1. Thanks very much for answer!
2. I tried asking my broker's hotline about what are the supported fillings, but they had no idea. Is there a technical way to find out?
3. My code for the order corresponds practically 100% to the example in the instructions (https://www.mql5.com/de/docs/integration/python_metatrader5/mt5ordersend_py):
# Vorbereitung der Struktur request für einen Kauf symbol = "USDJPY" symbol_info = mt5.symbol_info(symbol) if symbol_info is None: print(symbol, "not found, can not call order_check()") mt5.shutdown() quit() # Wenn das Symbol im MarketWatch nicht verfügbar ist, wird es hinzugefügt if not symbol_info.visible: print(symbol, "is not visible, trying to switch on") if not mt5.symbol_select(symbol,True): print("symbol_select({}}) failed, exit",symbol) mt5.shutdown() quit() lot = 0.1 point = mt5.symbol_info(symbol).point price = mt5.symbol_info_tick(symbol).ask deviation = 20 # Maximal akzeptierte Abweichung vom gewünschten Preis, angegeben in Punkten request = { "action": mt5.TRADE_ACTION_DEAL, "symbol": symbol, "volume": lot, "type": mt5.ORDER_TYPE_BUY, "price": price, "sl": price - 100 * point, #Ein Preis, zu dem eine Stop-Loss-Order aktiviert wird, wenn sich der Preis in eine ungünstige Richtung bewegt. "tp": price + 100 * point, #Ein Preis, zu dem ein Take-Profit-Auftrag aktiviert wird, wenn sich der Preis in eine günstige Richtung bewegt. "deviation": deviation, #Maximal akzeptierte Abweichung vom gewünschten Preis, angegeben in Punkten "magic": 234000, # EA-ID. Ermöglicht das Erkennen von Handelsaufträgen für eine anlytische Behandlung. Jeder EA kann beim Senden einer Handelsanfrage eine eindeutige ID festlegen. "comment": "python script open", "type_time": mt5.ORDER_TIME_GTC, "type_filling": mt5.ORDER_FILLING_RETURN, } # Senden eines Handelsauftrags result = mt5.order_send(request) # Prüfen des Ausführungsergebnisses print("1. order_send(): by {} {} lots at {} with deviation={} points".format(symbol,lot,price,deviation)); if result.retcode != mt5.TRADE_RETCODE_DONE: print("2. order_send failed, retcode={}".format(result.retcode)) # Abrufen des Ergebnisses als Liste und Darstellung Element für Element result_dict=result._asdict() for field in result_dict.keys(): print(" {}={}".format(field,result_dict[field])) # Wenn es die Struktur eines Handelsauftrags ist, werden die Elemente auch einzeln angezeigt if field=="request": traderequest_dict=result_dict[field]._asdict() for tradereq_filed in traderequest_dict: print(" traderequest: {}={}".format(tradereq_filed,traderequest_dict[tradereq_filed])) print("shutdown() and quit") mt5.shutdown() #quit() print("2. order_send done, ", result) print(" opened position with POSITION_TICKET={}".format(result.order))
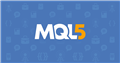
- www.mql5.com
1. Thanks very much for answer!
2. I tried asking my broker's hotline about what are the supported fillings, but they had no idea. Is there a technical way to find out?
3. My code for the order corresponds practically 100% to the example in the instructions (https://www.mql5.com/de/docs/integration/python_metatrader5/mt5ordersend_py):
What did the print out give you?
Did you try another broker, maybe? (demo account)
Print out the request structure to console before sending it to the function call:
# Senden eines Handelsauftrags # Bitte die request-struct auf der Konsole ausgeben, die Werte gegenprüfen. result = mt5.order_send(request)
What did the print out give you?
Did you try another broker, maybe? (demo account)
Print out the request structure to console before sending it to the function call:
So I got it working now :-)
The platform only accepts: "type_filling": mt5.ORDER_FILLING_IOC.
The mistake was also that you have to enter an explizit login for an order:
account=12345 authorized=mt5.login(account, password="XXXX") if authorized: # Anzeige der Daten des Handelskontos 'as is' print("") print("account_info:\n", mt5.account_info()) # Anzeige der Daten des Handelskontos als Liste print("") print("Show account_info()._asdict():") account_info_dict = mt5.account_info()._asdict() for prop in account_info_dict: print(" {}={}".format(prop, account_info_dict[prop])) else: print("failed to connect at account #{}, error code: {}".format(account, mt5.last_error()))
If you do it that way, you also get the individual specifics of the access back as an additional benefit, especially important for me that the ordering option is activated.
I faced the same problem, but to know the type_filling to, you need to use:
Go to Market Watch and select your symbol, then go to Specifications as shown below:
Then you will be able to see the filling from here. Hope this helps! I changed mine to FOK—works perfectly.
