Code:
extern string _INVISIBLE_TRAIL = "__________INVISIBLE TRAIL_____________________"; extern bool Use_Money_Trail = true;//ENABLE extern double Money_Trail_Start=5;//TP input double TrailingStop = 2; // SL input double TrailingStep = 3;//STEP double TrallB = 0; double TrallS = 0; double price=0; double PROFIT_SUM1=0; double Trail_Stop ; double Trail_Step ; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- if( Use_Money_Trail = true) {INVISIBLE_TRAIL();}; } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ int INVISIBLE_TRAIL() { string pair = Symbol(); TrailingStart = Money_Trail_Start; /* * (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01) ; */ Trail_Stop = TrailingStop * (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); // length אורך כןלל של הטרייל; Trail_Step =TrailingStep* (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); // step גורל צעד. Print("TrailingStart",TrailingStart); double OOP=0; double SL=0; double TP=0; int b=0,s=0,tip, TicketB=0,TicketS=0; PROFIT_SUM1=0; for(int j=OrdersTotal(); j>0; j--) { if(OrderSelect(j,SELECT_BY_POS,MODE_TRADES)) { if(OrderSymbol()==Symbol()) { if(OrderType() == OP_BUY || OrderType() == OP_SELL) { PROFIT_SUM1 = PROFIT_SUM1 + OrderProfit(); } Print("PROFIT_SUM",PROFIT_SUM1); if(PROFIT_SUM1>= TrailingStart) { PRICE_ASK_BID=PROFIT_SUM1* (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); Print("PRICE_ASK_BID",PRICE_ASK_BID); tip = OrderType(); { if(tip==OP_BUY) { b++; } if(tip==OP_SELL) { s++; } } if((b>s)||(b==s)) { //----------------------------------------------BUY UP-------------------------------------------------------- // OOP = Ask-PRICE_ASK_BID; OOP = NormalizeDouble(OrderOpenPrice(),Digits); Print("Ask",Ask); Print("OOP",OOP); //----------------------------------------------TAKE PROFIT-------------------------------------------------------- TP=NormalizeDouble(Ask+Trail_Stop*pips,Digits); if(TP>=OOP+TrailingStart*pips && (TRAIL_TAKE_PROFIT==0 || TRAIL_TAKE_PROFIT+Trail_Step*pips<TP)) // if(TRAIL_TAKE_PROFIT <TP) TRAIL_TAKE_PROFIT=TP; Print("TP", TRAIL_TAKE_PROFIT); //----------------------------------------------STOP LOSS-------------------------------------------------------- SL=NormalizeDouble(Bid-Trail_Stop*pips,Digits); if(SL>=OOP-TrailingStart*pips && (TRAIL_STOP_LOSS==0 || TRAIL_STOP_LOSS-Trail_Step*pips<SL)) // if(TRAIL_STOP_LOSS <SL) TRAIL_STOP_LOSS=SL; Print("SL",TRAIL_STOP_LOSS); } if(b<s) { //----------------------------------------------SELL DOWN-------------------------------------------------------- // OOP = Ask+PRICE_ASK_BID; OOP = NormalizeDouble(OrderOpenPrice(),Digits); Print("Ask",Ask); Print("OOP",OOP); //----------------------------------------------TAKE PROFIT-------------------------------------------------------- TP=NormalizeDouble(Bid-Trail_Stop*pips,Digits); if(TP>=OOP-TrailingStart*pips && (TRAIL_TAKE_PROFIT==0 || TRAIL_TAKE_PROFIT-Trail_Step*pips<TP)) // if(TRAIL_TAKE_PROFIT <TP) TRAIL_TAKE_PROFIT=TP; Print("TP", TRAIL_TAKE_PROFIT); //----------------------------------------------STOP LOSS-------------------------------------------------------- SL=NormalizeDouble(Bid+Trail_Stop*pips,Digits); if(SL>=OOP-TrailingStart*pips && (TRAIL_STOP_LOSS==0 || TRAIL_STOP_LOSS-Trail_Step*pips<SL)) // if(TRAIL_STOP_LOSS <SL) TRAIL_STOP_LOSS=SL; Print("SL",TRAIL_STOP_LOSS); } } } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ if(OrdersTotal()>1) { if(b>s||b==s) { if(TRAIL_TAKE_PROFIT!=0) { Comment("Trail order TP"); DrawHline("TP", TRAIL_TAKE_PROFIT,clrBlue,1); if(Bid>= TRAIL_TAKE_PROFIT) { RemoveAllOrders();} } //--- if(TRAIL_STOP_LOSS!