Hi everyone,
I am having a problem with my EA. Specifically when I use the following function:
Most of the time I get the correct information. However, on occasion I get false information. Today is the 24th August 2020. When I called this function a few minutes ago, I first got the daily candle information for the 19th August 2020. When I called it again 3 seconds later, I got the correct candle information (for the 21st August 2020). Has anyone encountered this problem before?
Please read this MQL5 Reference https://www.mql5.com/en/docs/series/copyhigh
When requesting data from an Expert Advisor or script, downloading from the server will be initiated, if the terminal does not have these data locally, or building of a required timeseries will start, if data can be built from the local history but they are not ready yet. The function will return the amount of data that will be ready by the moment of timeout expiration, but history downloading will continue, and at the next similar request the function will return more data.
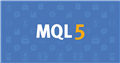
- www.mql5.com
You have to analyze the return value for all of these calls.
Return Value
The High price of the bar (indicated by the 'shift' parameter) on the corresponding chart or 0 in case of an error
So if some return 0 then of course you are going to get a different output.
//+------------------------------------------------------------------+ //| DailyPivot calculation function | //+------------------------------------------------------------------+ double DailyPivot(string CurrencyPair) { double dHigh, dLow, dClose; dHigh = iHigh(CurrencyPair,PERIOD_D1,1); if(dHigh == 0) { return(-1); } dLow = iLow(CurrencyPair,PERIOD_D1,1); if(dLow == 0) { return(-1); } dClose = iClose(CurrencyPair,PERIOD_D1,1); if(dClose == 0) { return(-1); } Print("dHigh: ",dHigh); Print("dLow: ",dLow); Print("dClose: ",dClose); return (dHigh + dLow + dClose)/3; }
Then when you call the function you check it's outcome also.
double pivot = DailyPivot("EURUSD"); if(pivot == -1) { Print("Pivot error !"); } if(pivot != -1) { Print("Pivot "+(string)pivot); }
Always check because it's possible that the data you are requesting is not available and this can (and will) result in undesirable results if left unchecked.
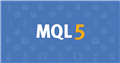
- www.mql5.com
Please read this MQL5 Reference https://www.mql5.com/en/docs/series/copyhigh
When requesting data from an Expert Advisor or script, downloading from the server will be initiated, if the terminal does not have these data locally, or building of a required timeseries will start, if data can be built from the local history but they are not ready yet. The function will return the amount of data that will be ready by the moment of timeout expiration, but history downloading will continue, and at the next similar request the function will return more data.
Thanks for your reply Roberto. That makes sense except that the data I had was already downloaded locally in my MT4 history centre. Is this what CopyHigh does? Or do I need to call CopyHigh regardless?
You have to analyze the return value for all of these calls.
So if some return 0 then of course you are going to get a different output.
Then when you call the function you check it's outcome also.
Always check because it's possible that the data you are requesting is not available and this can (and will) result in undesirable results if left unchecked.
On MT4: Unless the current chart is that specific symbol(s)/TF(s) referenced, you must handle 4066/4073 errors before accessing candle/indicator values.
Download history in MQL4 EA - Forex Calendar - MQL4 programming forum - Page 3 #26 № 4 2019.05.20
On MT5: Unless the chart is that specific pair/TF, you must synchronize the terminal Data from the Server before accessing candle/indicator values.
Is it mystical?! It is! - Withdraw - Technical Indicators - MQL5 programming forum 2019.05.31
Timeseries and Indicators Access / Data Access - Reference on algorithmic/automated trading language for MetaTrader 5
Synchronize Server Data with Terminal Data - Symbols - General - MQL5 programming forum
SymbolInfoInteger doesn't work - Symbols - General - MQL5 programming forum 2019.09.03
OnCalculate during weekend MT5 - General - MQL5 programming forum
Hi everyone,
I am having a problem with my EA. Specifically when I use the following function:
Most of the time I get the correct information. However, on occasion I get false information. Today is the 24th August 2020. When I called this function a few minutes ago, I first got the daily candle information for the 19th August 2020. When I called it again 3 seconds later, I got the correct candle information (for the 21st August 2020). Has anyone encountered this problem before?
because in getting these values sometimes its possible to have overflow.
Hi Marco, thanks for your reply. Yeah that is a simple filter I could use for zero values, however, in some instances (like the example I mentioned) when it does return values, but they are just for the wrong day!
because if you want current day's, you need to input 0 in change of 1.
Hi everyone
I am new here and I got confused, can any one enlight me please, why the questions in this forum look more like coding instead of trading and is it normal that I don't understand anything?
hmm, well you are returning yesterdays iHight here, is that right ?
because if you want current day's, you need to input 0 in change of 1.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone,
I am having a problem with my EA. Specifically when I use the following function:
Most of the time I get the correct information. However, on occasion I get false information. Today is the 24th August 2020. When I called this function a few minutes ago, I first got the daily candle information for the 19th August 2020. When I called it again 3 seconds later, I got the correct candle information (for the 21st August 2020). Has anyone encountered this problem before?