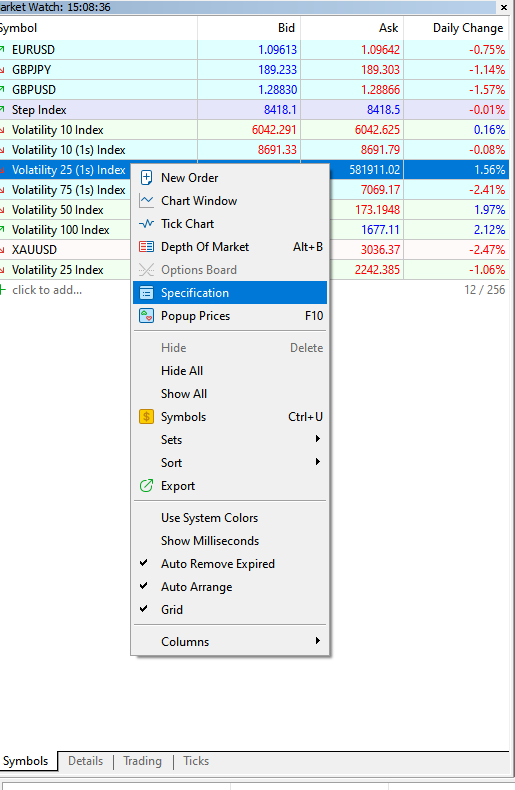
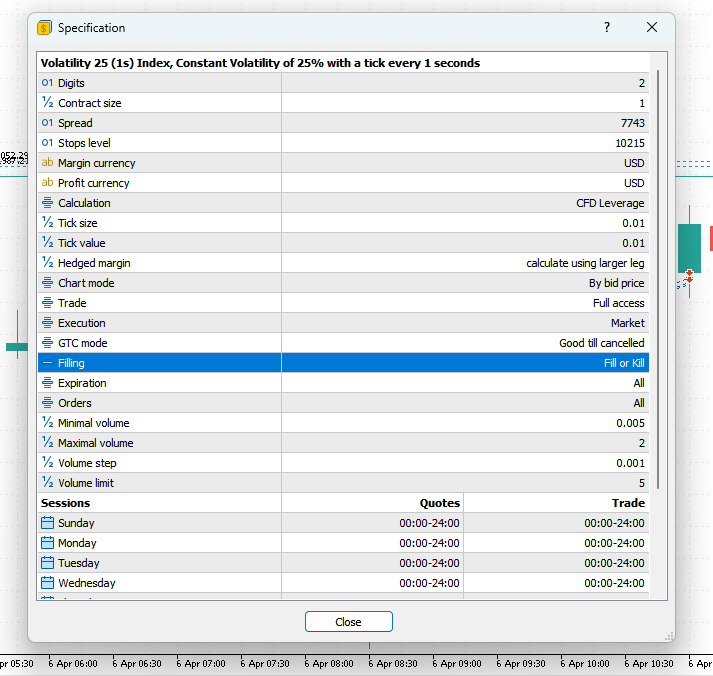
import MetaTrader5 as mt5 import time # Initialize MetaTrader 5 if not mt5.initialize(): print("initialize() failed, error code =", mt5.last_error()) quit() # Define trade parameters symbol = "Volatility 25 (1s) Index" # Ensure this is the exact symbol name in your MT5 lotsize = 0.005 deviation = 20 magic_number = 123456 # Optional: Your magic number comment = "Python Order" # Prepare the buy request structure symbol_info = mt5.symbol_info(symbol) if symbol_info is None: print(f"{symbol} not found, cannot place order") mt5.shutdown() quit() if not symbol_info.visible: print(f"{symbol} is not visible, trying to enable it") if not mt5.symbol_select(symbol, True): print(f"symbol_select({symbol}) failed, exit") mt5.shutdown() quit() point = symbol_info.point ask_price = mt5.symbol_info_tick(symbol).ask bid_price = mt5.symbol_info_tick(symbol).bid # --- Function to open a BUY order --- def open_buy_order(symbol, lotsize, price, deviation, magic, comment): request = { "action": mt5.TRADE_ACTION_DEAL, "symbol": symbol, "volume": lotsize, "type": mt5.ORDER_TYPE_BUY, "price": price, "sl": 0.0, # You can set stop-loss here if needed "tp": 0.0, # You can set take-profit here if needed "deviation": deviation, "magic": magic, "comment": comment, "type_time": mt5.ORDER_TIME_GTC, "type_filling": mt5.ORDER_FILLING_FOK, # Or other filling types } result = mt5.order_send(request) print(f"BUY order sent for {lotsize} lots of {symbol} at {price} with deviation {deviation} points") if result is None: print(f"order_send failed, retcode={mt5.last_error()}") elif result.retcode != mt5.TRADE_RETCODE_DONE: print(f"order_send failed, retcode={result.retcode}, comment='{result.comment}'") else: print(f"BUY order successful, deal ticket={result.deal}") return result # --- Function to open a SELL order --- def open_sell_order(symbol, lotsize, price, deviation, magic, comment): request = { "action": mt5.TRADE_ACTION_DEAL, "symbol": symbol, "volume": lotsize, "type": mt5.ORDER_TYPE_SELL, "price": price, "sl": 0.0, "tp": 0.0, "deviation": deviation, "magic": magic, "comment": comment, "type_time": mt5.ORDER_TIME_GTC, "type_filling": mt5.ORDER_FILLING_FOK, # Or other filling types } result = mt5.order_send(request) print(f"SELL order sent for {lotsize} lots of {symbol} at {price} with deviation {deviation} points") if result is None: print(f"order_send failed, retcode={mt5.last_error()}") elif result.retcode != mt5.TRADE_RETCODE_DONE: print(f"order_send failed, retcode={result.retcode}, comment='{result.comment}'") else: print(f"SELL order successful, deal ticket={result.deal}") return result # Open a BUY order buy_result = open_buy_order(symbol, lotsize, ask_price, deviation, magic_number, comment) # Wait for a moment (optional) time.sleep(1) # Open a SELL order sell_result = open_sell_order(symbol, lotsize, bid_price, deviation, magic_number, comment) # Shutdown MetaTrader 5 mt5.shutdown()

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
The full error message: