You should ask on the discussion page of that EA. https://www.mql5.com/en/code/1225
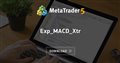
Exp_MACD_Xtr
- www.mql5.com
Trading system using the MACD_Xtr. A signal to perform a deal is formed at bar closing when a red or a green color bar appears. Exit from the deals is performed either by a pending order, or by bar color changing. Place compiled file to the...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
It gives me this error when I try to do a backtest on IcMarkets
BuyPositionOpen(): Incorrect data for a trade request structure!
BuyPositionOpen(): OrderCheck(): Specified type of order execution for the balance is not supported