After you have formed a trade order - you need to switch to the "tracking" mode. That is, you must strictly control the execution of a trade order. Until a trade order is executed, you cannot trade.
An example of a template that separates the execution of a trade order:
We catch the transaction
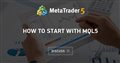
- 2020.09.17
- www.mql5.com
After you have formed a trade order - you need to switch to the "tracking" mode. That is, you must strictly control the execution of a trade order. Until a trade order is executed, you cannot trade.
An example of a template that separates the execution of a trade order:
We catch the transaction
Thanks a lot for you input Valdmir.
I found out a similar issue from long time ago in this forum, huge thread and it seems no definitive solution was found.
I'm going to study your approach, I'm afraid of these super complex coding to handle something that, IMO, the framework should do by ourselves somehow.
Thanks a lot for you input Valdmir.
I found out a similar issue from long time ago in this forum, huge thread and it seems no definitive solution was found.
I'm going to study your approach, I'm afraid of these super complex coding to handle something that, IMO, the framework should do by ourselves somehow.
easiest solution to avoid this type of problem is : Executing your code once per candle closure.
- Emado Btc #: I need help writing a code
Help you with what? You haven't stated a problem, you stated a want. Show us your attempt (using the CODE button) and state the nature of your difficulty.
No free help (2017)Or pay someone. Top of every page is the link Freelance.
Hiring to write script - General - MQL5 programming forum (2018)We're not going to code it for you (although it could happen if you are lucky or the issue is interesting).
No free help (2017) - Emado Btc #: How do I open a different batch of one lot after I open a deal?
Don't hijack other threads for your off-topic post. Next time, make your own, new, thread.
Hello there sirs,
I've run into a strange situation where my EA placed multiple orders even though I'm only alloting to perform CTrade PositionOpen if there is no open position.
This is a very simplistic approach from what I'm using:
Is there any particular case where PositionSelect would return false after a PositionOpen is sent?
I'm willing to believe that from the PositionOpen until there is a position, there are many thing between these steps that, on a very figh frequency market, another onTick would be processed before a the position is in place.
Check what happened to me live, the EA was trying to by only 1 volume/amount and ended buying 3. :(
I've thought about many ways to avoid this, but as awesome as MT5 is, I bet I'm missing something.
Thanks for any possible help.
Try this solution:
void OnTick() { MqlRates cnt[]; if(CopyRates(_Symbol,_Period,0,2,cnt)!=2) return; if(cnt[1].tick_volume>1) return; //--- your code here --- }
Have a nice coding :-)
if(cnt[1].tick_volume>1) return;
For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart), volume is unreliable (miss ticks), Price is unreliable (duplicate prices and The == operand. - MQL4 programming forum.) Always use time.
MT4: New candle - MQL4 programming forum #3 (2014)
MT5: Accessing variables - MQL4 programming forum #3 (2022)
I disagree with making a new bar function, because it can only be called once per tick (second call returns false). A variable can be tested multiple times.
Running EA once at the start of each bar - MQL4 programming forum (2011)
For a new bar test, Bars is unreliable (a refresh/reconnect can change number of bars on chart), volume is unreliable (miss ticks), Price is unreliable (duplicate prices and The == operand. - MQL4 programming forum.) Always use time.
MT4: New candle - MQL4 programming forum #3 (2014)
MT5: Accessing variables - MQL4 programming forum #3 (2022)
I disagree with making a new bar function, because it can only be called once per tick (second call returns false). A variable can be tested multiple times.
Running EA once at the start of each bar - MQL4 programming forum (2011)
Ok, thank you for the instructions you gave, I have seen the MT4 link you gave.
Does that mean your advice is to use time?
Because there is no Time[0] in MT5, then I will use TimeCurrent (), with the following code:
void OnTick() { static datetime Time0=0; if(TimeCurrent()>=Time0) {//start if //--- your code here --- Time0=TimeCurrent(); }//end if }
Is this OK?
Ok, thank you for the instructions you gave, I have seen the MT4 link you gave.
Does that mean your advice is to use time?
Because there is no Time[0] in MT5, then I will use TimeCurrent (), with the following code:
Is this OK?
No, TimeCurrent() updates on each new tick, not bar.
You need to use iTime() or something equivalent (CopyTime...).
iTime(NULL,0,0)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello there sirs,
I've run into a strange situation where my EA placed multiple orders even though I'm only alloting to perform CTrade PositionOpen if there is no open position.
This is a very simplistic approach from what I'm using:
Is there any particular case where PositionSelect would return false after a PositionOpen is sent?
I'm willing to believe that from the PositionOpen until there is a position, there are many thing between these steps that, on a very figh frequency market, another onTick would be processed before a the position is in place.
Check what happened to me live, the EA was trying to by only 1 volume/amount and ended buying 3. :(
I've thought about many ways to avoid this, but as awesome as MT5 is, I bet I'm missing something.
Thanks for any possible help.