=0) { Comment("Trail order SL"); DrawHline("SL",TRAIL_STOP_LOSS,clrRed,1); if(Ask<=TRAIL_STOP_LOSS) {RemoveAllOrders();} } } //--- if(b<s) { if(TRAIL_TAKE_PROFIT!=0) { Comment("Trail order TP"); DrawHline("TP", TRAIL_TAKE_PROFIT,clrBlue,1); if(Bid<= TRAIL_TAKE_PROFIT) {RemoveAllOrders();} } //--- if(TRAIL_STOP_LOSS!=0) { Comment("Trail order SL"); DrawHline("SL",TRAIL_STOP_LOSS,clrRed,1); if(Ask>=TRAIL_STOP_LOSS) {RemoveAllOrders();} } } } else if(b==0&&s==0) { TRAIL_TAKE_PROFIT=0; TRAIL_STOP_LOSS=0; ObjectDelete("TP"); ObjectDelete("SL"); } return(0); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void DrawHline(string name,double P,color clr,int WIDTH) { if(ObjectFind(name)!=-1) ObjectDelete(name); ObjectCreate(name,OBJ_HLINE,0,0,P,0,0,0,0); ObjectSet(name,OBJPROP_COLOR,clr); ObjectSet(name,OBJPROP_STYLE,2); ObjectSet(name,OBJPROP_WIDTH,WIDTH); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ // CLOSE && Remove All Orders //+------------------------------------------------------------------+ void RemoveAllOrders() { for(int i = OrdersTotal() - 1; i >= 0 ; i--) { if(!OrderSelect(i,SELECT_BY_POS)) Print("ERROR"); if(OrderSymbol() != Symbol()) continue; price = MarketInfo(OrderSymbol(),MODE_ASK); if(OrderType() == OP_BUY) price = MarketInfo(OrderSymbol(),MODE_BID); if(OrderType() == OP_BUY || OrderType() == OP_SELL) { if(!OrderClose(OrderTicket(), OrderLots(),price,5)) Print("ERROR"); } else { if(!OrderDelete(OrderTicket())) Print("ERROR"); } Sleep(100); int error = GetLastError(); // if(error > 0) // Print("Unanticipated error: ", ErrorDescription(error)); RefreshRates(); } } //+------------------------------------------------------------------+
- Money is irrevalent to closing trades.
- What is blinking? There are no mind readers here and our crystal balls are cracked.
-
Help you with what? You haven't stated a problem. You haven't shown us your attempt (using the CODE button) and state the nature of your problem.
No free help 2017.04.21 - No code posted.
Aharon Tzadik:
Code:
Topics concerning MT4 and MQL4 have their own section.
In future please post in the correct section.
I have moved your topic to the MQL4 and Metatrader 4 section.
Keith Watford:
Topics concerning MT4 and MQL4 have their own section.
In future please post in the correct section.
I have moved your topic to the MQL4 and Metatrader 4 section.
Thank you, sorry for that.
Made some changes , the "buy" part is working fine, the stop loss on the sell part does not work, what is wrong ?
int INVISIBLE_TRAIL() { string pair = Symbol(); TrailingStart = Money_Trail_Start; Trail_Stop = TrailingStop * (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); // length אורך כןלל של הטרייל; Trail_Step =TrailingStep* (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); // step גורל צעד. Print("TrailingStart",TrailingStart); double OOPu=0; double OOPd=0; double SLUP=0; double TPUP=0; double SLDOWN=0; double TPDOWN=0; int b=0,s=0,tip, TicketB=0,TicketS=0; PROFIT_SUM1=0; static double PRICE_ASK_BID=0; for(int j=OrdersTotal(); j>0; j--) { if(OrderSelect(j,SELECT_BY_POS,MODE_TRADES)) { if(OrderSymbol()==Symbol()) { if(OrderType() == OP_BUY || OrderType() == OP_SELL) { PROFIT_SUM1 = PROFIT_SUM1 + OrderProfit(); } Print("PROFIT_SUM",PROFIT_SUM1); if(PROFIT_SUM1>= TrailingStart) { PRICE_ASK_BID=PROFIT_SUM1* (MarketInfo(pair,MODE_TICKVALUE) /MarketInfo(pair,MODE_TICKSIZE))*(StringFind("JPY", _Symbol)<0?0.0001:0.01); Print("PRICE_ASK_BID",PRICE_ASK_BID); tip = OrderType(); { if(tip==OP_BUY) { b++; } if(tip==OP_SELL) { s++; } } RefreshRates(); if((b>s)||(b==s)&&ObjectFind(0,"TPd")<0) { //----------------------------------------------BUY UP-------------------------------------------------------- OOPu = Ask-PRICE_ASK_BID; Print("Ask",Ask); Print("OOP",OOPu); //----------------------------------------------TAKE PROFIT-------------------------------------------------------- TPUP=NormalizeDouble(Ask+Trail_Stop*Point,Digits); if(TPUP>=OOPu+TrailingStart*Point && ((TRAIL_TAKE_PROFITu==0) || (TRAIL_TAKE_PROFITu+Trail_Step*Point)<TPUP)) if(TRAIL_TAKE_PROFITu<TPUP) {TRAIL_TAKE_PROFITu=TPUP;} Print("TP", TRAIL_TAKE_PROFITu); //----------------------------------------------STOP LOSS-------------------------------------------------------- SLUP=NormalizeDouble(Bid-Trail_Stop*Point,Digits); if(SLUP>=OOPu-TrailingStart*Point && ((TRAIL_STOP_LOSSu==0) || (TRAIL_STOP_LOSSu-Trail_Step*Point)<SLUP)) if(TRAIL_STOP_LOSSu<SLUP) {TRAIL_STOP_LOSSu=SLUP;} Print("SL",TRAIL_STOP_LOSSu); } if((b<s||b==s)&&ObjectFind(0,"TPu")<0) { //----------------------------------------------SELL DOWN-------------------------------------------------------- OOPd = Ask+PRICE_ASK_BID; Print("Ask",Ask); Print("OOP",OOPd); //----------------------------------------------TAKE PROFIT-------------------------------------------------------- TPDOWN=NormalizeDouble(Bid-Trail_Stop*Point,Digits); if(TPDOWN<=OOPd-TrailingStart*Point && ((TRAIL_TAKE_PROFITd==0) || (TRAIL_TAKE_PROFITd-Trail_Step*Point)>TPDOWN)) // if(TRAIL_TAKE_PROFIT <TP) // if(TRAIL_TAKE_PROFITd<TPDOWN) TRAIL_TAKE_PROFITd=TPDOWN; Print("TPD", TRAIL_TAKE_PROFITd); //----------------------------------------------STOP LOSS-------------------------------------------------------- SLDOWN=NormalizeDouble(Ask+Trail_Step*Point,Digits); if(SLDOWN<=OOPd+TrailingStart*Point && ((TRAIL_STOP_LOSSd==0) || (TRAIL_STOP_LOSSd+Trail_Step*Point)>SLDOWN)) // if(TRAIL_STOP_LOSS <SL) // if(TRAIL_STOP_LOSSd>SLDOWN) TRAIL_STOP_LOSSd=SLDOWN; Print("SLD",TRAIL_STOP_LOSSd); } } } } } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ if(OrdersTotal()>1) { RefreshRates(); if(b>s||b==s) { if(TRAIL_TAKE_PROFITu!=0) { Comment("Trail order TP UP"); DrawHline("TPu", TRAIL_TAKE_PROFITu,clrBlue,1); if(Bid>= TRAIL_TAKE_PROFITu) { RemoveAllOrders();} } //--- if(TRAIL_STOP_LOSSu!=0) { Comment("Trail order SL UP"); DrawHline("SLu",TRAIL_STOP_LOSSu,clrRed,1); if(Ask<=TRAIL_STOP_LOSSu) {RemoveAllOrders();} } } //--- if(b<s) { if(TRAIL_TAKE_PROFITd!=0) { Comment("Trail order TP DOWN"); DrawHline("TPd", TRAIL_TAKE_PROFITd,clrBlue,1); if(Bid<= TRAIL_TAKE_PROFITd) Print("EVENTBid",Bid); {RemoveAllOrders();} } //--- if(TRAIL_STOP_LOSSd!=0) { Comment("Trail order SL DOWN"); DrawHline("SLd",TRAIL_STOP_LOSSd,clrRed,1); RefreshRates(); if(Bid>=TRAIL_STOP_LOSSd) Print("EVENTAsk",Bid); {RemoveAllOrders();} } } } else if(b==0&&s==0) { TRAIL_TAKE_PROFITu=0; TRAIL_STOP_LOSSu=0; TRAIL_TAKE_PROFITd=0; TRAIL_STOP_LOSSd=0; ObjectDelete("TPu"); ObjectDelete("SLu"); ObjectDelete("TPd"); ObjectDelete("SLd"); } return(0); }
It does not print this line:
Print("EVENTAsk",Bid);
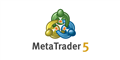
Basic Principles - Trading Operations - MetaTrader 5 Help
- www.metatrader5.com
Before you proceed to study the trade functions of the platform, you must have a clear understanding of the basic terms: order, deal and position...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello everyone,
I am 'm trying to build a trailing stop with money to close multiple trades
And encountered problems: it is blinking and not always closing trades .
I would appreciate any help.
This is the code: