
Seeing that now I'm thinking that this class can't be used outside the Expert class structure. In my code I do not have any objects named m_account, or m_symbol.
Thanks Vladimir, I didn't think about looking at the code in the library.
Seeing that now I'm thinking that this class can't be used outside the Expert class structure . In my code I do not have any objects named m_account , or m_symbol .
Carefully read the answers that are written especially for you:
Forum on trading, automated trading systems and testing trading strategies
CMoneyFixedRisk keeps returning 0.0 as volume
Vladimir Karputov , 2021.06.28 05:50
***
2. I created such an advisor for tests a long time ago: Money Fixed Risk
I am trying to find a way to calculate the appropriate volume for longs and shorts dependent on a fixed percentage of risk. I stumbled across the CMoneyFixedRisk money management class and set it up in my EA. Every time it is called it seems to always return the value 0.0. Below is an example of how I am using it:
I know my coding is not the greatest, I'm still learning. Anyways, the outcome on every buy or sell condition within the strategy tester results in tradeVolume = 0.00. I check that the starting balance is at least $1000 so there should be enough in the account to provide some sort of volume greater than 0.00. Am I misunderstanding how this class is meant to be used?
Any guidance would be appreciated. Thanks.
You can use CAcountInfo instead (balance and Margin level) ... i'm calculating the risk with them. (and it works)...
https://www.mql5.com/es/docs/standardlibrary/tradeclasses/caccountinfo
And i'm using this too...
https://www.mql5.com/en/docs/trading/ordercalcmargin
And this Too :-)
m_symbol.LotsMin()
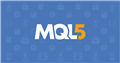
- www.mql5.com
Hello,
I tried to implement
#include <Expert\Money\MoneyFixedRisk.mqh>
in my expert advisor shown below.
//+------------------------------------------------------------------+ //| From Scratch.mq5 | //| Copyright 2022, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2022, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" #include <Expert\Money\MoneyFixedRisk.mqh> //--- inputs for money input double Money_FixRisk_Percent=2.5; // Risk percentage //| Global expert object | //+------------------------------------------------------------------+ CMoneyFixedRisk money; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- Set money parameters money.Percent(Money_FixRisk_Percent); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { double askPrice = SymbolInfoDouble(_Symbol, SYMBOL_ASK); double sl = askPrice - 0.0500; double lots = money.CheckOpenLong(askPrice, sl); Print(lots); } //+------------------------------------------------------------------+
Every time the variable "lots" return value 0.
I starteed Debug and noticed that in MoneyFixedRisk.mqh m_symbol is NULL.
When I call method CheckOpenLong() every time the following condition is TRUE.
double CMoneyFixedRisk::CheckOpenLong(double price,double sl) { if(m_symbol==NULL) return(0.0);
Do you have idea how to pass this and how to give value of m_symbol different of NULL?
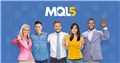
- 2022.10.17
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am trying to find a way to calculate the appropriate volume for longs and shorts dependent on a fixed percentage of risk. I stumbled across the CMoneyFixedRisk money management class and set it up in my EA. Every time it is called it seems to always return the value 0.0. Below is an example of how I am using it:
I know my coding is not the greatest, I'm still learning. Anyways, the outcome on every buy or sell condition within the strategy tester results in tradeVolume = 0.00. I check that the starting balance is at least $1000 so there should be enough in the account to provide some sort of volume greater than 0.00. Am I misunderstanding how this class is meant to be used?
Any guidance would be appreciated. Thanks.