Try this instead.
ObjectCreate("Line" + candleCount,OBJ_VLINE,0,Time[0],0);
Try this instead.
Thanks mt4ski! That made the difference. On that line, which input changes the colour? I'm trying to create a vertical line that is one colour for oversold and another for overbought. Your help is very appreciated!
PS
I tried looking in the docs but there are a couple pages of code examples that don't seem to look anything like the simple line you wrote.
Thanks mt4ski! That made the difference. On that line, which input changes the colour? I'm trying to create a vertical line that is one colour for oversold and another for overbought. Your help is very appreciated!
PS
I tried looking in the docs but there are a couple pages of code examples that don't seem to look anything like the simple line you wrote.
Don't just copy and paste code, try to understand what you're doing. You don't need to look further than the documentation.
bool ObjectCreate( string object_name, // object name ENUM_OBJECT object_type, // object type int sub_window, // window index datetime time1, // time of the first anchor point double price1, // price of the first anchor point datetime time2=0, // time of the second anchor point double price2=0, // price of the second anchor point datetime time3=0, // time of the third anchor point double price3=0 // price of the third anchor point );
A vertical line needs a vertical axis value: time, and a horizontal line needs a price value. When a default parameter value is set, the parameter is not required if you don't need it.
You set the color with ObjectSetInteger
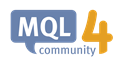
- docs.mql4.com
bool ObjectSet( string object_name, // object name int index, // property index double value // value );too.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm brand new to coding. I found a course on Udemy that is really helpful but when I want to do something that the course doesn't cover, I really struggle to understand the MQL4 Docs. For example, it doesn't cover adding objects to the chart. Right now I'd like to add a simple vertical line whenever the stochastics indicator crosses above the 80 or below 20.
As I look online I see people drawing vertical lines in all these different conditions but I'm having a hard time simplifying it to do what I want.
Could someone show me an example of the most basic form of adding an object? There are so many options that I think I'm a little overwhelmed. In the code below I am trying to draw a vertical line when stochastics crosses over 80. I created a variable for the upper and lower line so I can adjust them later as intern variables. I created that candleCount variable because I read somewhere that multiple lines can't be drawn unless their name is different. I think I saw someone write the name in this way before.
This is all making my head spin. I feel like I will be so well off if I can just see a basic example of how to add a vertical line, what the necessary inputs are and then I can tweak them and build on that as I learn.
Any help is appreciated.