If it's only two languages you could use the ternary operator:
string Language = TerminalInfoString(TERMINAL_LANGUAGE); #define FunctionLine ((Language=="Portuguese" ? "Função: "+__FUNCTION__+" linha: " : "Function: "+__FUNCTION__+" line: ")+(string)__LINE__) int start() { if (true) {Print(GetLastError()+" "+FunctionLine);} return(0); }
A macro will not work since line and function are also macros, not variables. If you define it on line 5 and use it on line 10 in a function, your output will be global 5 — not what you want.
Make a proper function and pass function/line/message to it.
1. Works like a charm over here:
#define FunctionLine ((Language=="Portuguese" ? "Função: "+__FUNCTION__+" linha: " : "Function: "+__FUNCTION__+" line: ")+(string)__LINE__) string Language; int OnInit() { Language="English"; Print("Language=",Language," ",FunctionLine); // --> Language=English Function: OnInit line: 634 Language="Portuguese"; Print("Language=",Language," ",FunctionLine); // --> Language=Portuguese Função: OnInit linha: 637
2. Agree, it's better wrapped into a function call.
#define LogError(s) _LogError(s,__FUNCTION__,__LINE__) void _LogError(string text,string funcname,int line) { string location="Function: " + funcname + " line: "; if(Language=="Portuguese") location="Função: " + funcname + " linha: "; else if(Language=="...") location="...: " + funcname + " ...: "; Print(text,(_LastError ? " error "+(string)_LastError : "")," ",location,line); } int OnInit() { if(InpPeriod<2) { LogError("invalid period: "+(string)InpPeriod); // --> invalid period: 1 Function: OnInit line: 15 return INIT_PARAMETERS_INCORRECT; } ... }
If it's only two languages you could use the ternary operator:
lippmaje YOU ARE THE BEST!
Thank you very much!
Here is the final solution for this case:
string Language = TerminalInfoString(TERMINAL_LANGUAGE); #define FunctionLine (((Language=="Portuguese (Brazil)" || Language=="Portuguese (Portugal)") ? "Função: "+__FUNCTION__+" linha: " : "Function: "+__FUNCTION__+" line: ")+(string)__LINE__) int start() { if (true) Print(GetlastError()+FunctionLine); return(0); }
My goal was to use as few words as possible in creating this solution, and this macro fell perfectly to save such words in code.
Below is a workaround that I had created while I couldn't find a better one, because I didn't want the macro to have the Print command because I wanted to call this macro into several different prints in the code.
string Language = TerminalInfoString (TERMINAL_LANGUAGE); #define FunctionLine if (Language == English) {Print ("Function:" + __ FUNCTION __ + "line:" + __ LINE__);} \ if (Language == Portuguese) {Print ("Função:" + __ FUNCTION __ + "linha:" + __ LINE__);} int start () { if (true) {FunctionLine;} return (0); }
My first attempt was just creating a function (as below) and I found that the macros __FUNCTION__ and __LINE__ were always printing the same line and function, ie function and line where I call them (FunctionLine and line X of it), then not It made sense, because I need them inside the code in different places, the solution I found was to define a new macro up there to save repetitive lines of code, but I was missing this detail that I had not discovered to make it work the way I need it.
string FunctionLine () { string Language = TerminalInfoString (TERMINAL_LANGUAGE); // --- language check if (Language == "English") {return ("Function:" + __ FUNCTION __ + "Line:" + __ LINE__);} // English // --- Portuguese - in all other cases return ("Função:" + __ FUNCTION __ + "Linha:" + __ LINE__); // Portuguese }
Of course, for more than two languages it will be necessary to create a function or the print alternative within the macro as I had originally created.
Thank you very much lippmaje and William Roeder for the considerations and shared knowledge, were very important!
Success and good trades! :D
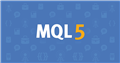
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello people! :)
All right?
I am looking for a way to define a FunctionLine conditional macro, that when the defined language is English, the macro returns ( " Function: " + __FUNCTION__ + " line: " + __LINE__"), when the defined language is Portuguese, the macro returns ( " Função: " + __FUNCTION__ + " linha: " + __LINE__"). I've researched a lot and haven't found a solution yet. How can I do this?
Thanks in advance for your attention! : D
Example with error: