I know that MathCeil() is used to Round up a double value to nearest integer value to the upside (eg: 0.01357 to 1.0)
but i am trying to round 0.01357 to 0.02 , whats my best option ?
I am trying to increase the lot size based on some conditions but cant find a way to round it up to increase the lot size.
any suggestions ?
Thanks in advance
The following code snippet may help a little bit:
#property strict double myCeil (double value, double step) { return (MathCeil (value/step)*step); } void OnStart() { double step, value; step = 0.01; value = 0.01357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.02357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.03357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f\n", step, value, myCeil(value,step)); step = 0.02; value = 0.01357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.02357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.03357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f\n", step, value, myCeil(value,step)); step = 0.05; value = 0.01357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.02357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f", step, value, myCeil(value,step)); value = 0.03357; PrintFormat ("step %0.2f value %0.5f ==> %0.2f\n", step, value, myCeil(value,step)); }
Output:
step 0.01 value 0.01357 ==> 0.02 step 0.01 value 0.02357 ==> 0.03 step 0.01 value 0.03357 ==> 0.04 step 0.02 value 0.01357 ==> 0.02 step 0.02 value 0.02357 ==> 0.04 step 0.02 value 0.03357 ==> 0.04 step 0.05 value 0.01357 ==> 0.05 step 0.05 value 0.02357 ==> 0.05 step 0.05 value 0.03357 ==> 0.05
Matthias
What is theprobleme with using NormalizeDouble function
The Math Utils - library may help you.
#include <math_utils.mqh> //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { double number = 0.01357; double result = RoundToDigitsUp(number, 2); Print(result); } //+------------------------------------------------------------------+ //output: 0.02
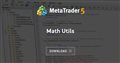
- www.mql5.com
The following code snippet may help a little bit:
Output:
Matthias
Dear Dr Matthias,
your solution is OK, but it fails some cases, due to the behavior of floating-point arithmetic.
Here is a test script to demonstrate the issues:
#include <math_utils.mqh> //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ double myCeil(double value, double step) { return (MathCeil(value/step)*step); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { Print( myCeil(9.13, 0.01) == 9.13 ); // output: false Print( RoundToStepUp(9.13, 0.01) == 9.13 ); // output: true Print( RoundToDigitsUp(9.13, 2) == 9.13 ); // output: true } //+------------------------------------------------------------------+

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I know that MathCeil() is used to Round up a double value to nearest integer value to the upside (eg: 0.01357 to 1.0)
but i am trying to round 0.01357 to 0.02 , whats my best option ?
I am trying to increase the lot size based on some conditions but cant find a way to round it up to increase the lot size.
any suggestions ?
Thanks in advance